Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial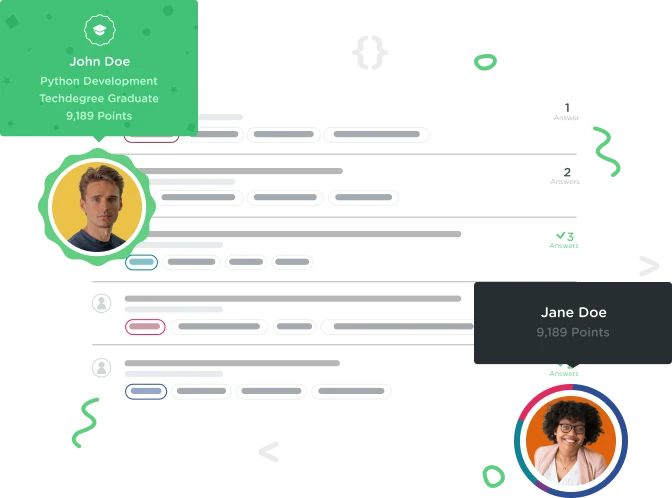
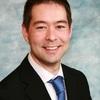
Mark Chesney
11,747 Pointscannot resolve "KeyError on the key "'name'". Did you unpack the dictionary that's passed as a parameter to favorite..."
KeyError results, but I don't see how to move forward with this.
My code: def favorite_food(name=None, food=None): return "Hi, I'm {name} and I love to eat {food}!".format(name, food)
# Complete the favorite_food function below.
# It accepts a dictionary as an argument.
# Your function should unpack that dictionary and pass it to the format method as keywords,
# then return the resulting string.
def favorite_food(name=None, food=None):
return "Hi, I'm {name} and I love to eat {food}!".format(name, food)
favorite_food(**{'name':'ME','food':'yogurt'})
4 Answers

Pete P
7,613 PointsYou do not have to create your own dictionary to unpack. Nor do you have to call the 'favorite_food' function yourself. Remember, some random dictionary named 'dict' will be passed to the function. Your job is to make sure it gets unpacked into the format method.
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(# Your code to unpack dict here)
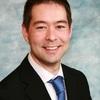
Mark Chesney
11,747 PointsSure thing, Pete. It's recorded in a separate discussion I duplicated inadvertently. And my solution is dictionary referencing, rather than unpacking, which shouldn't pass the challenge, but it does anyway!
def favorite_food(dict):
return "Hi, I'm {} and I love to eat {}!".format(dict['name'], dict['food'])
But I would've never learned the point had I not heard it from Dave, on the other discussion.

Pete P
7,613 PointsAh, I see. Thanks for sharing. I know this will be helpful to others as well!
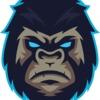
Hrithik Sharma
6,090 Pointsok but when i use this sol in VS code editor it is returning nothing but when i use print it works fine
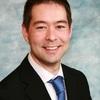
Mark Chesney
11,747 PointsHrithik, are you saying that the return command doesn't actually return in the Visual Studio code editor? That's unusual.
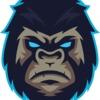
Hrithik Sharma
6,090 Pointsyes do you know why
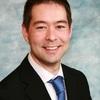
Mark Chesney
11,747 PointsI don't, and I don't use VS (sorry). Did you assign it a variable when you called it? For example:
mickey = {'name':'Mickey Mouse','food':'cheese'}
mickey_intro = favorite_food(mickey)
By the way, I hope you're using this code below (and NOT the dict['name'], dict['food'] code above):
def favorite_food(kwargs):
return "Hi, I'm {} and I love to eat {}!".format(**kwargs)
Mark Chesney
11,747 PointsMark Chesney
11,747 PointsThank you Pete. I resolved this, with more time and with your help. I had an incomplete understanding of packing and unpacking -- my incorrect understanding was that packing and unpacking always involves asterisks (** for dict and * for tuples), but I now know the assignment was asking for unpacking to be done without **. The difficult thing to grasp for me was that the use of "dict[name]" and "dict[food]" is considered unpacking -- I just thought of it as retrieving the value of a dict by passing the dict key through favorite_food(dict). So if that's incorrect, I welcome anyone's input. Alright, again, thank you!
Pete P
7,613 PointsPete P
7,613 PointsGlad I could help! But, I'm actually curious to see your answer now because I used ** in my solution.