Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial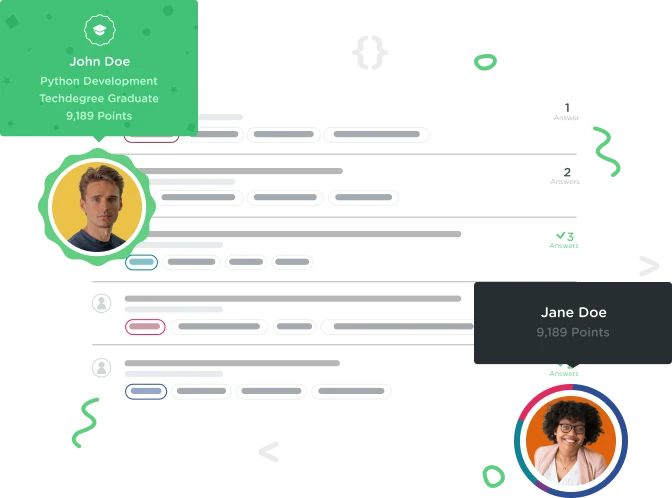

benjamin siverly
3,430 PointsConfused with how tests work in Python - see results
I'm not exactly sure how / why this is giving me trouble - since expected and got are the same, shouldn't that be a success?
treehouse:~/workspace/dungeon$ python -m doctest dd_game.py
**********************************************************************
File "/home/treehouse/workspace/dungeon/dd_game.py", line 54, in dd_game.get_moves
Failed example:
get_moves((0, 2))
Expected:
['RIGHT', 'UP', 'DOWN']
Got:
['RIGHT', 'UP', 'DOWN']
**********************************************************************
1 items had failures:
1 of 2 in dd_game.get_moves
***Test Failed*** 1 failures.

benjamin siverly
3,430 Points"""dungeon game : explore a dungeon to find a hidden door and escape. Be careful, a dragon is hiding somewhere in the dark."""
import os
import random
GAME_DIMENSIONS = (5, 5)
player = {'location': None, 'path': []}
def clear():
"""Clear the screen"""
os.system('cls' if os.name == 'nt' else 'clear')
def build_cells(width, height):
"""create and return width by height grid of two tuples
>>> cells = build_cells(2, 2)
>>> len(cells)
4
"""
cells = []
for y in range(height):
for x in range(width):
cells.append((x, y))
return cells
def get_locations(cells):
""" randomly pick starting locations for monster, door, player
>>> cells = build_cells(2,2)
>>> m, d, p = get_locations(cells)
>>> m != d and d!= p and m != p
True
>>> d in cells
True
"""
monster = random.choice(cells)
door = random.choice(cells)
player = random.choice(cells)
if monster == door or monster == player or door == player:
monster, door, player = get_locations(cells)
return monster, door, player
def get_moves(player):
"""based on tuple of player position, return list of acceptable moves.
>>> GAME_DIMENSIONS = (2, 2)
>>> get_moves((0, 2))
['RIGHT', 'UP', 'DOWN']
"""
x, y = player
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if x == 0:
moves.remove('LEFT')
if x == GAME_DIMENSIONS[0] - 1:
moves.remove('RIGHT')
if y == 0:
moves.remove('UP')
if y == GAME_DIMENSIONS[1] - 1:
moves.remove('DOWN')
return moves
def move_player(player, move):
x, y = player['location']
player['path'].append((x, y))
if move == 'LEFT':
x -= 1
elif move == 'UP':
y -= 1
elif move == 'RIGHT':
x += 1
elif move == 'DOWN':
y += 1
return x, y
def draw_map():
print(' _'*GAME_DIMENSIONS[0])
row_end = GAME_DIMENSIONS[0]
tile = '|{}'
for index, cell in enumerate(cells):
if index % row_end < row_end - 1:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
def play():
cells = build_cells(*GAME_DIMENSIONS)
monster, door, player['location'] = get_locations(cells)
while True:
clear()
print("WELCOME TO THE DUNGEON!")
moves = get_moves(player['location'])
draw_map(cells)
print("\nYou're currently in room {}".format(player['location']))
print("\nYou can move {}".format(', '.join(moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move in ['QUIT', 'Q']:
break
if move not in moves:
print("\n** Walls are hard! Stop running into them! **\n")
continue
player['location'] = move_player(player, move)
if player['location'] == door:
print("\n** You escaped! **\n")
break
elif player['location'] == monster:
print("\n** You got eaten! **\n")
break
if __name__ == '__main__':
play()
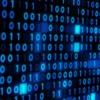
Alexander Davison
65,469 PointsTagging Kenneth Love and Chris Freeman
3 Answers
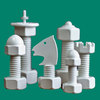
Steven Parker
229,744 Points
There's a space after the closing bracket on line 55.
Apparently extra white space on the line throws off the comparison.

benjamin siverly
3,430 PointsThanks!
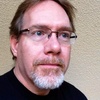
Chris Freeman
Treehouse Moderator 68,423 PointsBeyond getting the tests to pass there is a syntax error:
WELCOME TO THE DUNGEON!
Traceback (most recent call last):
File "<string>", line 141, in <module>
File "<string>", line 115, in play
TypeError: draw_map() takes 0 positional arguments but 1 was given
? Fix by adding parameter:
def draw_map(cells):
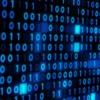
Alexander Davison
65,469 PointsNice catch Chris :)

benjamin siverly
3,430 PointsWay to go! That was very helpful!

benjamin siverly
3,430 PointsFigured it out! A space after the expected outcome threw it off but when I removed all whitespace it ran fine.
Good to know for the future though :)
Thanks!
Steven Parker
229,744 PointsSteven Parker
229,744 PointsSharing your code along with the results would be most helpful for analyzing your issue.