Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial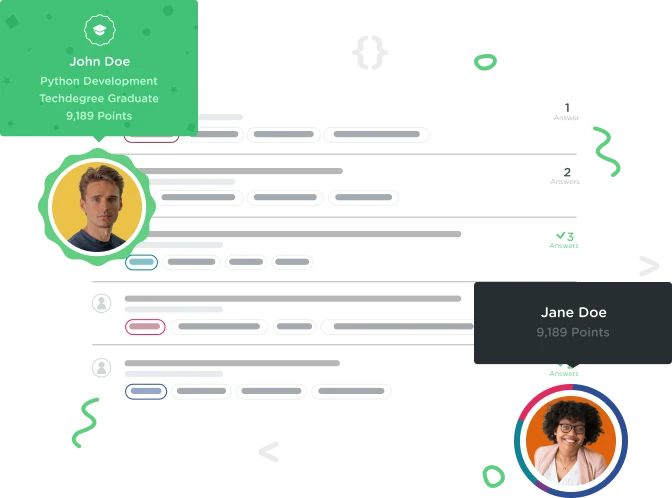

Liana Moleni
2,644 Pointsbummer indentation error
i have tried and tried a million times but it keeps saying indentation error, and if it is inline with the attributes it gives an error saying class meta is not an attribute of challenge. what should i do.
from peewee import *
db = SqliteDatabase('challenges.db')
class Challenge:
name = CharField(max_length=100)
language = CharField(max_length=100)
class Meta:
database = db
2 Answers
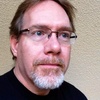
Chris Freeman
Treehouse Moderator 68,457 PointsLiana Moleni, you are correct in that, for your code, Meta
is not an attribute of Challenge
. This is because Challenge
has no inherited classes, so is type object
. The key missing part is inheriting from peewee.Model
which provides the Meta
attribute:
from peewee import *
db = SqliteDatabase('challenges.db')
class Challenge(Model): #<- added Model inheritance
name = CharField(max_length=100)
language = CharField(max_length=100)
class Meta: #<-- corrected indentation
database = db
So Kenneth Love's challenge is correct as written.
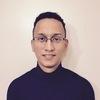
Daniel Santos
34,969 PointsThis worked for me, but I believe there is an error in this code challenge Kenneth Love.
from peewee import *
db = SqliteDatabase('challenges.db')
class Challenge:
name = CharField(max_length=100)
language = CharField(max_length=100)
class _meta:
database = db
If you see the class nested is '_meta', but in the instructions and the video it was 'Meta'.
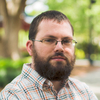
Kenneth Love
Treehouse Guest TeacherIt's not a bug, AFAICT, but just how Peewee exposes the Meta
class for a model. Both Meta
and _meta
are allowed.
But, as per Python's conventions, you don't want to rely on methods/attributes/properties/etc that start with an underscore. That generally implies that the thing isn't guaranteed to work the exact same way forever. The Meta
object, though, without having the underscore, is expected to be predictable from now on.