Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial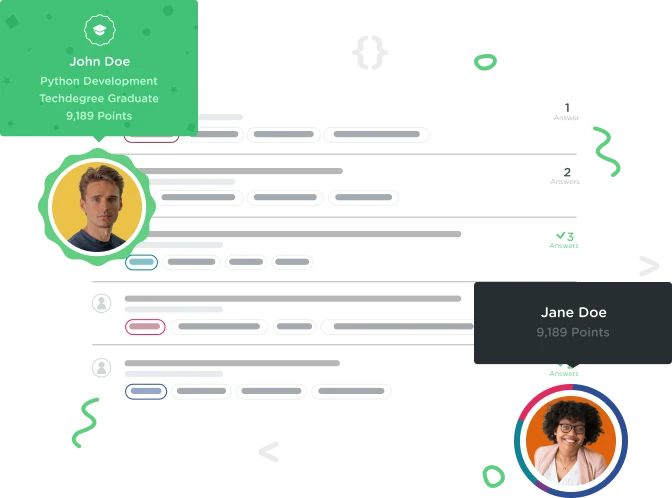
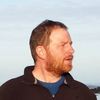
Nathan Clevenger
Courses Plus Student 17,759 PointsYoutube video continues to play after closing the Video Modal window
Has anyone else noticed that the video continues to play in the final version of this project? I'm a little curious how to make that stop, perhaps someone already knows how to fix it and could tell me. Thanks!
1 Answer
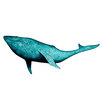
Deoward Kotze
14,604 PointsHi Nathan,
You need to use the YouTube iFrameAPI
Here is a quick example of what it might look like
This is the iframe you use to embed the video you have to make sure that you have this part "enablejsapi=1&origin=http://youwebsite.com" since it enables the javascript api and sets the origin
<iframe id="drills_player" width="560" height="315" src="http://www.youtube.com/embed/nkMiZEN5-Qk?enablejsapi=1&origin=http://yourwebsite.com" frameborder="0"></iframe>
You the need to load the api asynchronously
var tag = document.createElement('script');
tag.src = 'http://www.youtube.com/iframe_api';
var firstScriptTag = document.getElementsByTagName('script')[0];
firstScriptTag.parentNode.insertBefore(tag , firstScriptTag);
Then you need to create a player object, it's best to do it this way because if you have multiple players you can just add them to this object and all of them should work without adding listeners for each of them, you can call them whatever you like as long as it matches the id of your iframe
var player = {drills_player: {name: "drills_player", myplayer: ""}};
This refers back to the id of your iframe so make sure they are the same, then registers a listener to that player object (this is useful for when you want the player to reset when it's done playing instead of showing that crappy playlist)
function onYouTubeIframeAPIReady () {
for (var key in players) {
if(players.hasOwnProperty(key)) {
players[key].myplayer = new YT.Player( players[key].name , {
events: {'onStateChange': onPlayerStateChange
}
});
}
}
};
You then create the function that will be called when the state of the player changes
function onPlayerStateChange(e) {
for (var key in players) {
if (players.hasOwnProperty(key)) {
if (e.data == 0) {
players[key].myplayer.seekTo(0 , true);
players[key].myplayer.pauseVideo();
}
}
}
}
if you want the video to stop playing when the modal window is closed you can do something like this, this will look for the id of the iframe and use it to call the seekTo & pauseVideo methods of the corresponding player (because the id and YT.player object should be named the same)
function closevids($player) {
players[$player].myplayer.seekTo(0 , true);
players[$player].myplayer.pauseVideo();
}
$('.close').click(function () {
var $player = $(this).parent().siblings().find('iframe').attr('id');
closevids($player);
});
So the same things happens when you click outside of the modal
$('.bs-modal-lg').click(function () {
var $player = $(this).find('iframe').attr('id');
closevids($player);
});
Hope this helps you out, let me know if it does the trick for you