Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial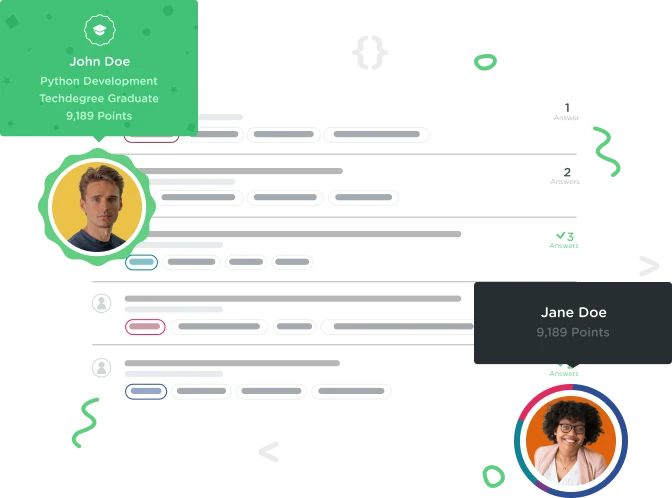
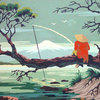
nick weber
Front End Web Development Techdegree Student 29,676 Pointsannoyed and sleepy...
no idea what is responsible for this code not passing.
from flask import Blueprint
from flask.ext.restful import Resource, Api, reqparse, inputs
import models
class IngredientList(Resource):
def __init__(self):
self.reqparse = reqparse.RequestParser()
self.reqparse.add_argument('name', required=True, help='no ...', location=['form', 'json'])
self.reqparse.add_argument('description', required=True, help='no ...', location=['form', 'json'])
self.reqparse.add_argument('measurement_type', required=True, help='no', location=['form', 'json'])
self.reqparse.add_argument('quantity', required=True, help='no ...', location=['form', 'json'], type=inputs.float)
self.reqparse.add_argument('recipe', required=True, help='no ...', location=['form', 'json'], type=inputs.positive)
super().__init__()
def get(self):
return 'IngredientList'
class Ingredient(Resource):
def get(self, id):
return 'Ingredient'
ingredients_api = Blueprint('resources.ingredients', __name__)
api = Api(ingredients_api)
api.add_resource(IngredientList, '/api/v1/ingredients')
api.add_resource(Ingredient, '/api/v1/ingredients/<int:id>')
7 Answers
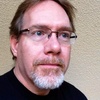
Chris Freeman
Treehouse Moderator 68,441 PointsYou are very close. I had the same error on my first attempt. The arguments to the type
keyword can be built-in types. Change type=inputs.float
to type=float
and your code will pass.
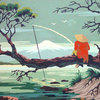
nick weber
Front End Web Development Techdegree Student 29,676 Pointsfixed it.
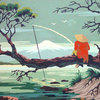
nick weber
Front End Web Development Techdegree Student 29,676 PointsThank you, that worked.
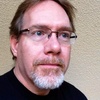
Chris Freeman
Treehouse Moderator 68,441 PointsYou gave yourself Best Answer?
Chris Grazioli
31,225 PointsKenneth Love Where is the documentation for Inputs? the http://flask-restful.readthedocs.io/en/0.3.5/api.html?highlight=inputs#inputs that was listed in the teachers notes yields a blank page with links to nothing. Even tried looking back a version or two.. still nothing
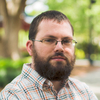
Kenneth Love
Treehouse Guest TeacherNot sure. They mention it here even.
You could check out the inputs themselves. I hate code-as-documentation, but I don't currently have anything better to offer. :(
Chris Grazioli
31,225 PointsHaving some trouble getting this to pass
from flask import Blueprint
from flask.ext.restful import Resource, Api, reqparse, inputs
import models
class IngredientList(Resource):
def __init__(self):
self.reqparse= reqparse.RequestParser()
self.reqparse.add_argument('name', required=True, help='no name argument given', location=['form', 'json'])
self.reqparse.add_argument('description', required=True, help='no description argument given',location=['form', 'json'])
self.reqparse.add_argument('measurement_type', required=True, help='no measurement type given', location=['form', 'json'])
self.reqparse.add_argument('quantity', required=True, help='no qty argument given', location=['form', 'json'], type=float)
self.reqparse.add_argument('recipe', required=True, help='no recipe argument given.', location=['form', 'json'], type=positive)
super().__init__()
def get(self):
return 'IngredientList'
class Ingredient(Resource):
def get(self, id):
return 'Ingredient'
ingredients_api = Blueprint('resources.ingredients', __name__)
api = Api(ingredients_api)
api.add_resource(IngredientList, '/api/v1/ingredients')
api.add_resource(Ingredient, '/api/v1/ingredients/<int:id>')
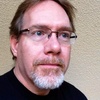
Chris Freeman
Treehouse Moderator 68,441 PointsYou have too much of a good thing: Remove the input.
prefix from float
but keep the input.
prefix for positive
. As in my comment above, "float" is a built-in type
and "positive" is not, but it is available as input.positive
.
With this change your code passes the challenge.
Chris Grazioli
31,225 Points@Chris Freeman too much of a good thing? Would you be referring to being explicit vs implicit and not abstracting things to death...? I just tried changing the "input." around and its still throwing the error: Bummer! "description" should be in the arguments, required, and located in form or json; "measurement_type" should be in the arguments, required, and located in form or json; "name" should be in the arguments, required, and located in form or json; "quantity" should be in the arguments, of type float, required, and located in form or json; "recipe" should be in the arguments, of type positive, required, and located in form or json
from flask import Blueprint
from flask.ext.restful import Resource, Api, reqparse, inputs
import models
class IngredientList(Resource):
def __init__(self):
self.reqparse= reqparse.RequestParser()
self.reqparse.add_argument('name', required=True, help='no name argument given', location=['form', 'json'])
self.reqparse.add_argument('description', required=True, help='no description argument given',location=['form', 'json'])
self.reqparse.add_argument('measurement_type', required=True, help='no measurement type given', location=['form', 'json'])
self.reqparse.add_argument('quantity', required=True, help='no qty argument given', location=['form', 'json'], type=float)
self.reqparse.add_argument('recipe', required=True, help='no recipe argument given.', location=['form', 'json'], type=input.positive)
super().__init__()
def get(self):
return 'IngredientList'
class Ingredient(Resource):
def get(self, id):
return 'Ingredient'
ingredients_api = Blueprint('resources.ingredients', __name__)
api = Api(ingredients_api)
api.add_resource(IngredientList, '/api/v1/ingredients')
api.add_resource(Ingredient, '/api/v1/ingredients/<int:id>')
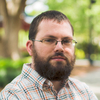
Kenneth Love
Treehouse Guest Teacherinput
!= inputs
(you imported the latter)
Chris Grazioli
31,225 PointsKenneth Love , always getting jammed up on a technicality. Is there a flask module that uses regex or a library of common mispelling, transposition errors, pluralities ,etc , to autocorrect code???
apparently I'm constantly in need
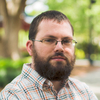
Kenneth Love
Treehouse Guest TeacherHa! A library to detect typos would be an amazing thing. This is where IDEs really come in handy. They'll highlight places where you create a variable and don't use it, or use something without defining/importing it, etc. Unfortunately, we can't put that full functionality into code challenges.
Chris Grazioli
31,225 PointsChris Grazioli
31,225 Points@Chris Freeman Do you have any idea as to why the code with the type=inputs.float wouldn't pass when thats the way Kenneth Love showed us in the video. I'm trying to look at the docs listed in the teachers notes for Inputs but the link is lacking any content. thanx
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsLooking at the imported
inputs.py
[source], the added types ininputs
are for non built-in types: url, boolean, positive, natural, date, and a few others. Built-in types, such as, int, float, str,... are specified directly as the argument without using the "inputs.
" prefix.I gathered this info through inspection. Not sure why the docs provide no API info. ?