Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial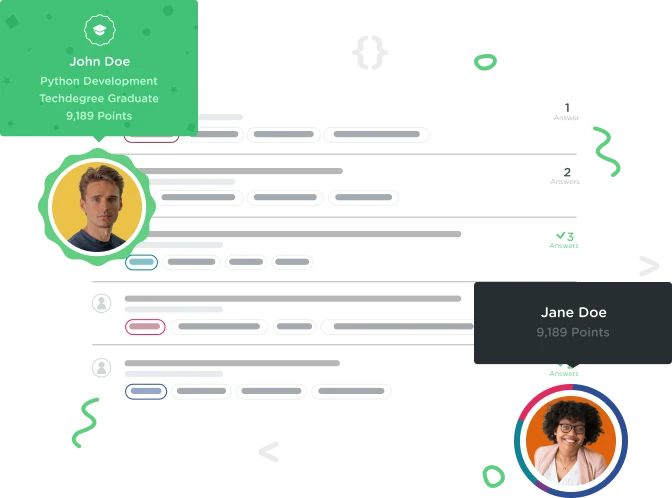
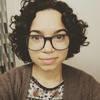
kelley riley
3,159 PointsWrote some simple code to study structs as I'm struggling with them. Will you check out my little method?
//Goal is to make a method to calculate the distance from one's location to the destination, using simple Coordinate custom Type
struct Coordinate {
let long: Int
let lat: Int
func computeDistance(x: Int, y: Int) -> Coordinate {
let distanceOfX = x - long
let distanceOfY = y - lat
let results = Coordinate(long: distanceOfX, lat: distanceOfY)
return results
}
}
let myLocation = Coordinate(long: 4, lat: 5)
let distanceToStore = myLocation.computeDistance(x: 6, y: 2)
1 Answer
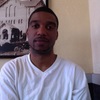
Marlon Henry
6,885 PointsYou could do all this in a init method
struct Coordinate {
var distanceOfX: Int
var distanceOfY: Int
init(long:Int, lat:Int, x: Int, y: Int){
self.distanceOfX = x - long
self.distanceOfY = y - lat
}
}
//Assign the struct here:
let distanceToStore = Coordinate(long: 4, lat: 5, x: 6, y: 2)
//And then all you got to do is call:
distanceToStore.distanceOfX
distanceToStore.distanceOfY