Well done!
You have completed Auto User Search with JavaScript - Treehouse Live!
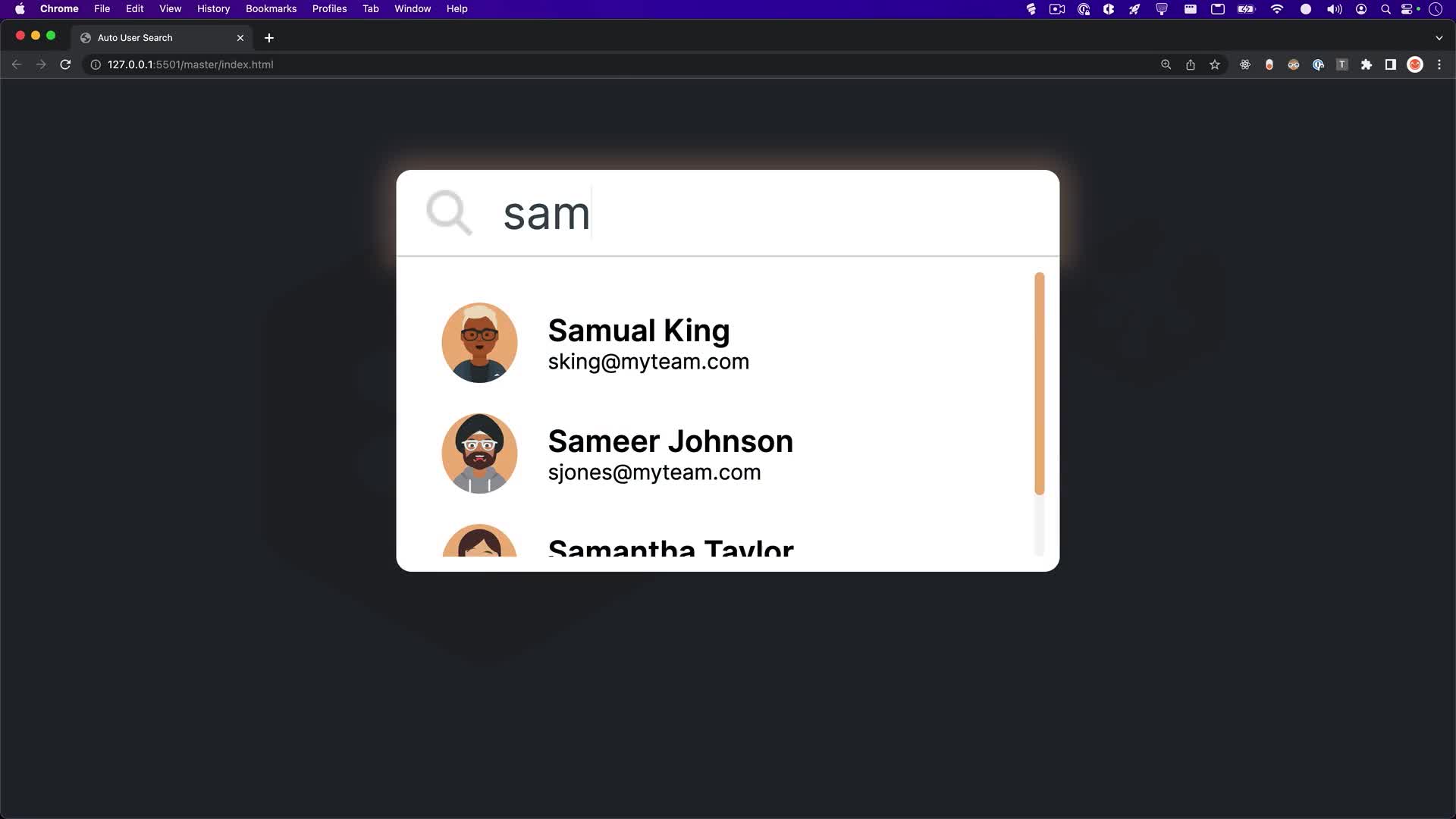
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Need practice working with the Document Object Model (DOM)? This project showcases some of the core concepts needed to interact with and manipulate the DOM. Follow along as Dustin builds a searchable input field that filters users based on your search query!
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up[MUSIC] 0:00 Hey, what's up? 0:09 My name is Dustin, and I'm a developer here at Treehouse. 0:10 Being able to search through your website or app, 0:13 is a super important feature that you can implement. 0:15 I'll show you one simple, 0:18 low-level way that we can add this to an existing project, or a brand new project. 0:19 Let's take a look at what I built. 0:23 Sometimes you may want to search through data on your website, or 0:25 maybe just a certain post on your app. 0:29 In this example, 0:31 I wanted to search for a specific user from a list of any user in my user base. 0:32 Take a look at how this works. 0:37 As soon as I start typing a drop down menu appears. 0:38 And it's populated with any user in my user base that contains the characters in 0:41 my search field. 0:46 We'll be building this project from scratch. 0:47 So the only prerequisites that I would mention would be to have a basic 0:49 understanding of HTML and CSS, and of course, a little bit of JavaScript. 0:53 We'll be working closely with the DOM as well as some loops in this project. 0:57 So, if you feel like you want to follow along with me, 1:02 grab the link in the teacher's notes, or description down below for 1:04 the GitHub repo, and let's get started. 1:07 Okay, so, just head on over to the description or teacher's notes for 1:09 the GitHub link, or you can just go to github.com/treehouse/auto-user-search. 1:15 And once you're there, you just wanna hit this big code button. 1:22 And in the drop down, you just wanna hit this copy button. 1:24 You can use GitHub desktop or your terminal to clone down the project. 1:27 I'm gonna use my terminal. 1:31 So I'm gonna open that up, type in cd desktop, and 1:32 then I'm just gonna type in git clone, and then paste in that link that we copied. 1:36 And then I'm just gonna drag this to VS Code to get started. 1:41 So let's take a look at the files and folders that we have in here. 1:46 You'll notice a master and a starter folder. 1:50 Inside master is all of the completed code in case you get lost or 1:53 stuck along the way and you need to refer to it. 1:57 But everything that we'll be using is gonna be in the starter folder. 1:59 So let's take a look at what we have in here. 2:02 All the way at the bottom of that folder, we have our index.html file. 2:06 And in here we are just linking our app.css file as well as our app.js file. 2:10 So let's go and take a look at those. 2:16 Inside of our styles folder we have our app.css and 2:20 we're just importing a Google font, we're resetting the browser just a little bit. 2:23 And we have a few CSS variables to style up the UI. 2:28 And inside of our scripts folder, we have an app.js file. 2:34 And in here is just one variable for an array. 2:38 And we call that userData, and it just holds a bunch of objects containing all 2:42 the data we need for each user. 2:47 So we'll be looping over this whenever we get to 2:48 the JavaScript portion of this project. 2:51 So the only other thing that we have in this starter folder that we should go 2:53 over is the images. 2:58 So in here we have a another folder for users. 2:59 And these are just some fun images that I have made that we can use for our users. 3:01 And then we have our mockup, which is what we'll be building out. 3:07 And then we have our search icon. 3:10 Okay, so that covers it for all the files and folders in this repo. 3:14 Let's get started actually coding. 3:18 The first thing I wanna do is just open up the index.html file as well as our markup. 3:21 And I'm gonna open them up side by side. 3:28 And let's see if we can't write the markup that we'll need to build this out. 3:30 So let's make the editor just a little bit smaller. 3:36 Okay, so let's break down this mockup. 3:40 It looks like we have one container and it's not centered, but it's sort 3:43 of at the top of the page, and we have a search field, and then a drop down menu. 3:48 So I think what I'll do is I'll create auto-user-search container. 3:54 And I'll put everything in here. 4:00 So first thing I wanna do is let's create a div called search-container. 4:02 And I'll first put in our image of our search icon. 4:08 So I'll navigate to images and then search.png. 4:13 And for the alt I'll just put an icon for our search input. 4:17 And after that I'll just go ahead and put our input with the type of text. 4:26 And I'll give it an id of searchInput. 4:31 And I'm gonna remove the name attribute, we won't need that. 4:36 And I'm also going to give an id to our search-container, 4:40 because we will need this in the JavaScript later also. 4:43 So I'll just call this searchContainer. 4:47 Sweet, so 4:51 now I guess let's also put our drop down menu inside of the search-container. 4:52 So I'll create a div with a class of .dropdown-container. 4:58 I'll also give it an id of dropdownContainer. 5:02 And in here, I'll just have a ul called dropdown. 5:07 And also give this an id, we'll need this in the JavaScript also. 5:11 And I'll just call this dropdown. 5:16 Now, all these list items will be rendered dynamically, so 5:19 we're gonna loop over that userData array in our JavaScript file. 5:23 But, in order to style this out, I want to create these statically. 5:28 So, I'm gonna create a list item, and in here I'm gonna put an image. 5:32 And for now I'm just gonna navigate to one of the random images in here, 5:38 and we don't need to worry about the alt attribute, 5:43 since this will be done dynamically later. 5:47 And then I'll just create a div with the class of .group, and 5:50 I'll put in a <p> for our username. 5:54 And I'll copy that down and replace the username with an email. 5:59 I'll right sjohnson@myteam.com. 6:03 Okay, so I think that's it that we'll need for the list item. 6:12 So let's copy that and create a few more of these. 6:15 And I'm gonna close each of these up so 6:19 that we can see our markup a little bit better. 6:22 Actually, I think that's all we'll need for the markup. 6:25 So let's close down each block and let's review what we've written. 6:27 So, inside of our body we just have one child element, 6:32 which is our auto-user-search container. 6:35 And inside of here we have our actual search-container. 6:37 And inside of the search-container holds everything. 6:41 We have our image for our search icon, 6:43 we have our input, we have our dropdown menu. 6:47 So I think that's everything. 6:51 Let's go ahead and save this. 6:52 And I'm gonna move the mockup back to the side with the HTML file. 6:54 I'm gonna open up the Explorer window and 6:59 I'm gonna open index.html with live-server. 7:01 Okay, so we have our live server open. 7:05 And it looks pretty bad right now. 7:08 So we need to style this. 7:10 But what's important to note is it looks like we have everything we need. 7:11 According to the mockup, we have our search icon, we have our search-container, 7:15 and we have our list items, and our list items have a name and a email address. 7:21 So, let's start styling this up. 7:26 Just so that we can kind of see everything better, I'm gonna hop back into our HTML 7:30 file really quickly, and I'm gonna comment out the dropdown-container. 7:34 And we can come back and 7:39 uncomment this out once we're ready to start styling that. 7:40 So, let's open up our style sheet. 7:45 Let's go to starter/styles/app.css. 7:47 Awesome, so the first thing I'll do is, I'll just create a rule for the body. 7:53 And I'll give it a background-color. 7:58 And I'm gonna use a CSS variable for this. 8:03 And if you're unfamiliar with how CSS variables work, 8:05 you just write the var keyword with a set of parentheses, 8:08 you copy the variable that you wanna use, and you paste it in. 8:12 And it's important to note that all CSS variables start with two dashes. 8:15 So I'll hit save and you'll notice our darker background color now. 8:20 So, let's start styling this up. 8:24 I'll do a min-height of 100vh for the body. 8:27 I'll give it a display of flex and I'll justify-content center. 8:30 And it looks like in the mockup it's a little bit off from the top. 8:38 So, what I'll do is I'll target our auto-user-search and 8:41 I'll just give it a margin top of maybe 3rem. 8:47 Cool, I think that looks good. 8:52 So let's also give it a background color. 8:54 I have a CSS variable for this called --white and I think that looks pretty good. 8:57 Why is this so big? 9:06 Let's see. 9:10 Okay, so this needs to be in our search-container. 9:15 So let's remove that background color, and let's create a new rule for 9:19 our search-container, and then paste that in there. 9:22 There we go, that looks much better. 9:25 I'll give it a border radius of .5rem. 9:28 I'll give it some box-shadow. 9:33 And I have a CSS variable set up for this too, it's called --box-shadow. 9:34 And I'll give it a little bit of padding, we can do .25rem. 9:41 Think that looks pretty good. 9:45 Now let's target our input and our image. 9:47 Actually we have more than one image in this container, so let's give our 9:56 Let's give the search-icon 10:03 a class of search-icon. 10:09 Save that, and we'll come back to the search-container, 10:14 and we'll do image with the class of search-icon. 10:20 So let's open these two rules up. 10:24 And for the search-icon, let's give it a width of 1rem, 10:27 which I think looks pretty good. 10:31 And we'll do a margin of auto on the top and bottom, and maybe 1rem left and right. 10:33 That's way too much, let's do maybe 0.25rem, left and right. 10:40 I think it looks okay. 10:45 Let's also give a display of flex to the search-container, 10:46 and we'll align the items center. 10:51 And that's gonna kinda put the search icon in the input container on the same line, 10:53 or more centered together, should say. 10:59 So great, that looks pretty good. 11:02 Let's target the input, and we'll give this a border of none and 11:04 an outline of none, and the font-size, we'll up to 1.3rem. 11:09 And we'll do a padding-left of 0.5rem 11:18 to space it a little bit more away from the icon. 11:23 Great, I think that looks pretty good. 11:29 One thing though is the auto-user-search. 11:31 Let's give it a width of 80%. 11:34 But let's give it a max-width of 350px, 11:37 Which I think looks pretty good. 11:45 But now our input element is outside of the container, 11:46 so let's give our input a width 100%. 11:51 Great, so I think this is looking pretty good. 11:55 Let's also give it a color. 11:57 We have a CSS variable named --text for this. 12:00 And I'm actually gonna copy this property and 12:04 I'm gonna set this on the body as well, so that everything has the same color. 12:07 Awesome, you might not notice it, but 12:12 it's just a little bit lighter than just pure black. 12:14 Okay, so I think that's actually it for the input, as well as the image. 12:17 So let's style up the, well, let's take a look at the mockup. 12:23 Okay, so it actually does not have a border-radius on the bottom right and 12:29 bottom left. 12:34 And what I think we can do is we can keep the border-radius as is, but 12:35 when the dropdown is open, we can remove that border-radius so 12:40 that the dropdown looks like it's part of this input. 12:45 So what we can do is, let's give our search-container a class of expanded, 12:49 so we'll do search-container when it has the class of expanded. 12:57 And when the dropdown menu is expanded, 13:03 we want the search-container to have a border-radius of 0.5rem on the top left, 13:06 0.5rem on the top right, and 0 and 0 on the bottom left and bottom right. 13:12 So let's check this out. 13:17 We'll give the search-container a class of expanded, sweet, 13:18 and it removes the border-radius from the bottom left and right. 13:23 That's awesome. 13:28 Okay, so we'll keep that on for now in the HTML because 13:29 we're gonna style up the dropdown menu next. 13:35 So let's go back to our stylesheet, and 13:39 let's target the dropdown-container. 13:44 What we'll do is we'll give this a padding. 13:48 Actually, we need to uncomment this out, it was commented out in HTML. 13:50 So we're gonna put it back into the mockup. 13:56 And instantly, everything's messed up, cuz these images are pretty big. 13:59 So let's actually target these images really quickly and 14:02 give them a smaller width. 14:07 So we'll do dropdown-container and we could 14:09 just target all the images inside of there and give them a width of 1rem for now. 14:12 Sweet, I think that looks pretty good. 14:17 So one thing that I do wanna mention is that this dropdown menu is gonna be 14:20 positioned absolute, so let's handle that now. 14:25 Inside of the dropdown-container itself, 14:28 let's give it a position of absolute, the top of 100%, and 14:32 a left of 0, and we'll also give it a width of 100%. 14:37 And you'll notice that it's completely gone. 14:41 It's all the way down here now, and that's because we're positioning this absolute. 14:44 And since its parent does not have a position of relative, 14:49 its absolute to the body. 14:54 So its parent, which is the search-container itself, 14:56 we're gonna put a position of relative. 15:00 And now you're gonna see the dropdown menu is now underneath the input. 15:04 So let's go back to the dropdown-container, and 15:09 we'll give it a background color. 15:13 We'll do our --white CSS variable. 15:16 And let's see, we'll also give it some padding of maybe 0.5rem. 15:21 And what we can do is target the dropdown, 15:28 actually, the ul with the class of dropdown, 15:32 and we'll give it a maximum height of 125px. 15:37 And now we see the overflow outside of the container. 15:42 And the easiest way to fix this would be to give it a overflow property of auto. 15:46 And now we'll have this little scrollbar. 15:53 And we can actually style up the scrollbar, but it's important to note that 15:55 the styles that I'm about to use for the scrollbar will only work on Google Chrome. 15:59 It's not gonna work on Firefox, so 16:04 you might not see the same thing if you're using Firefox. 16:06 But what we can do is we can target the ul with the dropdown class. 16:09 And we can do ::webkit-scrollbar. 16:14 And we can give it a background-color, 16:20 and we'll use --gray-light. 16:25 And we'll give it a border-radius of 25px, 16:30 and we'll give it a width of maybe, let's do 10px. 16:35 That is way too much width. 16:41 Let's do 5px. 16:43 I think 5px looks pretty good. 16:44 So it might be hard to see, this is actually the scrollbar track, and 16:47 we can actually target the thumb for it as well. 16:51 So we can write ul with the class of dropdown, 16:55 and we'll type in ::scrollbar-thumb. 17:01 And all we'll do with this is give it a background-color. 17:05 And we'll use our --primary color, which is a light orange, and 17:09 we'll give it a border radius of 25px. 17:14 And now we have a pretty neat looking scrollbar. 17:16 I think it's still a little wide. 17:21 Let's maybe do 3px in width, keep it kinda skinny, or maybe 4. 17:22 I think 4 looks pretty good. 17:29 Okay, sweet. 17:30 So now let's start targeting these list items inside. 17:32 And actually, we're gonna want to give bottom border-radius for 17:39 this dropdown menu, because it is round on the bottom as well. 17:44 So, This would be on the dropdown-container, 17:48 so we can do border-radius. 17:54 We'll do 0 for the top left, 0 for the top right, 0.5rem for 17:56 the bottom right, and 0.5rem for the bottom left. 18:00 Sweet, and now we have our rounded borders. 18:04 Awesome, so let's work on the list items now. 18:08 We can target, The ul with 18:12 the class of dropdown, and we'll target the list items inside. 18:17 And the first thing I wanna do is just give them a display of flex, and 18:21 we'll align the item center. 18:26 And what we can do now is, Okay, 18:28 so this image right here that's targeting these images, let's keep it consistent. 18:34 We'll do a ul with the class of dropdown, and we'll just target the list item image. 18:40 I'll hit Save, and we shouldn't see any differences, so that's good. 18:45 And now I wanna target the group. 18:48 So we'll do ul with the class of dropdown, li and 18:51 then the class group, and we want to put a margin-left of 1rem. 18:56 It might be too much, we'll see. 19:03 We're going to target the <p> inside. 19:08 And actually, we're gonna target each one individually, so we'll do :first-of -type. 19:15 So I wrote ul with the class of dropdown, li, we're targeting the group, and 19:21 we're targeting the very first <p> element. 19:25 And what we'll do with this is we'll just up the font-weight to maybe 500, 19:28 do font-size 0.7rem, and let's copy this down. 19:36 And we'll change :first-of-type to :last-of-type. 19:44 And we'll remove these properties, and we'll just lower the font-size to 0.5rem. 19:49 I think that looks okay. 19:56 So, now, let's go back to our images. 19:59 And what I'll do is I'll do a border-radius of 50% to give it a perfect 20:03 circle, and I'll do a background-color. 20:08 And we'll use our --primary color for this. 20:11 And now, we have our somewhat of an orange background color that matches 20:15 the rest of the UI. 20:20 I'm not liking the spacing on that margin-left, so let's do 0.5 rem. 20:22 And I think that looks pretty okay. 20:27 Let's also lower the email address font size. 20:29 Cool, I think that looks good. 20:37 So now for each list item, let's also give it a little bit of padding. 20:40 So we'll go back up to our list item rule, and we'll give it a padding of 0.5 rem. 20:44 And that's way too much, let's do 0.25 rem. 20:50 I think that looks pretty okay. 20:53 And we'll also give them a border-radius of .5 rem to be 20:54 consistent with everything else. 20:59 And I know what you're thinking, what's the point of a border-radius on that? 21:02 If there's no background-color, you can't see it, but 21:05 we will have a hover effect on this. 21:08 So let's do ul.dropdown li:hover. 21:09 And we just wanna target the background-color, 21:12 and we'll use our --gray-light CSS variable. 21:16 I'll hit that and now when we scroll over, we have a subtle background color change. 21:21 So let's actually transition our background color. 21:28 0.2 seconds ease, and 21:34 we're also gonna want to give a cursor a pointer to each list item. 21:36 And I think that looks pretty good. 21:43 So I think there's just a little bit too much padding on this left side, or 21:46 actually what I think that looks fine. 21:50 Let's add in a, or let's adjust the maximum height so 21:53 we can see what this looks like with the scroll bars. 21:58 So, URL, max height, let's do like 50 pixels. 22:06 Okay, cool. 22:10 So, our scroll bar still looks good. 22:10 Everything's looking pretty okay. 22:12 Sweet. So, let's check out the mock-up. 22:14 And this looks pretty close. 22:17 So it looks like there is a somewhat of a border up here or a divider. 22:19 And I think we can tackle that by giving our drop-down container a border-top. 22:24 So, let's look for our drop down container, which is right here. 22:31 We'll do border top, one pixel, solid and we'll use our, let's try grey dark. 22:35 There we go, I think that looks pretty good. 22:44 So, yeah, I think this is everything we need. 22:50 Let's go ahead and update our maximum height on our drop down again. 22:53 Set to 100 pixels, or maybe let's do like something like 75. 23:01 What about 65? 23:08 There we go. 23:10 That way you can kinda see there's another item there so it would let the end user 23:11 know that, hey, that you might need to scroll down for more options. 23:16 Sweet, so I think we're actually done with the UI. 23:21 So now what we need to do is render these items dynamically when a user 23:25 types in a name. 23:29 I take it back, there is actually one other thing we need to do in the styles so 23:31 by default this drop down menu is gonna be hidden. 23:35 So we need to go ahead and hide that. 23:38 If we look at our dropdown container rule, we'll see that the top is set to 100%. 23:41 So what we wanna do is we wanna have a dropdown-container.show class. 23:45 So I'll add a class of show to dropdown-container. 23:53 And we want the top to be that 100%. 23:57 And, we're gonna change the 100% in the original 24:01 dropdown-container rule to something like maybe 75%. 24:05 And, we're also gonna give it an opacity of zero, and pointer events set to none. 24:09 And, pointer events set to none basically removes any events that the pointer can 24:17 trigger as far as clicking, hovering, focus all that. 24:22 So with that done let's also give it a transition to transition these things so 24:25 that we have a smooth effect. 24:30 We can just type in transition and we can just write all and do 0.2 seconds ease and 24:33 that will transition everything, 0.2 seconds ease. 24:37 So in our show class, we need to adjust the top back to 100%. 24:42 And we need to bump the opacity back up to one and 24:46 give it access to all the pointer events again. 24:49 And we can do that by writing pointer-events: all. 24:52 So we can test this by going into the Developer Console. 24:58 And let's find our dropdown-container and give it a class of show. 25:05 And our dropdown menu shows up. 25:12 So that's awesome. 25:15 Let's also remove the expanded class from our searchContainer. 25:16 So I'll remove that and now we are left with the way our UI should look when 25:23 the page loads, which is just a search field. 25:26 Sweet. So now let's work on the JavaScript. 25:30 So what I'll do is I'll open up the app.js file. 25:35 And I'm going to open up the developer console again, and 25:39 I'm gonna head on over to the console tab. 25:42 And I think the first thing that I wanna try to tackle is seeing if I can get 25:45 whatever we type in this search field to show up in the browser's console. 25:49 So I do know that our input right here has an ID of searchInput, so 25:54 let's create a variable for that. 25:57 I'll type in const searchInput = document.getElementbyId('searchInput'): 26:00 and we want to listen for the keyup event on this. 26:07 So let's set up an event listener. 26:11 We'll do searchInput.addEventListener, and 26:13 we wanna listen for keyup and we want to grab the event. 26:17 And what we can do is log the value of this input to the console. 26:22 So let's set up a variable for this as well. 26:29 We can write, let value=e.target.value. 26:31 And let's log this to the console every time this event handler runs. 26:38 So we'll console.log(value) 26:42 So if I come over here and I start typing, hello world, 26:46 you'll see in the console that every single time that this input 26:50 detected a key up event it logged the value of the input to the console. 26:54 Which is exactly what we want because we're gonna wanna test what words, 26:59 and what letters are in here to match with our user data. 27:04 So let's go ahead and remove this console.log and 27:09 let's loop over our user data. 27:13 Or actually let's make sure our input is not empty. 27:16 So we'll do if value is not equal to an empty 27:19 string we want to first let's see. 27:24 The first thing we'll wanna do is probably 27:28 add that expanded class to the search 27:33 container as well as show our dropdown. 27:37 So let's set up some variables for those elements. 27:42 So I'll write const searchContainer=document.getElementById('searchContainer') 27:46 and 27:51 then I'll set up a variable for our dropdown container as well. 27:55 All right, const dropdownContainer = document.getElementById ('dropdownContainer') 28:01 28:04 So if we start typing into our search field and 28:09 the value is not empty, we want to take our 28:14 searchContainer.classList.add( 'expanded'). 28:18 And then we want to take our dropdownContainer.classList.add( 'show'). 28:24 So let's start typing and see if anything happens. 28:32 Sweet, we started typing and our drop down menu appeared. 28:36 So if we backspace it should go away, right? 28:42 Nope, we have not written the logic for that. 28:46 So, we need to write an else statement in our conditional. 28:48 So if we write else, we can just reverse these two statements. 28:51 So I'll just change add to remove for both of these. 28:57 I'll hit Save and I'll start typing, and then I'll backspace and 29:02 I'll start typing again. 29:06 And I'll backspace. 29:08 Sweet that works. 29:09 I am noticing that the border radius or the lack of border radius that is applied 29:11 to our search containers instant, so it kind of messes up the animation. 29:16 So let's see if we can hop back in the CSS, and 29:21 we'll scroll up to the searchContainer. 29:24 And we will give it a transition of border radius 0.2 seconds ease. 29:27 And now that should be a pretty fluid animation. 29:33 Sweet that looks so much better. 29:38 Awesome, okay. 29:41 And you're probably wondering why there's already people in here. 29:43 This is just the static markup that we wrote. 29:45 We'll be deleting this pretty soon and we'll actually loop over our data. 29:48 So we now have a conditional statement inside of our eventListener. 29:53 So our value is not empty we want to show our drop down menu, so 29:58 it would make sense that also in this first block of our conditional, we 30:03 start matching the users that are in our user data variable up here with our value. 30:08 So what we can do is we can loop over userData,so we can do 30:15 userData.forEach() and for each user what do we wanna do, 30:20 well for each user we want to add them to the page as a list item and 30:25 we want to append that list item to our ul, so 30:30 we'll need to grab this ul's id and reference it. 30:34 So I'm gonna copy this and while I'm in here, 30:40 I'm also going to remove all of these li's but one, and I'm only gonna' leave one in 30:42 here so that we can copy this markup here and a little bit. 30:46 So what we'll do is inside of this for each method, all right userData.forEach()_ 30:51 and we wanna write, well, we need to set up our variable first. 30:56 We'll type const dropdown = document.getElementById('dropdown') 31:01 and now we have access to it, 31:07 so we'll put dropdown.innerHTML += 31:12 And what we want to render is this markup here, so I'll cut this and 31:19 I'll leave a comment in the HTML called dynamic. 31:25 And now if we start typing, nothing actually shows up cuz we don't 31:31 have anything in there yet, so we will paste in that markup and 31:36 the easiest way to get the data that we need is to use interpolation. 31:41 So if we come up to our user data variable, 31:48 you'll notice that we have a name attribute, I'm sorry, a name property, 31:50 an email property and an image, so we'll be using these. 31:55 So each time that this for each method iterates over our array, 31:59 this variable that we set called user holds that current iteration. 32:05 So the first time it will hold the very first item. 32:12 So what we can do is in the source attribute, we can do user dot image, 32:17 and it will give us a reference to that current iteration of our array and 32:22 it will give us the image. 32:27 And for the alt attribute we can write a picture of, 32:30 and we could write user.name and for 32:36 the name we can also just write user.name, 32:40 and for the email, we can write, user.email. 32:46 And it's important to note that this string interpolation only works if 32:53 you're using backticks, so you have to use backticks right here. 32:57 So, basically, for each user, 33:02 it's going to automatically throw every one of our users in here. 33:04 Now, you're gonna see why this is not a good idea. 33:08 So, I'm gonna hit save, and if we start typing, 33:12 you're gonna see everyone's in here. 33:14 So, all we're doing now is just adding all these users each time the event 33:17 handler runs, which is not good. 33:22 Look how many users we have, we actually only have a few users in here, 33:24 we don't have that many. 33:28 So what's happening is each time this runs, 33:30 we're just adding and adding and adding to this unordered list. 33:34 So, What we should do is reset it each time. 33:40 So at the very top of our if statement, 33:44 we wanna do dropdown.innerHTML = ''; 33:46 And now no matter how many times we type, the list of users will never get any 33:51 bigger it'll always be our full list of users. 33:55 So that leaves us with one other problem, 33:58 is we want the users to match the search, so how do we do that? 34:01 Well, this problem is actually really easy, so inside of our four loop, 34:07 we can run another conditional to test if the value is equal or 34:13 includes any of the usernames. 34:17 So what we can do is, we can write if and then in here we can put user.name and 34:20 we wanna make sure it's in toLowerCase() so that we can always match the string. 34:26 So we'll do .toLowerCase() and we'll write that includes value, 34:32 and we'll also do .toLowerCase() on the value. 34:38 And if this is true, we want to run this bit of logic right here, 34:43 so I will paste that in. 34:49 Oops. 34:52 So I'll cut this and I'll paste this in the conditional, and I'll hit Save. 34:54 So now if I come over here and 34:59 I start typing in things It actually will start matching. 35:00 So if I type in Samantha,Samantha Taylor will show up, if I type in Sam, 35:05 we have Samuel King,Sameer Johnson and Samantha Taylor, if I type in Smith, 35:10 we have two people with the same last name Jay Smith and Kenan Smith both show up. 35:15 And if we just start typing, it will always match the person. 35:20 Sweet, so that about covers it for using just a little bit of JavaScript to add 35:26 a ton of functionality to your Web page. 35:29 I hope you were able to understand the relationship between the markup and 35:32 the JavaScript, and how we use the DOM to kind of tie it all together. 35:35 This is a great feature to add to your projects, even your old ones. 35:39 So I hope you build something really, really great with this, 35:42 I'll see you in the next one, and until then, have fun and happy coding. 35:45
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up