Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Course Overview 2:16
- What Is a Game Engine? 1:59
- What Is Phaser? 2:43
- My Development Setup 3:30
- What Will Be Built in This Stage 1:27
- Phaser Project Setup 6:29
- Loading Game Assets 6:06
- Setting up a Server 5:51
- Adding Grouped Images 4:53
- Phaser Explanation and Setup Quiz 5 questions
- Adding Paddle Movement 6:05
- Adding Physics 9:36
- Adding Basic Collisions 4:40
- Adding Logic on Collision 5:05
- Winning and Losing Text 13:58
- Adding Sound 8:02
- Final Thoughts 9:55
- Player Movement and Collision Quiz 6 questions
- How to Improve Our Game 4:41
Preview
Video Player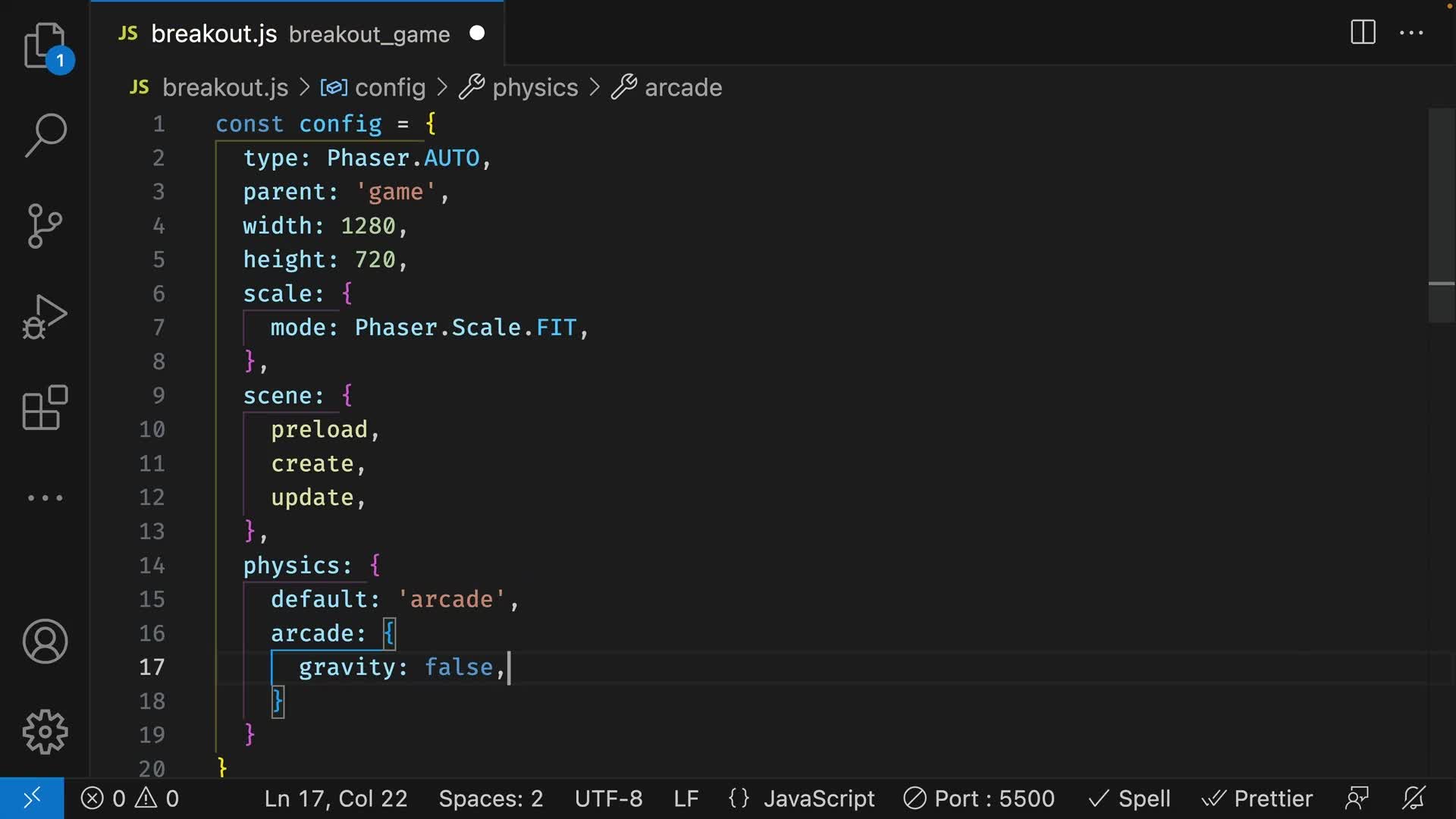
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Learn how to add physics properties to the ball in our breakout game so that it is affected by gravity.
Resources
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
In the last video,
we added some movement to our paddle, but
0:00
we realized our paddle
was going off screen.
0:04
In this video,
0:07
we're going to add some physics to our
game to prevent that from happening.
0:07
Let's make sure we're at
the top of our file, and
0:12
we can enable our page
physics in our config object.
0:15
We can do that by adding the following
code below the scene property.
0:19
Let's add a new property called physics,
and this is an object.
0:24
This is used to set the physics engine for
our game.
0:29
Let's open this object and
give it some properties.
0:34
We'll first give it
a property called default.
0:37
And we'll give this a value
of arcade as a string.
0:41
Arcade is the name of the lightweight
physics engine in phaser.
0:45
Below that, let's set some configuration
for the arcade physics engine.
0:50
We'll create a new property called arcade,
which is an object.
0:54
And inside this object we'll create
a another new property called gravity,
1:00
which accepts a boolean value.
1:05
Let's set it to false.
1:07
For this game we're not going to use
any gravity and I'll explain why later.
1:09
Cool, now let's scroll down to
the correct function and let's add some
1:15
physics to our paddle by using the physics
add method instead of just the add method.
1:20
We can do this by dragging
our cursor to the ad and
1:26
typing the word physics followed by a dot.
1:30
Beneath line 37,
let's add some screen boundary collisions.
1:34
We can do that by using a helper
method provided by the arcade
1:38
physics engine called Set
collide world bounds.
1:43
Let's press the return key and
right paddle.setCollideWorldBounds
1:47
right parenthesis and
end with a semicolon.
1:52
This makes sure that the paddle doesn't
go beyond the edge of the screen.
1:57
It's important to make sure you've got the
correct spelling for the helper method.
2:02
Make sure you don't have
setColliderWorldBound or
2:06
setCollideWorldsBound or
anything like that because any incorrect
2:10
character will mean
the helper method won't work.
2:14
Now let's test this in the browser.
2:19
As you can see,
if we hold on to the right key,
2:22
the paddle moves all the way to the right
and stops at the screen boundary.
2:24
And if you hold on to the left key, the
paddle goes all the way to the left and
2:28
stops at the screen boundary as well.
2:31
Perfect.
2:34
Now let's go back to our code and
add some movement to the ball.
2:34
To add movement to the ball,
2:38
we can follow a similar process
to what we did with the paddle.
2:40
Let's first scroll to the top of the file
where we've added our variables and
2:44
below the keys variable on line 25 we'll
write let ball and end with a semicolon.
2:50
Now let's scroll down to
the create function, and
2:57
we'll assign our ball object
to our ball variable.
3:00
Let's go to the front of line 41 and
write ball equals.
3:04
Next we'll set screen boundary collisions
for our ball, just like the paddle, so
3:09
our ball doesn't go off screen.
3:13
First let's turn our ball
into a physics object.
3:16
Just before the word add,
write the word physics followed by a dot.
3:20
Then below line 41, let's write
the code ball.setCollideWorldBounds,
3:25
followed by parentheses and a semicolon.
3:31
And beneath line 42, let's give the ball
some bounce by writing the code
3:35
ball.setBounce() and give that an argument
of 1, then end with a semicolon.
3:40
The setBounce() method will cause the ball
to bounce off the edges of the screen, and
3:47
anything else it collides with.
3:52
This method takes in one argument,
which is a number between 0 and 1.
3:54
This number defines
the elasticity of the ball,
4:00
which is the speed at which
it will bounce off something.
4:02
Setting it to 1 means the ball will
bounce off at the same speed that it hit
4:06
the object with, and setting it to 0
means the ball will not bounce at all.
4:11
If we set it to the middle, like 0.5,
4:16
this means the ball will bounce off at
half the speed it hits the object with.
4:19
You can experiment with different values
to see how this will affect the ball.
4:24
Right now, we're going to leave it at 1.
4:28
Let's test this in the browser.
4:31
We'll see that nothing is happening,
the ball just sits there.
4:34
This is because we haven't
given the ball any velocity, or
4:37
we haven't given the ball
any force to move it.
4:41
Let's go ahead and add that to our code.
4:44
Let's scroll down to our update
function and beneath all the if
4:48
statements, let's write
the code ball.setVelocityy,
4:53
open parentheses, and
give that an argument of minus 200.
4:58
This set the velocity of the ball
to minus 200 on the y axis which
5:03
means the ball will move up the screen
at a speed of 200 pixels per second.
5:08
Right now because the update
function is always running the ball
5:14
keep moving up the screen
until it hits a boundary.
5:19
We only want the ball to move
up at the start of the game,
5:23
then change direction after it hits
something like the edge of the screen.
5:27
To fix that we can create a variable to
keep track of whether the ball has been
5:32
launched or not.
5:36
Let's scroll up to our ball
variable on line 26 and
5:38
beneath that we'll write
the code letHasBallLaunched.
5:42
This is going to be a boolean
which we'll set to false.
5:46
Now let's scroll back down
to the update function and
5:50
let's find our
setBlastTyHelper on line 66.
5:54
And above that we'll write
the following code if
5:58
parentheses exclamation
mark has ball launched and
6:02
then we'll wrap line 67 in our if block.
6:07
Don't forget to change
the formatting of the code.
6:11
Okay, so right now the ball will only
move up if the has ball launched
6:14
variable is set to false.
6:19
But when the ball launches we want
to stop our custom velocity so
6:21
we need to set our new variable to true.
6:25
Below line 67 let's hit Enter and
6:28
write hasBallLaunched equals true and
finish with a semicolon.
6:31
Now let's test our game in the browser.
6:37
We'll see that the ball instantly
moves up when the game starts, and
6:39
comes down to the bottom of the screen.
6:43
This happens because of the bounce
property we've set, and
6:47
that's why we don't need to add
any gravity to the physics engine.
6:50
This is great, but we don't want
the ball to launch instantly.
6:55
We want the player to control
when the ball launches, and
6:59
also change the position of
the ball by moving the paddle.
7:02
Let's address both of
these issues in the code.
7:06
In the update function around
our if block on line 66,
7:11
let's add some code to launch the ball
only when the space base bar is pressed.
7:14
At the end of line 66, let's hit Enter and
7:20
write if parentheses keys.space.isDown and
7:23
we'll add curly braces and
wrap our code around it.
7:27
Of course,
don't forget to set the formatting.
7:32
Cool, now let's add some code to
move the ball with the paddle.
7:35
We can also add this code in the update
function again at the end of line 66,
7:40
let's hit Enter and write ball.x =
paddle.x, then end with a semicolon.
7:46
This will set the x position of the ball
to the x position of the paddle just
7:54
before the ball is launched.
7:59
And after the ball has been launched,
8:02
it will no longer be set
to the paddles x position.
8:04
While we're here, let's change the speed
of the ball to make it a bit faster.
8:08
Let's scroll up to line 27 and
below our ballHasLaunched variable,
8:14
let's hit Enter a few times and write
const and set our keyboard to uppercase or
8:19
hold the shift key then write
ball underscore speed equals 400.
8:26
Using uppercase characters is
a convention for global constants.
8:31
Now, let's double click on ball underscore
speed to select the text, press
8:36
command C to copy the text and scroll down
to our update function, where we've set
8:40
our ball y velocity and let's change
minus 200 to minus ball underscore speed.
8:46
We can do this by pressing command V
to paste the text that we've copied.
8:51
Cool, you can increase or decrease
the ball speed based on your preference.
8:56
Now let's test all these
changes in the browser.
9:01
So now when the game starts,
the ball doesn't move up immediately.
9:05
I can move the paddle left or
right and the ball will move with it.
9:09
When I press the spacebar,
the ball launches and
9:15
starts to bounce off the top and
bottom of the screen.
9:17
This is better, but right now the ball is
just going to keep hitting the top and
9:21
bottom of the screen.
9:25
This isn't really a fun game to play.
9:27
Let's change this in the next video by
adding some collision to the ball and
9:30
the paddle.
9:34
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up