Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial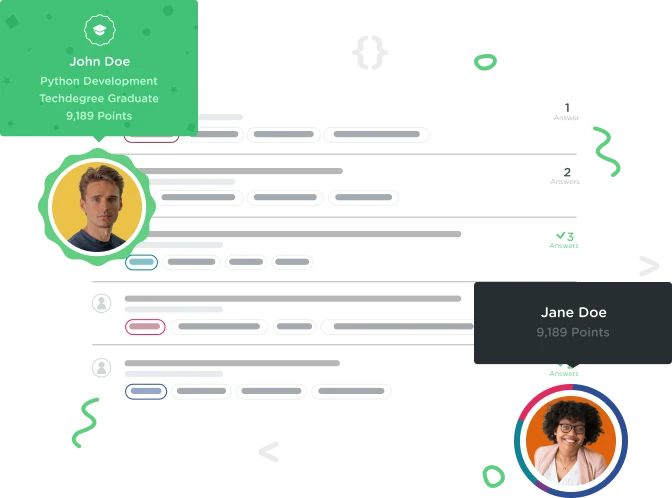
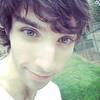
Alphonse Cuccurullo
2,513 PointsA bit confused on classes. attr reader and writer?
"""""""""""""So just a few questions to get me on the right path with classes. The attr_reader and writers. I have noticed that its a way to rewrite over certain functions in a class but in this video it is used in a different way. Like the accessor holds vaiables or symbols i'm guessing but there being used in a way similar to how we use instance variables in a initialize method. So just wondering whats going on there."""""""""
""""""""""Also i have noticed certain videos that have classes without a initialize method. Can someone give me a point is to why this initialize method is necessary and in what situations? Is it only to have a constructor? if so that is the constructor of importance?""""""""""
1 Answer
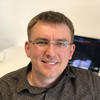
Ilya Dolgirev
35,375 PointsHi! Regarding classes - all your custom classes inherit from Object class if you don't define your own inheritance instead. You can check it in IRB:
class Person
end
Person.superclass # => Object
Therefore, if you didn't define your custom initializer, Ruby will lookup to superclass `initialize' method (Object in this case) and will invoke that one - it just creates a blank instance without any instance variables. But If you need to initialize some variables when creating instance of your class you have to define your own initializer.
About attributes methods - it just a handy way(aka 'syntax sugar') to defining methods for getting or setting the values of instance variables. These classes below are identical but look at amount of code we need to write in second case.
# USING SYNTAX SUGAR
class Person
attr_reader :name
attr_accessor :hobbies
def initialize(name)
@name = name
end
end
# NOT USING SYNTAX SUGAR
class Person
def initialize(name)
@name = name
end
# This is 'attr_reader' for 'name' instance variable under the hood
def name
@name
end
# And this is 'attr_accessor' for 'hobbies' instance variable under the hood
def hobbies
@hobbies
end
def hobbies=(value)
@hobbies = value
end
end