Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial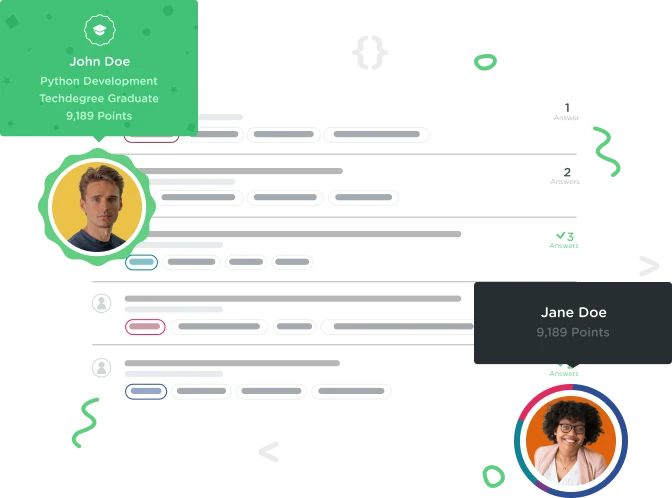

Kristen Thayer
5,501 Points'You're missing the "Person.call"'... except I'm not.
This is my code, but I keep getting an error message... any ideas?
function Teacher(firstName, lastName, roomNumber) { Person.call(this, firstName, lastName); this.room = roomNumber; }
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.fullName = function() {
return this.firstName + " " + this.lastName;
};
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.room = roomNumber;
}
function Teacher(firstName, lastName, roomNumber) {
this.firstName = firstName;
this.lastName = lastName;
this.room = roomNumber;
}
2 Answers
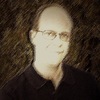
Jason Anders
Treehouse Moderator 145,860 PointsHey Kristen.
First off, for this challenge it's important to know that the person.js
file does not get altered or added to at all, so all the code you will write will be only in the teacher.js file. So, delete any code you added to the `person.js' file.
So in the teacher.js
file, you are on the right track, but you don't need to 're-declare' the firstName and lastName variables as this is done in the Person construct. You will only need to declare the unique variable for the Teacher. So, for the first part of the challenge, you are to call
the Person constructor in the Teacher constructor like so
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.room = roomNumber;
}
For the second part of the challenge, you just need to add the line of code so that the Teacher can inherit from the Person successfully.
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.room = roomNumber;
}
Teacher.prototype = Object.create(Person.prototype);
It's been quite some time since I've done JavaScript, so I may not have the most clear explanation, but Andrew does a really good job of it in the video just prior to this challenge starting at about 2:30.
Hope this helps some. Keep Coding! :)

Kristen Thayer
5,501 PointsThanks! I've got it now :)