Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial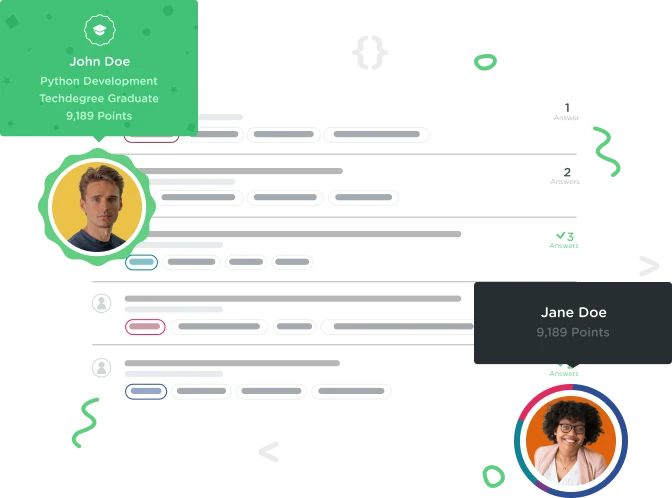

boris said
3,607 PointsX-Code giving error that I can not assign type 'Point' to 'Int' in enemy class. Happened after tower was created
Can somebody please tell me why this is happening. Thank you in advanced Here is my code:
let x1 = 0
let y1 = 0
let coordinate1: (x: Int, y: Int) = (0, 0)
struct Point {
let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
func surroundingPoints(withRange range: Int = 1) -> [Point]{
var results: [Point] = []
for xCoord in (x-range)...(x+range) {
for yCoord in (y-range)...(y+range) {
let coordinatePoint = Point(x: xCoord, y: yCoord)
results.append(coordinatePoint)
}
}
return results
}
}
let coordinatePoint = Point(x: 2, y: 2)
coordinatePoint.surroundingPoints()
class Enemy {
var life = 2
let position: Int = 1
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseHealth(factor: Int) {
life = life - factor
}
}
class Tower {
let position: Point
var range = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
}
1 Answer
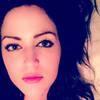
Nikki Bearman
3,389 PointsHi Boris,
There are a couple of minor errors/mix ups I've found in the code you've provided, which I think are just copywriting errors so just worth checking over quite closely in future. It's easy to do though, I've done the same many times!
Within class Enemy, you have entered:
var life = 2
let position: Int = 1
var strength: Int = 1
This should actually be:
var life = 2
let position: Point
var strength: Int = 1
You have entered the constant position as type Int = 1 when it should have been type Point. This explains why you were receiving the error "could not assign type 'Point' to 'Int'". You entered self.position = Point(x: x, y: y) and so it was trying to assign Point which opposes the type Int you set earlier.
Just as a heads up I also noticed that within your class Tower you have entered:
var range = 1
This should actually be:
var range: Int = 1
So with everything corrected the code should be as below:
struct Point {
let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
func surroundingPoints(withRange range: Int = 1) -> [Point] {
var results: [Point] = []
for xCoord in (x-range)...(x+range){
for yCoord in (y-range)...(y+range) {
let coordinatePoint = Point(x: xCoord, y: yCoord)
results.append(coordinatePoint)
}
}
return results
}}
let coordinatePoint = Point(x: 2, y: 2)
coordinatePoint.surroundingPoints()
class Enemy {
var life = 2
let position: Point
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseHealth(factor: Int) {
life = life - factor
}}
class Tower {
let position: Point
var range: Int = 1
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}}
Hope that helps but let me know if you have any questions.
Nikki