Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial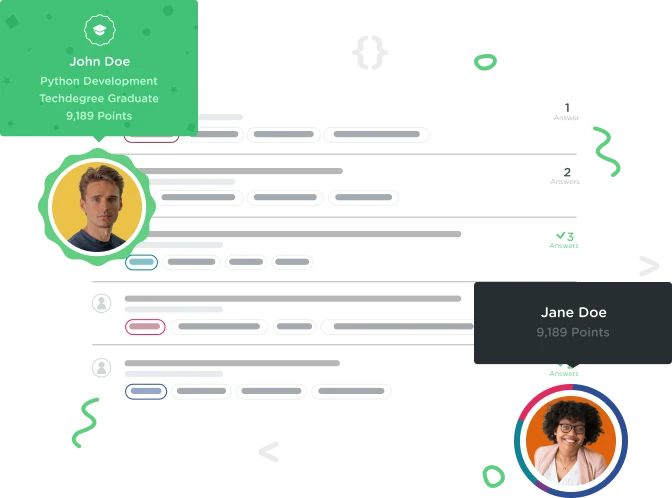

Micheal Jones
3,972 PointsCombine and Manipulate strings
I dont know what im doing wrong here>
let firstName="Micheal";
let lastName="Jones";
let role = 'developer';
var msg="firstName "+"lastName "+"role.";
1 Answer
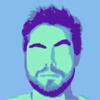
Cameron Childres
11,820 PointsHi Micheal,
When you surround a value in quotes you're signifying that it's a string. Right now you've set your msg variable equal to the string "firstName lastName role.", when you want it to read "Micheal Jones: developer".
Remove the quotes and you can access the variables. Here's an example:
let firstName = "Micheal";
let lastName = "Jones";
let role = "developer";
var msg = firstName + " " + lastName;
// msg is now "Micheal Jones"
Notice that I've added a space in quotes between the two variables. If I didn't, msg would read "MichealJones".
You can also use backticks to make a template literal instead:
let firstName = "Micheal";
let lastName = "Jones";
let role = "developer";
var msg = `${firstName} ${lastName}`;
// msg is now "Micheal Jones"
With this in mind, take another look at the challenge prompt. It wants you to make a string that looks exactly like "Micheal Jones: developer". Whichever method you go with you need to make sure that you match the spacing and punctuation exactly.
Micheal Jones
3,972 PointsMicheal Jones
3,972 PointsI dont know if I fully understand this.
let firstName = "Micheal"; let lastName = "Jones"; let role = "developer"; var msg = firstName + " " + lastName;
What would go inside the parentheses? wouldn't role go inside of them? role would also be placed at the end of my last name right. I wrote it down the way you have have it here and its still coming up wrong.
Cameron Childres
11,820 PointsCameron Childres
11,820 PointsI only gave you a partial answer to demonstrate how you can construct the real answer by yourself. You still need to add a colon, a space, and the variable
role
to the end.About what goes inside the quotes here:
var msg = firstName + " " + lastName;
Anything inside the quotes is considered a string. In this case I have a single space inside the quotes. We set the variables to strings in the lines above, so when they are accessed this line adds 3 strings together:
role
should not be placed inside quotes when setting yourmsg
variable because then it would literally read "role" and wouldn't be replaced by the string you set, "developer".You're correct that it should be added after the
lastName
variable. You'll also want to put a colon and space in quotes betweenlastname
androle
so that you match the punctuation that the challenge is looking for.It may be worth reviewing these videos to go over the concepts of variables, strings, and concatenation:
I hope this helps! Let me know if you get stuck.