Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial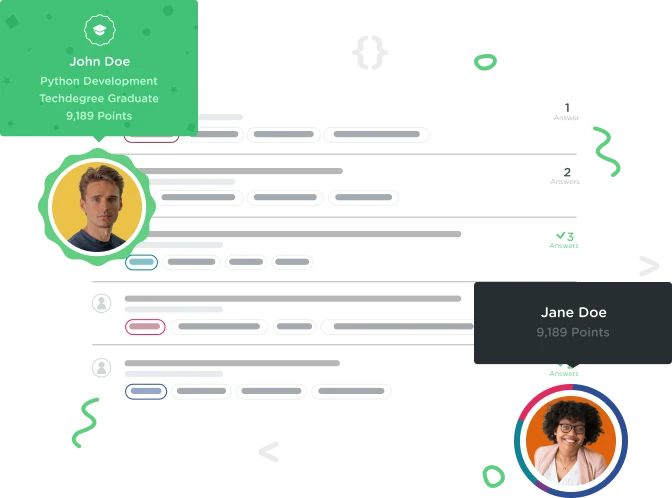

qfmftjlpgl
11,971 PointsCallback hell?
Not a question, but a bit of a feedback and it is related to the video: https://teamtreehouse.com/library/default-values-in-mongoose
Why do we need to save the data by typing it into our code? There are callbacks inside the callback. I doubt that we would ever use something like that on the real project.
The rest of the course is simply great, thanks.
3 Answers
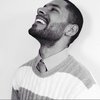
Michael Liendo
15,326 PointsYou're one step ahead of the game :) Later on in the lecture he addresses that very question by showing ways to get around that. He simply demonstrated that as means of working in the sandbox.

qfmftjlpgl
11,971 PointsThanks for the input, Michael. The course is really great, though as Andrew tries to explore all the possibilities, it gets confusing as he rewrites the code to optimize it, which is great, however not ideal for a novice like me.

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsThis isn't directed at the original poster, but anytime you disagree with the style of the instructor, you can always rewrite it yourself (in this case using global.Promise or Bluebird).
Here is my code using native ES6 promises (the remove
, save
, and find
methods return a promise) and ES7 async/await. Any errors are sent to the catch block and logged to the console.
Note: You need a minimum of Node v7.6.0, which uses V8 5.5.
const mongoose = require('mongoose');
mongoose.Promise = global.Promise;
mongoose.connect('mongodb://localhost:27017/treehouse');
const db = mongoose.connection;
db.on('error', (err) => { console.log('Connection Error: ', err); });
// Once the connection opens, the callback will be called
db.once('open', () => {
console.log('Database connection successful!');
const Schema = mongoose.Schema;
const AnimalSchema = new Schema({
type: { type: String, default: 'goldfish' },
size: { type: String, default: 'small' },
color: { type: String, default: 'gold' },
mass: { type: Number, default: 0.01 },
name: { type: String, default: 'Angela' }
});
const Animal = mongoose.model('Animal', AnimalSchema);
const elephant = new Animal({
type: 'elephant',
size: 'big',
color: 'gray',
mass: 6000,
name: 'Lawrence'
});
// Color supplied by defaults
const whale = new Animal({
type: 'whale',
size: 'big',
mass: 190000,
name: 'Fig'
});
// All values supplied by defaults
const animal = new Animal({});
(async function () {
try {
// Remove all documents
console.log('Deleting existing documents...');
await Animal.remove({});
// Save all animals
console.log('Saving new documents...');
await Promise.all([elephant.save(), whale.save(), animal.save()]);
// Log each animal with a size of 'big'
console.log('\n' + 'Big animals:');
const animals = await Animal.find({ size: 'big' });
animals.forEach((item) => { console.log(item); });
// Close the database connection
db.close();
console.log('\n' + 'Database connection closed.');
// Catch errors here
} catch (err) {
console.log(err);
db.close();
}
}());
});
UPDATE: After moving ahead in the course and spending a day playing around with Mongoose, I've decided that using features like arrow functions and promises do not play nicely with Mongoose. Some things work, some things don't. These issues might get resolved when Mongoose 5 is released, but until then, there are more modern libraries like camo and mongorito that work much better if you want to use the latest JavaScript features.
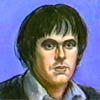
Jesse Thompson
10,684 PointsNice code you have here. Yeah it seems Andrew was just trying to write the ugliest code possible to just see how bad it could get. This looks nice though how you did this. At the same time I swear there are synchronous alternatives to most async commands so while learning about promises is of course helpful, learning anything is helpful, in a lot of cases i assume the option to use synchronous commands are still there.
Promises seem useful though because it still does several commands at the same time.