Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial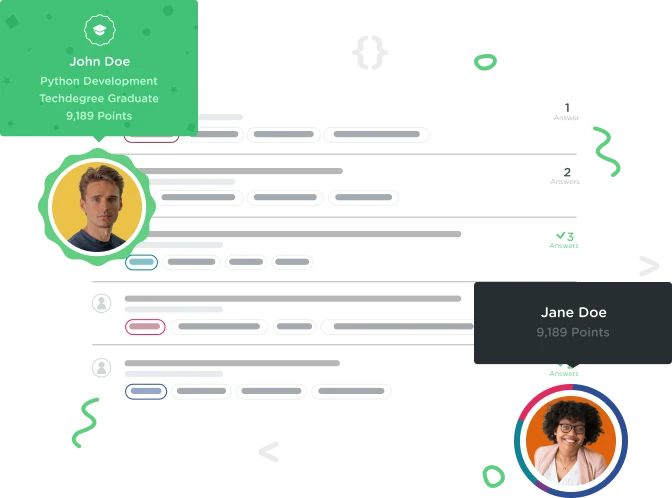
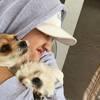
Carlos Marin
8,009 PointsYatzy help!!! I not sure if I understand the objective quite clearly.
I am checking all of the dice values and checking to see if any of them were rolled 5 times. if so, then I return the integer 50. else, I return 0. am I misunderstanding the objective, or is my code faulty?
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
list_of_die_values = []
for worth, quantity in hand._sets.items():
list_of_die_values.append(worth*quantity)
return sum(list_of_die_values)
def score_yatzy(self, hand):
five_point_combo = 0
for _ in hand._sets.values():
if _ == 5:
five_point_combo = 50
else:
five_point_combo = 0
return five_point_combo
2 Answers
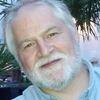
Jeff Muday
Treehouse Moderator 28,716 PointsSince we know that in order to score a yatzy, all the dice must be the same as the first one. And the hand inherits from the native Python list object, it is pretty easy to compute. We can get the value of the first die in the hand by accessing hand[0], then we check the every die in the hand to see if it equals the first die.
The weakness is that we don't check if the hand is 5 dice, but one could add that check too.
Good luck with Python!
def score_yatzy(self, hand):
first_die = hand[0]
for value in hand:
# if any dice are different from the first_die, return 0
if value != first_die:
return 0
# we know all dice are the same, return a 50
return 50

KRIS NIKOLAISEN
54,968 PointsYou could do it the way you have it as well. However in your loop you will only want the if statement. Since you loop through values for 1 to 6 if you include the else statement, you'd only score 50 if the hand was 5 sixes. Any other Yatzy and five_point_combo
would be set to 0 on the final iteration. So remove the else statement and you should be good.
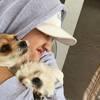
Carlos Marin
8,009 PointsHey KRIS, thanks for the information. Makes total sense, glad I got quick help.