Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial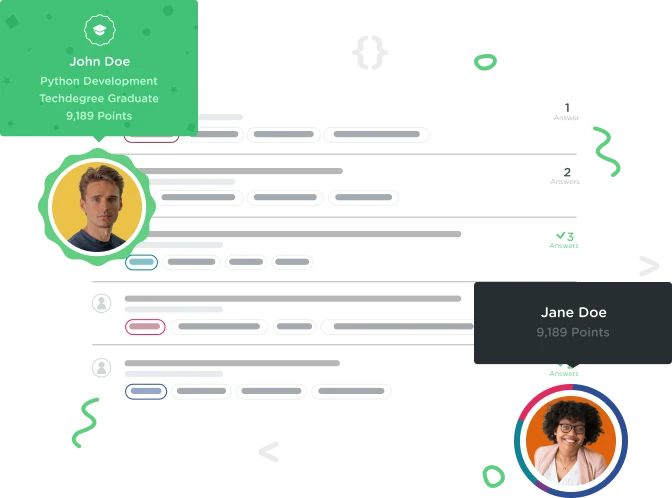

Shawndee Boyd
6,002 PointsCreate a function named search_challenges that takes two arguments, name and language.
I may be reading this wrong or over-thinking it but any help would be appreciated.
from models import Challenge
def create_challenge(name, language, steps=1):
Challenge.create(name=name,
language=language,
steps=steps)
def search_challenges(name, language):
count = 0
if name == name | language == language:
return Challenge
5 Answers
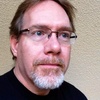
Chris Freeman
Treehouse Moderator 68,423 PointsFollowing the form used in the video, select all the Challenge
s then filter using where()
.
Edit: added parens around each condition
def search_challenges(name, language):
"""Search challenge by name and language."""
# select all the challenges
challenges = Challenge.select()
# filter by name and by language
challenges = challenges.where((Challenge.name==name) | (Challenge.language==language))
# return filtered challenges result
return challenges
Also, according to this thread, Kenneth Love, provides an alternative answer that combines both statements and uses a "comma" notation:
Challenge.select().where(
Challenge.name.contains(name),
Challenge.language==language
)

Ary de Oliveira
28,298 Pointsfrom models import Challenge
def create_challenge(name, language, steps=1): Challenge.create(name=name, language=language, steps=steps) def search_challenges(name, language): """Search challenge by name and language."""
challenges = Challenge.select()
challenges = challenges.where((Challenge.name==name) | (Challenge.language==language))
return challenges

Shawndee Boyd
6,002 PointsThe code you have provided is saying "Didn't find the right number of records".
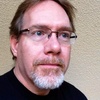
Chris Freeman
Treehouse Moderator 68,423 PointsRight you are! Sorry, pasted wrong version. I've updated the answer by adding parens around each condition.

Shawndee Boyd
6,002 PointsI should have seen that!! Thank you!!!

wads wdsf
Python Web Development Techdegree Student 1,678 Pointsdef search_challenges(name,language):
return Challenge.select().where(Challenge.name==name and Challenge.language==language)
Colby Work
20,422 PointsColby Work
20,422 PointsI got stuck on this. The way the challenge is worded threw me off ("You don't need boolean and or binary & for this, just put both conditions in your where()"). I must have missed the part in the video where "|" is mentioned. Thanks for this.