Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial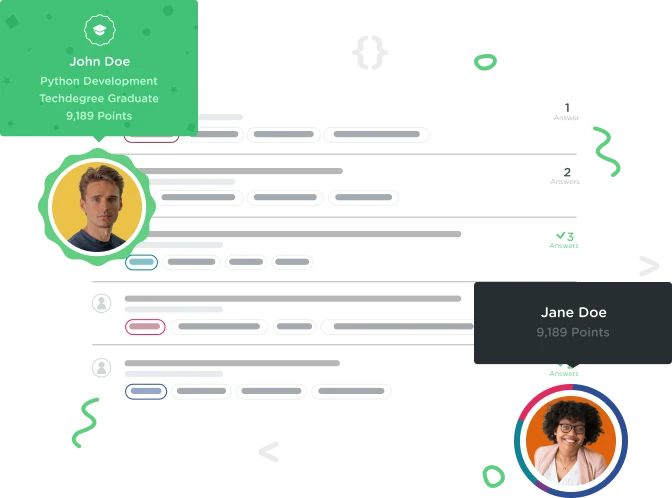
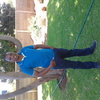
MUZ140063 Glen T. Moyo
5,257 PointsYou've got the $.get() method and first argument in place. The second argument to the $.get() method is a callback funct
You've got the $.get() method and first argument in place. The second argument to the $.get() method is a callback function. Pass an anonymous function as the second argument to the method. Don't forget to set a parameter for that function to catch the server's incoming response
$.get("footer.html");
$.get("footer.html", callback=function(response){});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
</head>
<body>
<div id="main">
<h1>AJAX with jQuery</h1>
</div>
<div id="footer"></div>
<script src="jquery.js"></script>
<script src="app.js"></script>
</body>
</html>
2 Answers

Andrew Barrette
Courses Plus Student 2,219 PointsThey are looking for an anonymous function, meaning a function that is not named.
// two normal, named functions:
function foo (param) { }
bar = function (param) { };
// anonymous (unnamed) function:
function (param) { }
Therefore, when you pass an anonymous callback function to the get method, simply don't name it. The get method already knows that the second parameter is supposed to be the callback function anyway.
$.get("footer.html", function (response) { });
Cheers!

Raymond Wach
7,961 PointsRemember that an anonymous function is a function without a name. In Javascript, functions are first-class which means that they can be treated as values and assigned to variables or passed as arguments to functions. To assign an anonymous function to a variable, you use the regular variable declaration and assignment syntax.
var foo = function () {
console.log("This is an anonymous function.");
};
However, the only part that is the anonymous function is the actual function declaration (the part after and including the keyword function
).
function () {
console.log("This is an anonymous function.");
}
Note, that we don't do anything with the anonymous function, we just declare it in place and don't save the value. To pass an anonymous function as an argument, just include the anonymous function as a function literal in the arguments to the function.
bar(function () {
console.log("This is an anonymous function passed as an argument.");
}); // Note, we close the parameter list of `bar` here.