Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial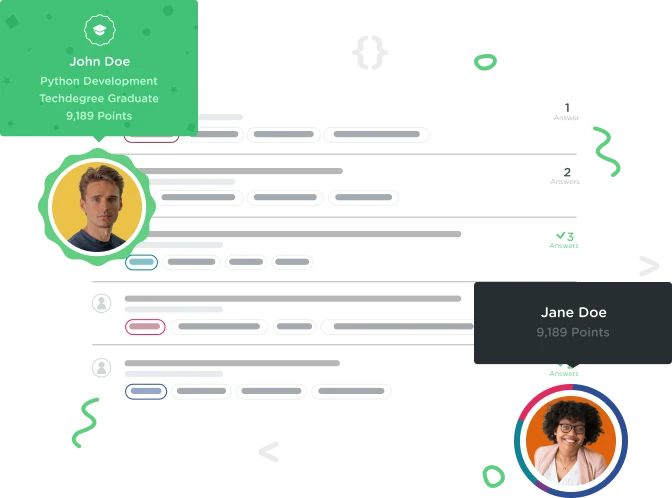

Shaun Glassman
6,496 PointsAnother 'Uncaught TypeError: Cannot read property 'querySelector' of undefined'
I'm not sure why I've been getting this error. Chrome developer tools are telling me it's on line 62 (which is the first time I use the query selector on taskListItem.
Any help would be much appreciated :)
Here is the code:
//Problem: User interaction doesn't provide desired results. //Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new task var addButton = document.getElementsByTagName("button")[0]; //first button var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks var completedTasksHolder = document.getElementById("completed-tasks"); // completed-tasks
//Add a new task var addTask = function() { console.log("Add task..."); //When the button is pressed //Create a new list item with the text from #new-task: //input (checkbox) //label //input (text) //button.edit //button.delete //Each elements, needs modified and appended }
//Edit an existing task var editTask = function() { console.log("Add task..."); //When the Edit button is pressed //if the class of the parent is .editMode //Switch from .editMode //label text become the input's value //else //switch to edit mode //input value becomes the label's text
//toggle .editMode }
//Delete an existing task var deleteTask = function() { console.log("Delete task..."); //When the delete is pressed //remove the parent list item from the ul
}
//Mark a task as complete var taskCompleted = function() { console.log("Task complete"); //When the checkbox is checked //Append the task list item to the #completed-tasks }
//Mark a task as incomplete var taskIncomplete = function() { console.log("Task incomplete..."); //When the checkbox is unchecked //Append the task list item to the #incomplete-tasks }
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) { console.log("Bind list item events"); //select taskListTItem's children //NOTE -- RIGHT BELOW IS WHERE THE ERROR IS, BUT IT LOOKS RIGHT ... var checkBox = taskListItem.querySelector("input[type=checkbox]"); var editButton = taskListItem.querySelector("button.edit"); var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to the delete button
deleteButton.onclick = deleteTask;
//bind the checkBoxEventHandler to the checkbox
checkBox.onchange = checkBoxEventHandler; }
//Set the click handler to the addTask function addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items for (var i = 0; i < incompleteTasksHolder.children.length; i++) { //bind events to list item's children (taskCompleted) bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted); }
//cycle over completeTaskHolder ul list items for (var i = 0; i < incompleteTasksHolder.children.length; i++) { //bind events to list item's children (taskCompleted) bindTaskEvents(completedTasksHolder.children[i], taskIncomplete); }
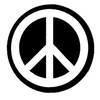
john larson
16,594 PointsDid you ever resolve this?
1 Answer
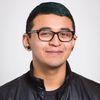
Ben Flores
17,074 PointsSomeone else answered it here: https://teamtreehouse.com/community/console-error-referenceerror-tasklistitem-is-not-defined
But basically, your loops at the bottom are currently both using incompleteTasksHolder.children.length, but you want to cycle over both incomplete AND completed tasks.
To fix it, you should change your second loop so that it uses completedTasksHolder.children.length and you should be good to go.
Harjot Kaur
7,277 PointsHarjot Kaur
7,277 PointsI have the same issue. Please tell me if you could fix it.