Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial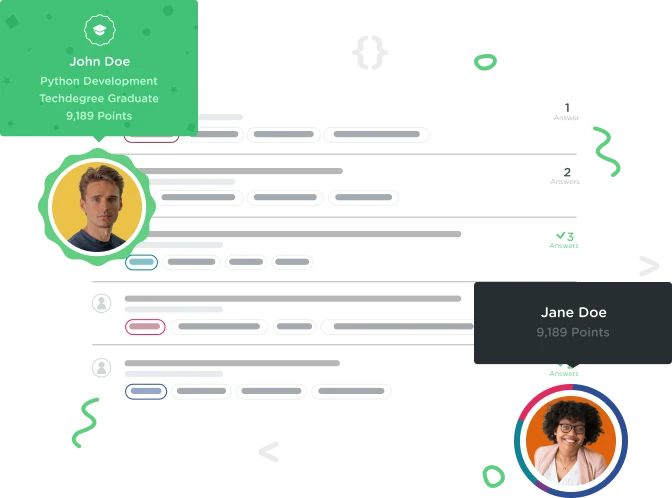

Ullas Savkoor
6,028 PointsCode for Letter game with file import and variable length strike with with explanation
Ok so for the extra credit for letter_game we were told to use open and read() method. *The crucial piece of information was to have "one word per line". *So using open we get the file object and then using read() method we get the entire content of the file containing list of fruits. *The next step is to use the split() method using '\n'(new line separator) on the string received from using read() method. *I have use len(secret_word) to get variable length of strikes Note: I have used global variable fruits since it would be better to initialize it once. Kenneth Love : request your inputs for my code given below.
import random
import os
import sys
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(bad_guesses, good_guesses, secret_word):
clear()
print("Strikes: {}/{}".format(len(bad_guesses),len(secret_word)))
print('')
for letter in bad_guesses:
print(letter, end = ' ')
print("\n\n")
for char in secret_word:
if char in good_guesses:
print(char,end='')
continue
print('_',end='')
print()
def get_guess(bad_guesses, good_guesses):
while True:
char = input("Guess the character of the word ")
char = char.lower()
if len(char) != 1:
print("You can only guess one character at a time")
elif char in bad_guesses or char in good_guesses:
print("You have already guessed the character")
elif not char.isalpha():
print("You can guess only alphabets")
else:
return char
def play(done):
clear()
secret_word = random.choice(fruits)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print("You win!")
print("The secret word was {} ".format(secret_word))
done = True
else:
bad_guesses.append(guess)
if len(bad_guesses) == len(secret_word):
draw(bad_guesses, good_guesses, secret_word)
print("You lose!")
print("The secret word was {} ".format(secret_word))
done = True
if done == True:
play_again = input("Press Y/n to play again ").lower()
if play_again != 'n':
play(done=False)
else:
sys.exit()
def welcome():
action = input("Press enter/return to continue or Q to quit ").lower()
if action == 'q':
sys.exit()
set_fruits()
def set_fruits():
try:
file = open('letter_game_input.txt')
except IOError:
print("Unable to form list of fruits, exiting the game")
sys.exit()
else:
global fruits
fruits = file.read().split('\n')
file.close()
fruits = []
clear()
welcome()
play(done=False)
2 Answers
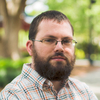
Kenneth Love
Treehouse Guest TeacherYou shouldn't need to global
your fruits
variable. Since fruits
is a list (which is mutable) and it's already defined at the outermost scope, it should be accessible and modifiable from anywhere in your script. This has plusses and minuses, but I think it's OK in this scenario.
To save yourself from having to do the split, look up readlines
.

Ted Holmes
Courses Plus Student 713 PointsExperiencing same issue as Ullas regarding global and readlines
Ullas Savkoor
6,028 PointsUllas Savkoor
6,028 PointsKenneth Love : When I didn't use keyword global in my set_fruits() , the fruits variable was treated as a local variable in the function causing the fruits variable in the outermost scope to not be intialized. I am not sure but when I used readlines the newline character was also appended to the fruit literals in the list.For ex: string literal 'apple' would be 'apple\n' , and when I used len() function it would include the newline character. I am not sure how to get the list of string literals without getting the newline character when I use readlines().
My input file contains one string literal(each representing a fruit) per line.