Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial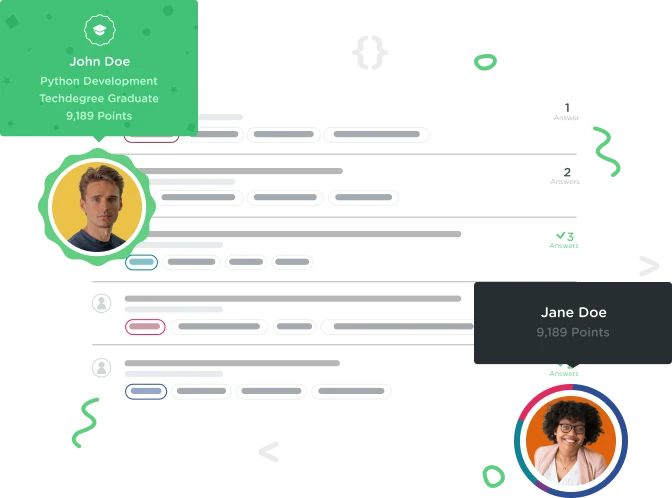

Christopher Borchardt
2,908 PointsWrong random number
ok, I made my code a bit more complicated than it strictly needed to be for the challenge, but I had already made almost exactly this program to challenge myself earlier in the course. I made the program to prompt the user for a lower and upper value. Then have the program adjust the number of guesses allowed to get the number based on how big a gap there is between the values.
I have 2 problems, one that i had before this challenge and one that only came up when i added in the user picking the lower value instead of it just being 1.
When i set the program to prompt for a lower value too, the math seems to be off. For testing i was putting in 50 and 100 for the lower and upper values, but i was regularly getting random numbers smaller than 50.
The problem that i had from the beginning was if the number of possible guesses was over 1k, the program just stops. My code is below.
/* Random number guessing game. The user can select a number and the game Will generate a random number from 1 to the number selected. The user has a different number of chances to guess the number depending on how large a number selected */
// Prompt user to pick how high the random number will go and generate the random number alert('Pick 2 numbers, and I will pick a random number between them for you to guess'); var lower = prompt("Pick a number"); var upper = prompt("Pick a larger number"); var range = upper - lower; var randomNumber = Math.floor(Math.random()* (upper- lower + 1 )) + 1; var guesses = 0; var guessed = false;
// dermine the number of guesses allowed by how large a number the user picks.
function numberOfGuesses (range) {
if (range <= 10) {
guesses = 3 ;
} else if (range <= 100) {
guesses = 8;
} else if (range <= 1000) {
guesses = 15;
}else {
guesses = math.ceil(math.log2(range));
}
return guesses;
}
// compare the guess to the random number generated. function main (guesses) { for (i = guesses; i > 0; i--) { var guess = prompt('I am thinking of a number between ' + lower + ' and ' + upper + '. What is it? You can guess ' + guesses + 'time(s)'); if (parseInt(guess) === randomNumber){ guessed = true; break; } else if (guess < randomNumber) { alert('Your guess was too low, try again!'); guesses -= 1; } else { alert('Your guess was too high, try again!'); guesses -= 1; } } if (guessed === true) { alert('You guessed it! My Number was' + randomNumber + '!'); } else { alert('Betetr luck next time! My Number was ' + randomNumber + '!'); } }
numberOfGuesses(range); main(guesses);

Christopher Borchardt
2,908 Pointsok, i fixed one of my problems, i forgot to convert the strings from the prompts to ints.
this is my current code. the only problem I have right now is if the range is over 1000. it doesn't matter what type of math i try to use to make the number of guesses scale , it still fails.
1 Answer
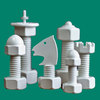
Steven Parker
231,269 PointsI don't know why the amount would make a difference, but I'm guessing your calculations sometimes become string concatenations. Some things I noticed are:
- Remember that prompt returns a string. To be safe, convert it to a number right away.
- To make your range fully adjustable, multiply by one more than your range, and then add the lower value.
- JavaScript is case-sensitive, so "Math" must have a capital "M".
(I learned something myself .. I thought you were going to need ln(range)/ln(2) .. but look, there's a log2!)
Some suggestions:
- make sure there are spaces where literal strings concatenate with variables
- fold alert text into prompts so the computer waits on the person and not vice-versa
- add validity checking (and re-tries) on inputs
- don't say "try again" right before "game over"
And a code snippet:
var lower = parseInt(prompt("Pick 2 numbers, and I will pick a random number between them for you to guess"
+ "\n\nFirst, pick the Smaller number"));
var upper = parseInt(prompt("Now, pick the Larger number"));
var range = upper - lower;
var randomNumber = Math.floor(Math.random() * (range + 1)) + lower;
var guesses = numberOfGuesses(range);
var guessed = false;
// dermine the number of guesses allowed by how large a number the user picks.
function numberOfGuesses(range) {
return Math.ceil(Math.log2(range + 1));
}
Happy coding!

Christopher Borchardt
2,908 PointsOk, i've got most of it working.
I added a validity check, but i'm having issues with it.
I have a while loop so it will repeat until both inputs are valid.
It works great if the information is not valid, it gives the expected error and prompts again.
The problem is when the information IS valid it doesn't exit the loop like i expect it to, it just keeps prompting for values .
Christopher Borchardt
2,908 PointsChristopher Borchardt
2,908 Pointshere is a link to a snapshot of my code if that would help
http://w.trhou.se/dtfv8xhvs0