Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial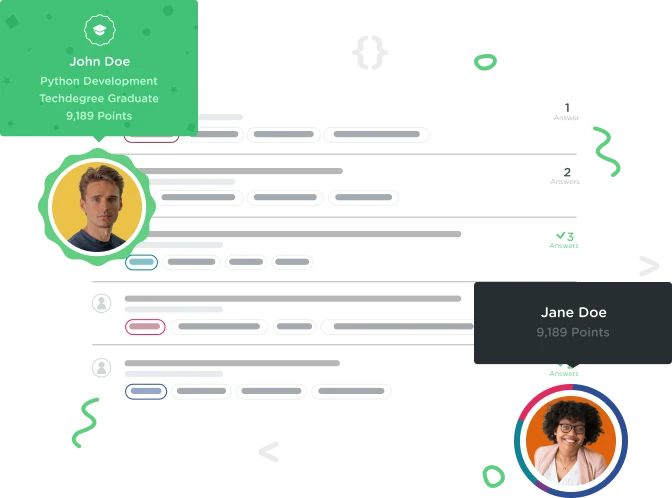
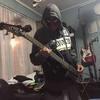
Rodrigo Carvajal
Courses Plus Student 12,374 PointsClear screen for pycharm as if it were on console or cmd
In the python videos, the teacher uses a function to clear the screen which works great when you then run the script in a terminal or cmd. However, I'm developing a game in pycharm and it obviously doesn't work. I cam across this same issue in NetBeans when programming in java and came up with a very very nasty solution (that I found online and modified):
static void clearScreen() throws IOException, InterruptedException, AWTException {
String path = System.getProperty("user.dir").toLowerCase();
// new ProcessBuilder("cmd", "/c", "cls").inheritIO().start().waitFor();
if (path.contains("documents") ||
path.contains("documentos") ||
path.contains("google")) {
Robot pressbot = new Robot();
pressbot.keyPress(17); // Holds CTRL key.
pressbot.keyPress(76); // Holds L key.
pressbot.keyRelease(17); // Releases CTRL key.
pressbot.keyRelease(76); // Releases L key.
TimeUnit.SECONDS.sleep(1);
} else if (path.contains("c:")) {
new ProcessBuilder("cmd", "/c", "cls").inheritIO().start().waitFor();
}
}
Would there be an easier way to do this in Python? I really don't want to resort to it working only in certain directories and simulating key strokes.
Most development nowadays ends up happening in IDEs, so it would be important to 'maybe' cover this stuff in a separate course?
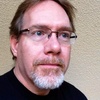
Chris Freeman
Treehouse Moderator 68,423 PointsI am unaware of a programmatic way to clear the pycharm screen.
2 Answers
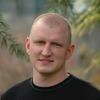
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsI could not find any clear way to clear the PyCharm screen. A simple way to make it look like you cleared the PyCharm screen though is to print a bunch of line breaks. Like 80 of them. Even though you can still scroll up and see your last output, you will have what looks like a clear screen. This would be less than ideal for console app though.
Faking Clear Screen (for PyCharm)
print('\n'*80) # prints 80 line breaks
Clearing Screen for Command/Terminal
import os
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
Otherwise you have to go through that hacky way of emulating Key presses for a Ctrl+L key press strictly for PyCharm.

Allison Schaaf
33,322 PointsYou can create your own keyboard shortcuts in PyCharm:
- Open Pycharm preferences
- Search: "clear all"
- Double click "clear all" -> add keyboard shortcut (set it to CTRL + L or whatever you'd like)
- Make sure to click in the console (or Run window) before using the shortcut or it doesn't do anything.
Rodrigo Carvajal
Courses Plus Student 12,374 PointsRodrigo Carvajal
Courses Plus Student 12,374 PointsKenneth Love any thoughts?