Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player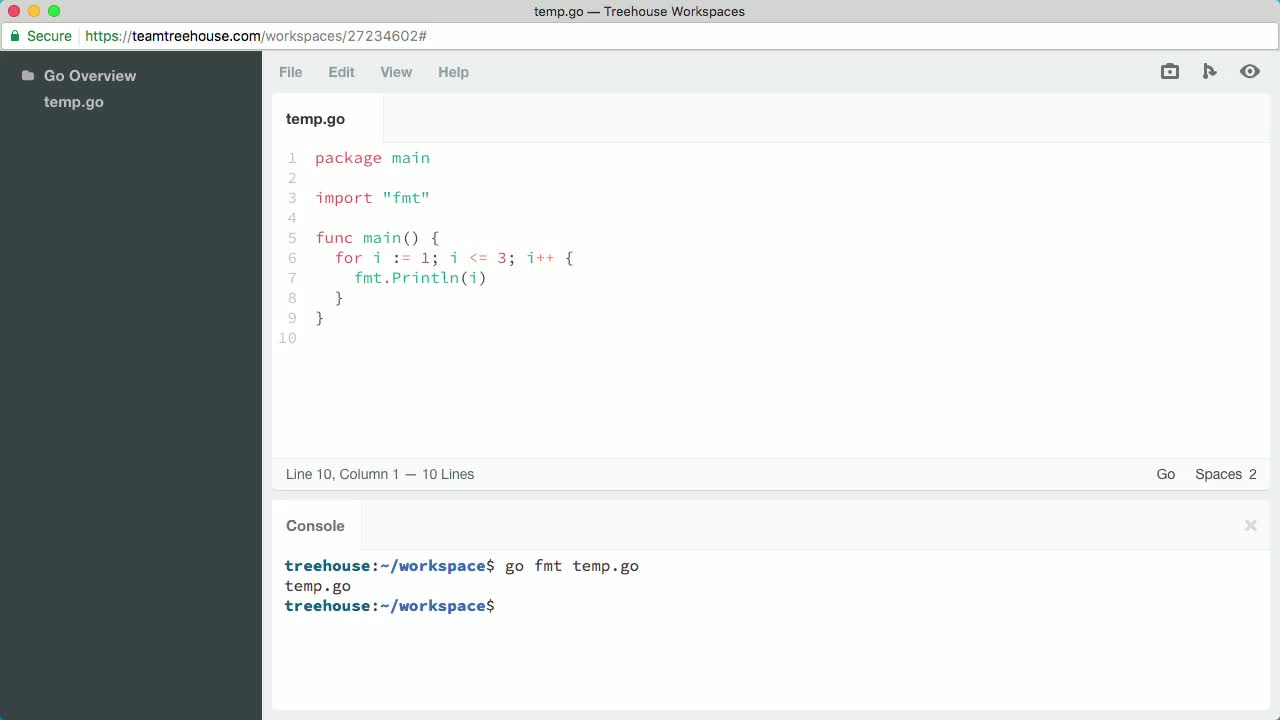
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
This stage is all about control structures; program structures that control which code runs next. First up, we'll look at loops, which cause a block of code to execute over and over.
- The
for
keyword is used for all loops in Go. - The most basic type of
for
loop looks similar to what you see in other C-like languages:- init statement
- condition
- post statement
- Followed by block
- Block code executed on each pass through loop
package main
import "fmt"
func main() {
for i := 1; i <= 3; i++ {
fmt.Println(i)
}
}
- Post statement can be whatever you want
-
i++
,i--
(change init/condition accordingly) -
i += 1
,i += 2
- But don't fail to increment it! If we used
i += 0
, for example, we'd have an infinite loop and would have to press Ctrl-C to interrupt the program.
-
Block scope rules apply to for
loops:
package main
import "fmt"
func main() {
beforeLoop := 888
for i := 1; i <= 5; i++ {
inLoop := 999
fmt.Println(i)
}
fmt.Println(beforeLoop) // OK
fmt.Println(i) // Error!
fmt.Println(inLoop) // Error!
}
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
This stage is all about
control structures,
0:00
which are program structures that
control which code runs next.
0:02
First up, well look at loops which cause
a block of code to execute over and over.
0:06
The for key word is used for
all loops and go.
0:11
The most basic type of for
0:14
loop looks similar to what you
see in other C like languages.
0:15
There's an init statement,
which is usually use to set up a variable.
0:18
A condition statement,
0:23
which causes the code to continue
looping until the condition is false.
0:24
And a post statement, which runs
after each iteration of the loop.
0:29
This is followed by a block
of code within curly braces.
0:33
The code within the block gets executed
on each pass through the loop.
0:37
So let's try running this.
0:40
And you can see that
the loop runs three times.
0:45
On the first pass, i gets set for 1.
0:48
The variable i gets set
to the value 1 initially.
0:50
Then it runs a test on i.
0:54
If i is less than or equal to the value 3,
then the loop will go ahead and
0:55
execute at this time.
1:00
Which it is.
1:02
So it goes ahead and
runs and it runs the format
1:03
print line call here and
passes the value of the i variable to it.
1:08
So it prints out the value 1.
1:12
Then, following the loop,
1:14
the post statement runs which
increments the value in i.
1:16
That's this i++ here that you see.
1:19
So, i is 1 and
i++ sets the i variable to 2.
1:23
Next pass through the loop, it tests
whether the I variable is less than or
1:29
equal to 3, and
since it's currently set to 2, it is.
1:33
So, the loop runs again, it prints out
the value 2, which you see down here.
1:36
The post statement runs again,
incrementing i from 2 up to 3.
1:40
Then it runs the test one more time.
1:45
Is i less than or equal to 3?
1:46
Well it's set to 3 currently,
so yes that's true.
1:48
It runs one more time,
it prints out the value 3.
1:52
The post statement runs,
increments i from 3 up to 4.
1:53
And it runs this test
again which does not pass,
1:58
because i is currently set to 4 which
is not less than or equal to 3.
2:01
And so the loop stops running and
our program completes.
2:05
The post statement can
be whatever you want.
2:08
For example, if we had wanted
to start our loop at three, and
2:10
gone down toward 0 we could test whether
i is currently greater than 0 and
2:14
we could change our post
statement to be i--.
2:20
That subtracts one from the value on
the i variable it's time it's evaluated.
2:23
So let's save this, try running it.
2:28
And there we go, our variable starts at 3,
2:29
gets decremented down toc 2,
gets decremented down to one1,
2:32
at which point it is no longer greater
than 0 and our loop stops running.
2:37
We could also change our loop back
to the way it originally was, and
2:43
increment by steps other than 1.
2:48
Well first let me show you
2:50
an equivalent to i++ we can
use the plus equals operator,
2:53
which takes whatever value is currently
in the variable and adds onto it.
2:57
So if I is currently set to 2,
for example,
3:02
i += 1 would assign the value 3 to i.
3:06
So let's try saving this, running it.
3:09
And you can see,
that as before it goes 1, 2, 3.
3:13
But we can skip by steps other than one,
using the += operator, as well.
3:17
Let's change our condition
to continue running as long
3:22
as i is less than or equal to 5,
and we'll update i by 2 each time.
3:26
Save that, try running it.
3:32
Now our variable will skip one
on each pass through the loop.
3:35
But whatever you do, don't fail to
increment your loop counter variable.
3:39
If we had used i + 0, for example,
3:43
which would set i back to the same
value every time it runs.
3:46
Then if we were to try running this
we'll get an infinite loop, and
3:51
we'll have to press Ctrl+C to
cancel out of our program.
3:54
Now as I mention this code here
in the curly braces is a block.
3:58
And that means that the rules
regarding variable scope apply.
4:01
If we define the variable before the loop
then its value is accessible within
4:05
the loop and it'll be accessible
after the loop as well.
4:09
But if we define the very
bold within the loop block,
4:13
then it will not be accessible
after the loop is over.
4:16
The same holds true for variables that
you declare in the initialization.
4:20
These are considered to be part
of the block scope for the loop.
4:26
So the i variable is accessible within
the loop block but not afterwards.
4:31
So this line here is okay because it
accesses a variable that was declared
4:39
before the loop even began, but
this line is not because the i variable
4:42
was declared within the loop
initialization statement.
4:47
And this line is also not okay,
because the in loop variable
4:51
was declared within the loop block,
so we get an error for those lines.
4:56
You can see the compile errors
down here in our console.
5:00
So that's our brief
introduction to the for loop.
5:03
It causes a block of code to execute
repeatedly while a condition is true.
5:06
But often we need to run a block of code
just once when a condition is true, and
5:10
not at all when a condition is false.
5:14
For that, we have if statements.
5:16
We'll look at those next.
5:18
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up