Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial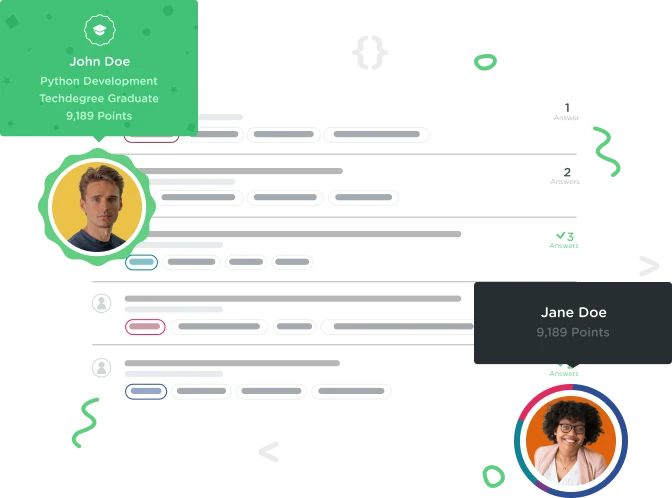

Atul Varma
4,285 PointsCode for finding if String contains only letters and $ character..??
private String normalizeDiscountCode(String discountCode){
if( discountCode != "[a-zA-z]" || char!= '$'){
throw new IllegalArgumentException("Invalid Discount Code");
}
1 Answer
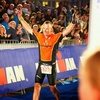
Steve Hunter
57,712 PointsHi Atul,
You need to break the parameter that is passed in into a char array and iterate over each char in turn. There, test to see if the character is a letter (Use the class method isLetter()
). Also test if the character is a dollar sign. It is better to test whether the character is not a letter and not a dollar sign. Use the not operator, !
.
So, set up a for
loop and break the discountCode
(or whatever you called your parameter) down to an array using toCharArray()
. Then do the test, !Character.isLetter(c)
where c
is your loop local variable &&
also c != '$'
. If that evaluates to true
, throw the IllegalArgumentException
, else return the upper case discountCode
and exit the method. That all looks like:
private String normalizeDiscountCode(String code){
for(char c : code.toCharArray()){
if(!Character.isLetter(c) && c != '$'){
throw new IllegalArgumentException("Invalid discount code.");
}
}
return code.toUpperCase();
}
This post Goes into a little more detail of this, should you need it.
Steve.