Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial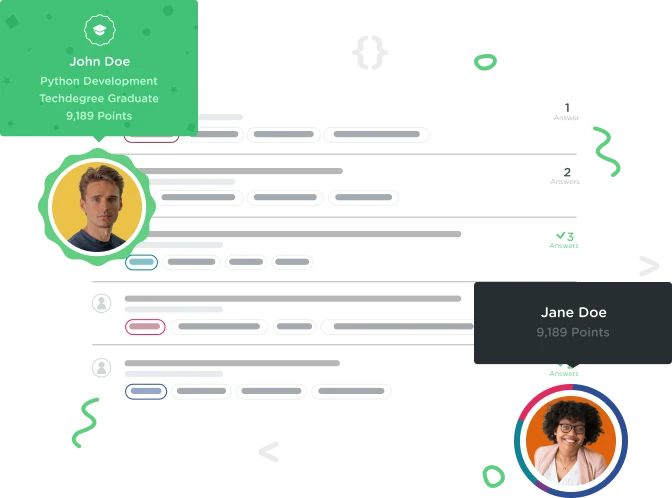

Matthew Lurie
3,512 Pointsattempting to solve this using switch. What am I doing wrong?
just watched the video and...switch was not addressed as a possible solution. Also all the "return" statements not addressed. Also no explanation of the function that was pre-loaded...am I the only one who felt the video solution did not address the parameters of the challenge?
Any help into my coding errors would be appreciated.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case (n % 3 == 0) && (n % 5 == 0): return "FizzBuzz"
case (n % 3 == 0): return "Fizz"
case (n % 5 == 0): return "Buzz"
// End code
return "\(n)"
}
2 Answers
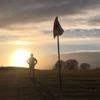
Stuart Wright
41,118 PointsI think the intended solution for this challenge is to use if/elseif/else. That would certainly be much easier than using a switch statement. That said, getting this to work with a switch statement was a fun challenge - here are the changes I made to get it to work:
- Added a curly brace at the end of the switch statement. This was missing in your code above.
- This is the most important one: the value that you are switching on (n) is of type int, but your case statements evaluate to type boolean. This causes a compile error. The type of the value you are switching on must match the type of the cases you define. It seems we need boolean cases here, so you should switch on a boolean. Perhaps surprisingly, simply entering true works.
- Switch statements must be exhaustive, so you need to add the default case to the switch statement.
Here's my solution:
func fizzBuzz(n: Int) -> String {
switch true {
case (n % 3 == 0) && (n % 5 == 0): return "FizzBuzz"
case (n % 3 == 0): return "Fizz"
case (n % 5 == 0): return "Buzz"
default: return "\(n)"
}
}
An alternative solution I came across by searching the forums is:
func fizzBuzz(n: Int) -> String {
switch(n % 3 == 0, n % 5 == 0) {
case(true, false):
return "Fizz"
case(false, true):
return "Buzz"
case(true, true):
return "FizzBuzz"
default:
return "\(n)"
}
}

Matthew Lurie
3,512 Pointsthanks. This challenge was especially confusing because it came right after Pasan had just said that we almost always want to use switch instead of if statements. In addition, the video solution didn't actually reflect a lot of what we had just learned. For example, the "for in" statement in the video is NOT in the solution. And replacing print with return is not in the video. I see from the forums that I'm not the only one experiencing this. Just seems like there's a sloppy level of quality between what the challenges ask and what is being taught. Doesn't help anyone when one explicitly doesn't follow the other.