Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial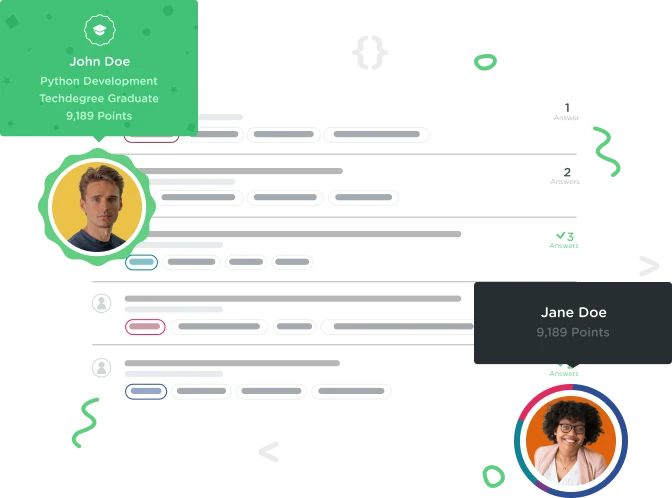
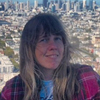
Cissie Scurlock
11,072 PointsCan't beat the Timezone Strings challenge in Python
I feel like I'm close to getting this but with this code I get the error: 'to_timezone` didn't return the right, converted datetime.' I've tried including a fmt = '%Y-%m-%d %H:%M:%S' as a variable while returning new_datetime.strf(fmt) and and that gives me an error saying that I didn't return a datetime. Any clues?
import datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(eastern):
eastern = pytz.timezone('US/Eastern')
new_datetime = starter.astimezone(eastern)
return new_datetime
4 Answers
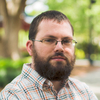
Kenneth Love
Treehouse Guest TeacherThis challenge has nothing to do with strftime()
. It's only about creating a pytz
timezone object and use .astimezone()
to turn starter
into that new timezone. Your original code is 100% correct with one mistake :) Let's look at it.
def to_timezone(eastern):
eastern = pytz.timezone('US/Eastern')
new_datetime = starter.astimezone(eastern)
return new_datetime
OK, your to_timezone()
function takes an argument, just like it's supposed to. You're calling it eastern
but we don't know that it'll be the US/Eastern timezone. Let's rename it to tz
, for timezone, yeah?
def to_timezone(tz):
eastern = pytz.timezone('US/Eastern')
new_datetime = starter.astimezone(eastern)
return new_datetime
Now, right inside the function, you're making a pytz.timezone
object, just like you should, but you're always making it be 'US/Eastern'
. Where did you get that from? We need to make it be whatever timezone came in as tz
. So, since tz
is a timezone name, just like 'US/Eastern'
is, how would you change that line to use the name that's in tz
instead of 'US/Eastern'
?
The rest of your code is correct.
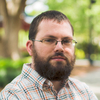
Kenneth Love
Treehouse Guest Teacherto_timezone
is supposed to take a string that's a timezone's name and convert starter
to that timezone. You've hardcoded US/Eastern
...
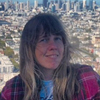
Cissie Scurlock
11,072 PointsHmmm. I'm still at a loss. I don't understand how to convert starter to a new timezone without hardcoding it.
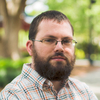
Kenneth Love
Treehouse Guest TeacherCissie Scurlock What are you doing on the first line inside of your to_timezone()
function? You're creating a new timezone object which you then use to convert the datetime
. Just don't hardcode the timezone it's set to, use the one that gets passed to the function.
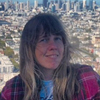
Cissie Scurlock
11,072 PointsThanks for the hints but I still can't quite grasp what I should be doing:
def to_timezone(eastern):
fmt = '%Y-%m-%d %H:%M:%S %Z%z'
new_datetime = starter.astimezone(eastern)
return new_datetime.strf(fmt)

james white
78,399 PointsCissie,
I definitely feel your pain!
This was a very difficult Python course to get through
(even though I took Python basics and several other courses first)
Luckily I knew enough (in general) about "def"s
(which are how Python defines functions)
to come up with:
import datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(tz):
eastern = pytz.timezone(tz)
new_datetime = starter.astimezone(eastern)
return new_datetime
This code still doesn't seem right, because you are supposed to be able to use any timezone for tz and not have it internally use 'eastern' but it passed for me <shrug>
I would recommend this Python DateTime course as the last Python course to take
(even after the new Python Regular Expressions course).
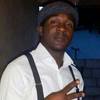
MUZ140305 Kudakwashe Siziva
5,600 Pointsthis worked for me
def to_timezone(tz): Zim = pytz.timezone(tz) new_time = starter.astimezone(Zim) return new_time
Cissie Scurlock
11,072 PointsCissie Scurlock
11,072 PointsAha. Got it. Thanks Kenneth Love!