Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial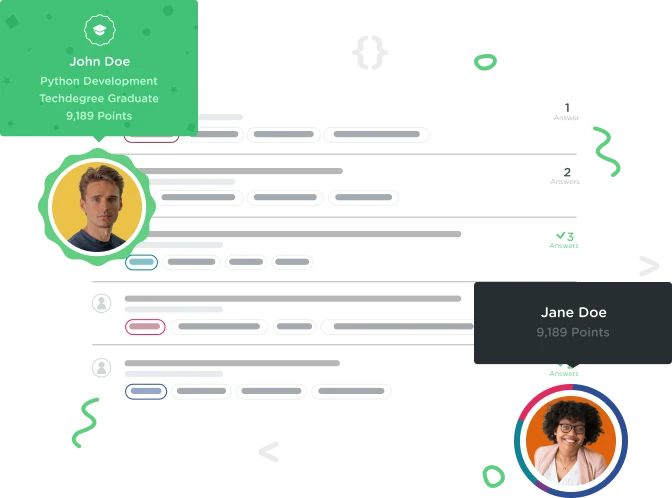

Jessica Smithers
Python Development Techdegree Student 227 PointsZeroDivisionError not working?
Just for fun, below my ValueError exception, I tried adding another exception for a ZeroDivisionError. However, if I put in 0 for the number of people who will be splitting the check, I still get the error and the exception doesn't run. Why does my first exception (ValueError) work correctly while my second one (ZeroDivisionError) fails to run the code and instead kicks to the else statement, thus continuing to produce a ZeroDivisionError?
Here's my code:
import math
def split_check(num, amt):
return math.ceil(amt / num)
try:
total = float(input("How much was the total bill? "))
persons = int(input("How many people are there? "))
except ValueError:
print("Oops! Invalid value!")
except ZeroDivisionError:
print("Invalid input, you need 2 or more people.")
else:
print(f"Each person owes {split_check(persons, total)} dollars!")
1 Answer
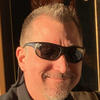
Peter Vann
36,428 PointsThis works as you intended it, I believe:
import math
def split_check():
try:
total = float(input("How much is the total bill? "))
persons = int(input("How many people are there? "))
each_tab = (total / persons)
print_string = "Each person owes ${:,.2f}".format(each_tab)
print(print_string)
except ValueError:
print("Oops! Invalid value!")
except ZeroDivisionError:
print("Invalid input, you need 2 or more people.")
split_check()
With 0 persons, "Invalid input, you need 2 or more people." prints
With H for either input, you get "Oops! Invalid value!"
If you input 100.00 for the check, and 4 for the number of people, "Each person owes $25.00" prints out.
By the way, you can easily test it here:
https://www.katacoda.com/courses/python/playground
I hope that helps. Stay safe and happy coding!