Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial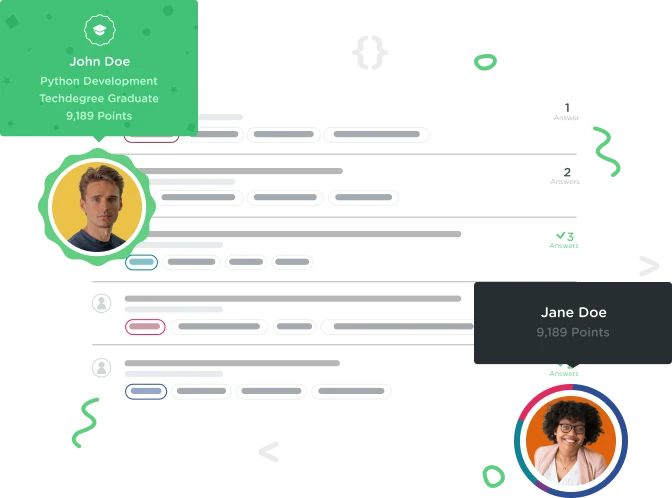
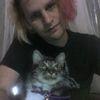
Bryan Wiedeman
10,203 PointsYet another null pointer exception - DayAdapter ConvertView is null
Like a lot of ppl i'm getting a null pointer exception when I click DAILY. It errors on line 49 of Day adapter which is
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
which is the same as in the package files. i ran the debugger and all i found out was what I already knew, that the convertView was coming up null. here's my DayAdapter, let me know if theres any other info that could be valuable.
package com.example.paxie.stormy.Adapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.paxie.stormy.R;
import com.example.paxie.stormy.weather.Day;
/**
* Created by paxie on 9/17/15.
*/
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; //we aren't going to use this. tag items for easy reference
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return null;
}
private static class ViewHolder {
ImageView iconImageView; //public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
4 Answers

Seth Kroger
56,413 PointsYou're forgetting to set mContext in the constructor, which will cause LayoutInflater.from(mContext) to throw a NullPointerExecption.
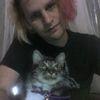
Bryan Wiedeman
10,203 Pointsi have
private Context mContext;
at the top. is there someone else it needs referenced?

Seth Kroger
56,413 Pointspublic DayAdapter(Context context, Day[] days) {
mContext = context; // <--needs to be set here.
mDays = days;
}
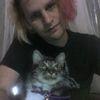
Bryan Wiedeman
10,203 Pointsi see now. i made that change and am getting a new error though
10-14 15:48:22.018 1040-1040/com.example.paxie.stormy E/AndroidRuntime: java.lang.NullPointerException: Attempt to invoke virtual method 'int android.view.View.getImportantForAccessibility()' on a null object reference
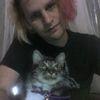
Bryan Wiedeman
10,203 Pointsok nvm figured that one out. had to change return null to return convertView. Thank you!