Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial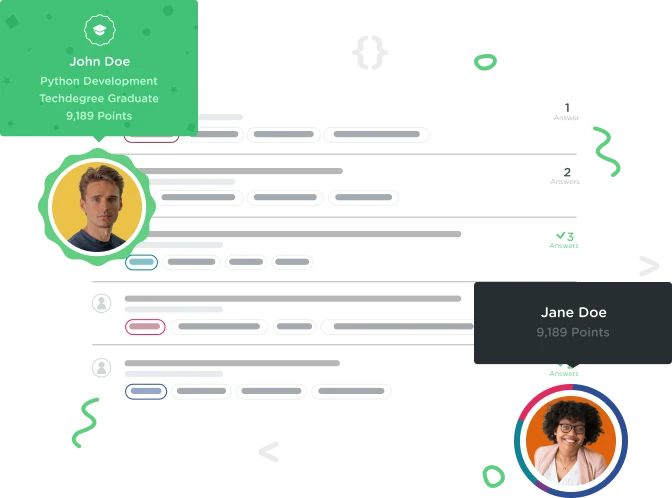
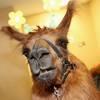
A X
12,842 PointsAdvantages/Disadvantages of Using Const vs. Var in an Array
Just finished watching the video where Andrew uses const to create variables in an array vs. the var keyword. I found the video confusing as I couldn't quite follow the const keyword's behavior, and the advantages/disadvantages of using const with an array vs. var. It seems to me since arrays frequently change that you should never or rarely use const, but that wasn't the impression I was left with at the end of this video. Can someone elaborate? Thanks!
4 Answers

Seth Kroger
56,413 PointsArrays are what's called a "reference variable" in that they refer to, or point to a value in memory instead of directly holding the value. This distinction doesn't often make much difference but here it does. With const
the effect is only one level deep, so can't change which array it points to, but you are allowed to alter the array.
const array1 = [1,2,3,4,5] // This creates the array in memory and array1 points to it.
const array2 = array1 // This doesn't copy the array, only the reference pointing to the array.
// can change the underlying array
array2.pop()
// <- 5
array1
// <- Array [ 1, 2, 3, 4 ] The 5 is gone because array1 and array2 are the same array
//But you can't change which array it points to:
array1 = [6,7,8,9]
// <- TypeError: invalid assignment to const `array1'
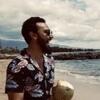
Ali Raza
13,633 PointsCONST vs VAR
Think of Const and Var as a placeholder, now the difference between them is Var is changeable e.g. If var name had a string "Ali" it can later be changed to "Raza" which is why it is called a "variable"; whereas Const stays constant so if you create a const name APR = 24.1 percent, it will stay the same throughout. That way you can't mess it up with something else when it was not intended. It is just to stay on the safer side.
JS doesn't have strict type variables but in some other languages you cannot change the type of a variable for example a string type variable will always hold a string value. You can check the MDN Reference Guide for more info.
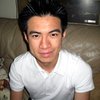
jason chan
31,009 Pointsconst
const
means the variable can't be changed // so your code will error out on the compiler if it changes later in the program
let
let
is another way to define the variable but it can mutate (change)
const carTires = 4;
let engineHp = 5, accelerate = true;
if(accelerate) {
engineHp * 5 // 25
}
carTires = 5; // this will error
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/const
var is es5 is to define a variable. Javascript is trying to be more classically typed language. Typed language is like java where you define all your datatypes.
We also have es2015 (new version of javascript) class here taught by Guil. So you can have stronger understanding of these new features in javascript.
https://teamtreehouse.com/library/introducing-es2015
If you take the java course here at teamtreehouse you will see what classically typed language is like.
https://teamtreehouse.com/tracks/java-web-development
I hope that answers your question. You'll understand once your get little more progress in your coding career.

Prabal Dutta
304 Pointsywang04 What kind of situation we need to use const keyword with the array or object literal and what the advantage of using const in real projects?
The straight answer is you don't use const in case of arrays and objects. CONST works on variables but not very useful in case of arrays and objects.
ywang04
6,762 Pointsywang04
6,762 Points@Seth Kroger We understand what you mentioned above. Actually running the following code with var keyword, it still get the same result.
Only difference is const array1 cannot be reassigned while var array1 can be reassigned.
I have the same question like nekilof. What kind of situation we need to use const keyword with the array or object literal and what the advantage of using const in real projects? Thanks in advance. :)