Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial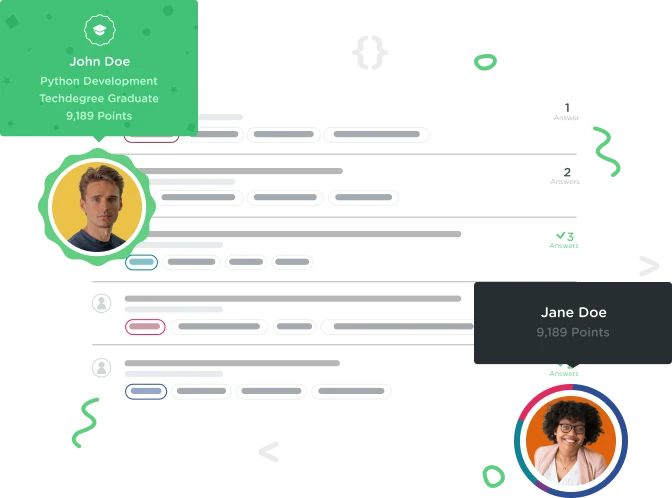
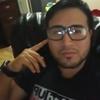
Frank Campos
4,175 PointsCan somebody help me to use the attrs library that kenneth mentioned?
I did my own version of class Die with the library attrs. I dont know if the code is pythonic but it replaced all the magic method used in this lecture.
import random
import attr
@attr.s
class Die:
sides = attr.ib()
value = attr.ib()
def selection(self, sides=2,value=0):
# self.sides=sides
if not sides >= 2:
raise ValueError("Must have at least 2 sides")
if not isinstance(sides, int):
raise ValueError('side must be a whole number')
self.value = value or random.randint(1, sides)
return int(self.value)
dice =Die(2,0)
print(dice.selection(9) +2)
print(dice.selection == 100)
#and so on
Kenneth Love can you help me with this?porfavor, and also if we can create the class D6 will be very awesome, too. I have been trying to figure out how to make it works, but I broke my code.
4 Answers
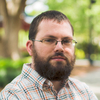
Kenneth Love
Treehouse Guest TeacherThis worked for me. Didn't test everything but made sure the values and sides were set correctly.
import random
import attr
@attr.s
class Die:
sides = attr.ib(
default=2,
validator=attr.validators.instance_of(int)
)
value = attr.ib(validator=attr.validators.instance_of(int))
@sides.validator
def check_sides(self, attribute, value):
if not value >= 2:
raise ValueError("Must have at least two sides")
@value.default
def random_value(self):
return random.randint(1, self.sides)
@value.validator
def check_value(self, attribute, value):
if value and not 0 < value <= self.sides:
raise ValueError("Value must be in range(0, sides)")
D6 = attr.make_class("D6", {"sides": 6}, bases=(Die,))
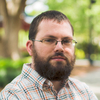
Kenneth Love
Treehouse Guest TeacherWhat is it doing/not doing that you're not expecting?
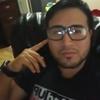
Frank Campos
4,175 PointsI tried to recreate the classes Die and D6, exactly what you did in the videoComparing and Combining Dice, but I could never figure out how to inherent the class Die to the D6 class. My goal is to do exactly what you did in the video with the help of the library attrs. I don't know if I used the library attrs properly. The Die class is working, it can add, compare and so on. I would love to see how you will resolve this with the attrs library. Gracias Kenneth Love
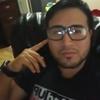
Frank Campos
4,175 PointsThank you, Kenneth Love , you are amazing.