Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial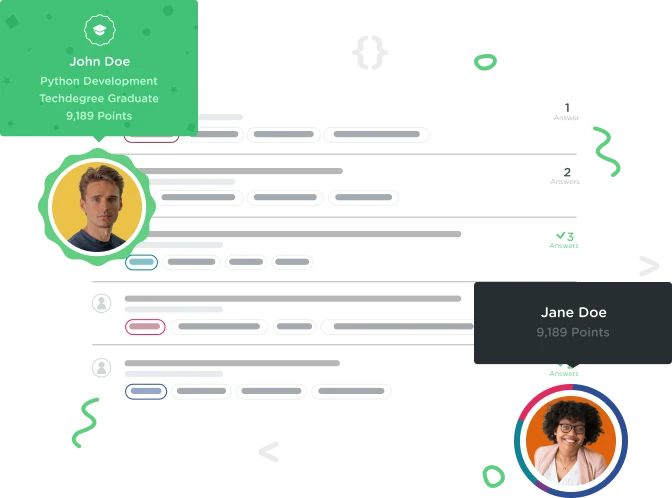

Mark Douglass
4,093 PointsCan't work out why my 'disemvowel' function won't work.
I ran this in the workspace, with various strings, and it seemed to work fine.
I can't work out why, according to the error, this still returns vowels.
Any feedback would be greatly appreciated.
def disemvowel(word):
#print(word)
letter_list = []
vowel_list = ["a", "e", "i", "o", "u"]
# Converting 'word' string to a list.
for letter in word:
letter_list.append(letter.lower())
#print(letter_list)
# Iterating through vowel list.
for vowel in vowel_list:
# Checking if the vowel still exists in letter list.
while vowel in letter_list:
try:
letter_list.remove(vowel)
except ValueError:
pass
#print(letter_list)
# Converting list back to string.
word = ''.join(letter_list)
#print(word)
return word
4 Answers
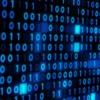
Alexander Davison
65,469 PointsJust note you can actually iterate over strings.
That means this code is valid:
def disemvowel(word):
result = ''
for letter in word:
if letter not in 'aeiou':
result += letter
return result
If you want to get super fancy, you can use list comprehensions. Don'y worry; you learn them in a future course/workshop (if you want to check 'em out now, click me to go to a wonderful workshop all about List Comprehensions):
def disemvowel(word):
return ''.join([x for x in word if x not in 'aeiou'])

Mark Douglass
4,093 PointsThank you very much Alex. Much appreciated.
I look forward to getting to the List Comprehensions. I have played with them a teeny weeny bit before, and love them, but don't want to jump ahead, yet. I don't want my brain to explode with all of this awesome Python.
Thanks for taking the time to reply. Much appreciated.

Mark Douglass
4,093 PointsOne little note: as you didn't see the notes to the challenge, you may not have noticed the request for it to take UPPERCASE and lowercase vowels, so I simply had to add .lower() to the if statement.
Thanks again for your clean solution.
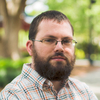
Kenneth Love
Treehouse Guest TeacherThe challenge says to remove the vowels, upper and lower. It doesn't say to change the case of the word. Your code is wrong, not the grader. Same for the two samples in the "best answer". Gotta follow the instructions, y'all. :)

Mark Douglass
4,093 PointsThanks, Kenneth. Adding the .lower() to the if statement makes the code run, and run fine. It passed the challenge, and is completing the task.
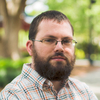
Kenneth Love
Treehouse Guest TeacherYeah, you can lower for the comparison...Or just compare against both cases, which is more explicit and doesn't require any extra method calls.

Mark Douglass
4,093 PointsThanks again Kenneth.
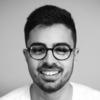
João Elvas
5,400 PointsYou also need to lower it like, using the fancy way:
def disemvowel(word):
return ''.join([x for x in word if x.lower() not in 'aeiou'])

Mark Douglass
4,093 PointsThanks Joao. I like how concise your version is.
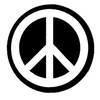
john larson
16,594 PointsHey Mark, Alex gave a concise way to solve this challenge. I went through your whole code several times. It works local. It does not pass the challenge, but you know that. This challenge has been somewhat glitchy. Maybe it still is.

Mark Douglass
4,093 PointsThank you for trying, John. I agree, it works locally, but something is going wrong with the challenge.
I enjoyed the response from Alex, as it reminded me I don't need to convert from strong to list, and back again.
Thanks for making the time to help out. Much appreciated.
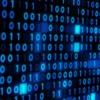
Alexander Davison
65,469 PointsI'm tagging Kenneth Love
john larson
16,594 Pointsjohn larson
16,594 PointsI just ran Alexes code, and it did not pass the challenge. I think the challenge is having issues.