Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial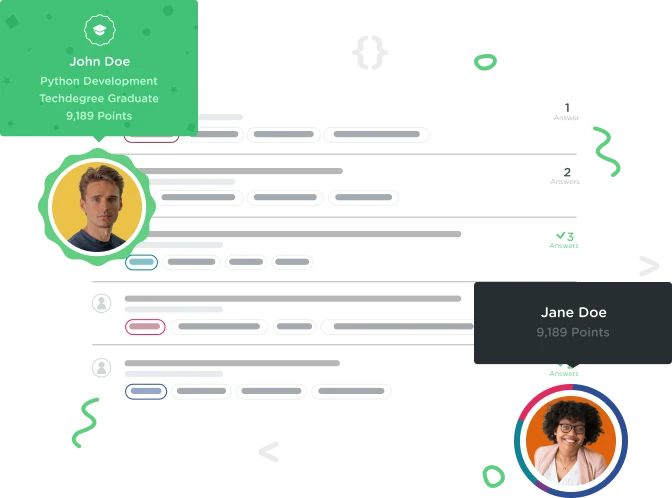
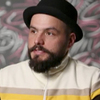
sergio verdeza
Courses Plus Student 10,765 Pointsstage 3 Logical Operators ruby
Lost on this question :( Help..........
9 Answers
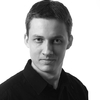
Maciej Czuchnowski
36,441 PointsFirst you need to understand what check_speed
is and what car_speed
is. check_speed
is the name of the function, so you only use it OUTSIDE of that function to run it. car_speed
is the name of the argument that you are passing in and you will be using it INSIDE of the function. So you are quite close, but you're comparing the wrong thing - the function instead of the argument.

uvwitpmluq
14,915 PointsI had a same issue. I note it just in case.
def check_speed(car_speed)
# write your code here
if car_speed > 39 && car_speed <= 50
return "safe"
end
end
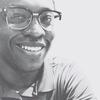
Ricardo Hill-Henry
38,442 Pointsif arg1 (insert logical operator) term1 && arg1 (insert logical operator) term 2
Do some magical Ruby 1337
end
The goal is to perform some type of action if two terms in a conditional are evaluated to be true. If either condition one or condition two are evaluated to be false, the code inside will not be run.
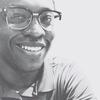
Ricardo Hill-Henry
38,442 PointsYour use of the operator is right, but you aren't comparing the right terms. You're calling and checking the method check_speed within your conditional for itself. If your method doesn't return anything, then this doesn't make sense - your method check_speed holds no value so it will never be greater than 39 or less than or equal to 50.
Instead, what you want to check, is the argument passed into your method, and return the the string "safe" if the conditional is satisfied (true).
Example:
def check_speed(param1)
if param1 > value1 && param1 <= value2
return "w0w such ruby sweg"
end
end

Jac Watson
779 PointsGreat job Ricardo!
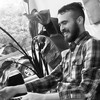
Sage Elliott
30,003 PointsNever mind I got it! I was missing the extra "end"! *Facepalm *

sebastien penot
3,292 PointsTry this:
def check_speed(car_speed)
if car_speed >= 40 && car_speed <= 50
return "safe"
end
end
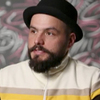
sergio verdeza
Courses Plus Student 10,765 PointsGuys thank for all your help!
Here is what I understood from explanations:
def check_speed(car_speed)
if car_speed > 40 && car_speed <= 50
return "Safe"
end
end
The check_speed method isn't producing the correct output. Isn't the value of the method check_speed(car_speed)?
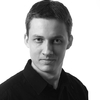
Maciej Czuchnowski
36,441 PointsYou're almost there: just change the capital S
to lower s
and add one missing =
symbol in the if
statement :)
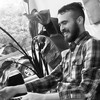
Sage Elliott
30,003 PointsDid you ever figure it out? I'm having the same issue...
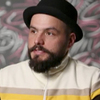
sergio verdeza
Courses Plus Student 10,765 PointsSage sorry for the late response: Here is how I got it to work:
def check_speed(car_speed)
if car_speed > 39 && car_speed <= 50
return "safe"
end
end
You can see the param1 and the value1 was what was giving me a problem.
Best of luck.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsAlso, make sure that you substitute this line:
puts "Do some magic Ruby"
with what they are asking you -Set the return value (...) to the string "safe"
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsAnd make sure you have the proper number of
end
statements.