Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Start a free Courses trial
to watch this video
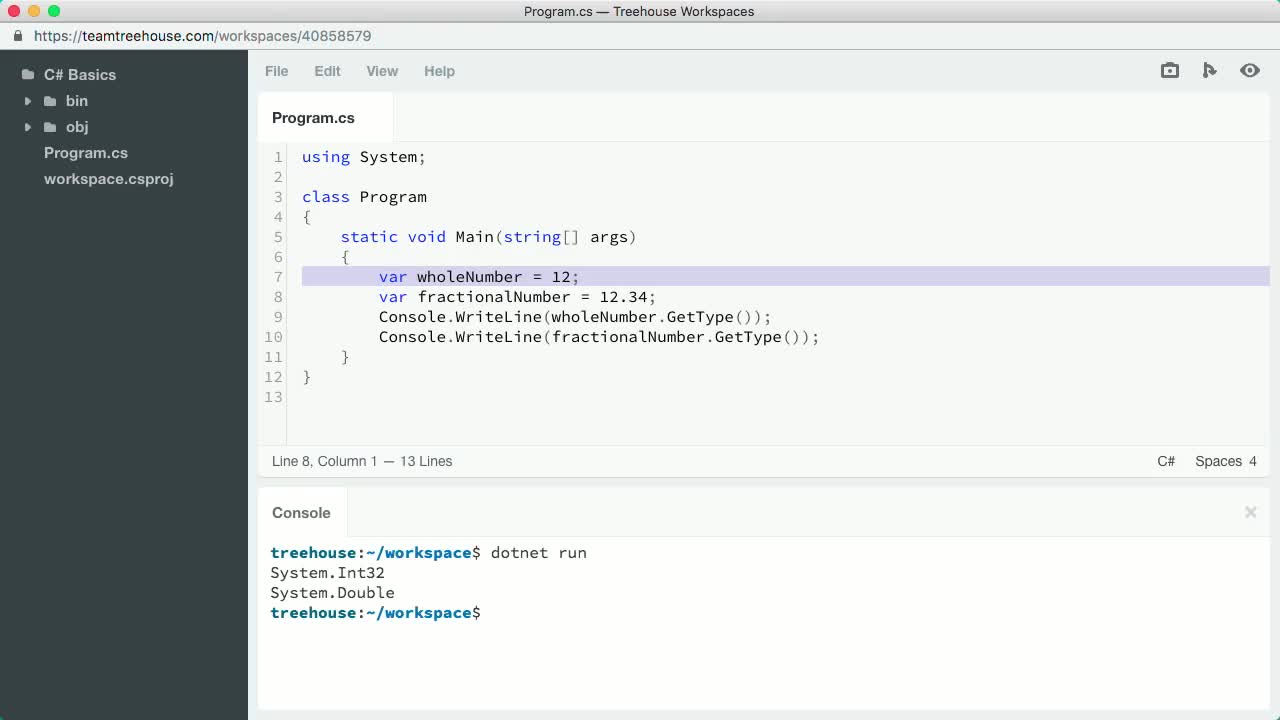
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
A conversion explicitly converts a numeric value of one type to another type.
Here we have some code that assigns the integer 12
to a variable named whole_number
, and the fractional number 12.34
to a variable named fractional_number
. That decimal point within the numbers is important, because it decides which of C#'s two most common numeric types the number will be treated as.
var wholeNumber = 12;
var fractionalNumber = 12.34;
Console.WriteLine(wholeNumber.GetType());
Console.WriteLine(fractionalNumber.GetType());
- The whole number automatically gets assigned a type of
Int32
, and the number with a decimal point gets a type ofDouble
. - As we saw in the previous video, even a number that's technically an integer will be treated as a
Double
if you include a decimal point.- Let's change the assignment to
wholeNumber
from12
to12.0
. - It's still technically the same number, but the decimal point changes its default type.
- You can see that the type for
wholeNumber
is now alsoDouble
.
- Let's change the assignment to
- Remember that
var
implicitly sets the type of a variable based on the value you initially set it to.- We can also explicitly set the type of our variables.
Let me set wholeNumber
's type to int
... And fractionalNumber
's type to double
:
int wholeNumber = 12.0;
double fractionalNumber = 12.34;
Console.WriteLine(wholeNumber.GetType());
Console.WriteLine(fractionalNumber.GetType());
- But this gives us an error when assigning to
wholeNumber
: "Cannot implicitly convert type 'double' to 'int'." What does that mean? - Well, this really doesn't seem to matter much when assigning
12.0
to an integer variable. But what if we were trying to assign12.5
or3.14
or some other number with a fractional part?int wholeNumber = 12.75
- For an integer variable to hold the
double
value, we have to convert it to an integer. - But what do we do with the fractional part, the .75?
- Does it just not matter? Can we drop it? That seems unlikely. You wouldn't want the fractional dollars dropped from your bank account, for example.
- To protect you from issues like this, C# won't let you assign a
double
value to anint
variable without converting it first. - A conversion explicitly converts a numeric value of one type to another type.
- A conversion shows the compiler that you know this is a
double
value, but you definitely want to convert it to anint
, and are certain that there either is no fractional portion to the number, or that losing the fractional portion won't cause any problems. - You do a conversion by placing parentheses before the value you want to convert, containing the type you want to convert the value to:
int wholeNumber = (int)12.75;
Conversions only work with numeric types. You can't convert from a string
to an int
or an int
to a string
, for example.
wholeNumber = (int)"12";
string myString = (string)12;
Cannot convert type 'string' to 'int'
Cannot convert type 'int' to 'string'
- There are often other ways to convert from one non-numeric type to another, but those are provided by libraries.
- Conversions, on the other hand, are provided by the language itself.
- We'll see how to convert from a string to a numeric type in an upcoming video.
Casting is an explicit conversion. It's required when making a conversion from a more-precise numeric type to a less-precise one, because data might be lost. Converting from a floating-point number to an integer loses all the decimal places, for example, so it requires an explicit cast.
But when you're converting from a less-precise numeric type to a more-precise one, there's no risk of data loss, because the more-precise numeric type will always be able to hold the full value from the less-precise one. For example, when converting from an integer to a floating-point number, you can just add ".0` onto the end. It consumes more computer memory to store it now, but it's still the same value.
When converting from a less-precise numeric type to a more-precise one, C# will do an implicit cast. An implicit cast is a conversion between numeric types that happens automatically, without the need to specify that it should.
- For example, suppose I wanted to change the initial value of
fractionalNumber
from12.34
to12
. - There's no need to write
12.0
. - I can just get rid of the decimal point and all the decimal places:
double fractionalNumber = 12;
- It still works, and the resulting value still has a type of
double
because I declaredfractionalNumber
with adouble
type.- C# does an implicit conversion from the less-precise
int
type to the more-precisedouble
type.
- C# does an implicit conversion from the less-precise
int wholeNumber = (int)12.5;
double fractionalNumber = 12;
Console.WriteLine(wholeNumber.GetType());
Console.WriteLine(fractionalNumber.GetType());
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up