Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial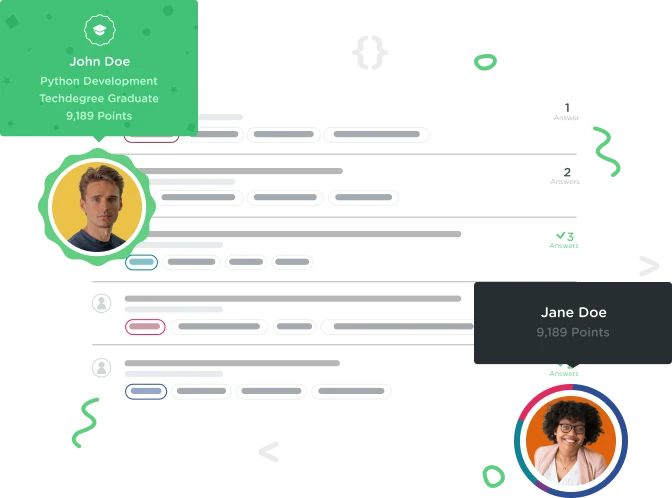
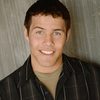
Kyler Smith
10,110 PointsXCODE application giving fatal error.
I am working on my own App for some practice and am running into a semantics problem. The following code is only the ViewController.swift file. This is a single view application. It loads onto the screen fine and then when I try to move the slider, it crashes and gives me a fatal error: unexpectedly unwrapped nil in an optional value. Will someone please help me find where the optional value is that is causing the problem? I can post other files if requested.
//
// ViewController.swift
// RBGSlider
//
// Created by Kyler on 7/3/16.
// Copyright © 2016 Kyler. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var screenView: UIView!
var redSliderValue: Float = 50.0
var blueSliderValue: Float = 50.0
var greenSliderValue: Float = 50.0
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// Get the value of the sliders
@IBAction func redSlider(sender: UISlider) {
redSliderValue = sender.value
updateScreenColor()
}
@IBAction func blueSlider(sender: UISlider) {
blueSliderValue = sender.value
updateScreenColor()
}
@IBAction func greenSlider(sender: UISlider) {
greenSliderValue = sender.value
updateScreenColor()
}
// Helper functions
func updateScreenColor() {
screenView.backgroundColor = UIColor(colorLiteralRed: redSliderValue, green: greenSliderValue, blue: blueSliderValue, alpha: 1)
}
}
2 Answers
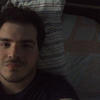
henriquegiuliani
12,296 PointsYou've made an IBOutlet to an UIView and not to the UISlider itself. Try adding an IBOutlet for it and it should work fine.
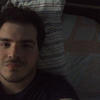
henriquegiuliani
12,296 PointsThe IBAction performs some code but needs a sender that is a UISlider as a parameter. The error is occurring because you are not specifying which slider performs that action. Got it? Did it work?

M Willens
125 PointsHenrique, please help :( I ran into an issue when making a simple interactive story app (choose your own adventure type). Here's my issue:
Kyler Smith
10,110 PointsKyler Smith
10,110 PointsI do not understand why I need an IBOutlet for the sliders because I have the IBAction for them. Can you explain this for me?