Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial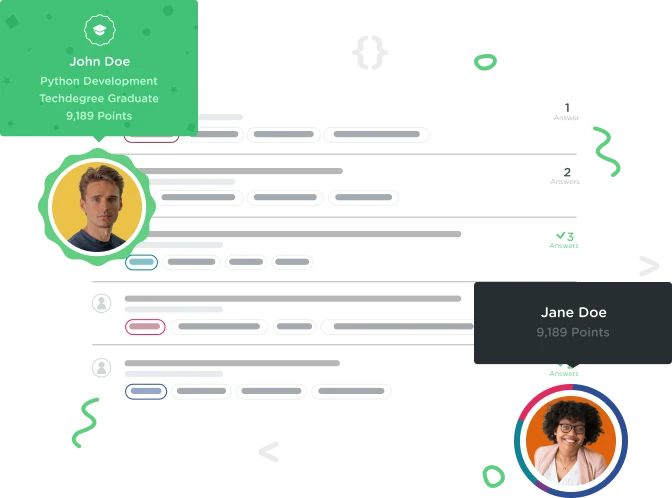
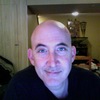
Charles Harpke
33,986 PointsCreate a function named to_timezone that takes a timezone name as a string. Convert starter...
I can pass this code in my local environment, but not the challenge.. Here is my code:
import datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(aware_dt, zonename):
tz = pytz.timezone(zonename)
return tz.normalize(aware_dt.astimezone(tz))
starter = datetime.datetime(2015, 10, 21, 23, 29, tzinfo=pytz.utc)
print(to_timezone(starter, "America/Los_Angeles"))
2015-10-21 16:29:00-07:00
import datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
8 Answers
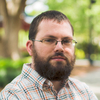
Kenneth Love
Treehouse Guest Teacherto_timezone()
only takes one argument, the name of the timezone to convert starter
to. starter
is already an UTC-aware datetime
, so you'll need to use .astimezone()
on it. The only problem is figuring out which timezone...good thing you get the name of the TZ as the argument to the function. Use pytz
's timezones and return that new datetime
.
Since starter
is defined outside of the function, you'll be able to get its value inside the function even without getting it as an argument.
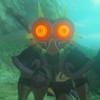
tomasvukasovic
24,022 PointsOk so here is what I found out about this Challenge:
def to_timezone(arg):
timezone = pytz.timezone(arg)
return starter.astimezone(timezone)

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 Pointsimport datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(timezone_str):
return starter.astimezone(pytz.timezone(timezone))
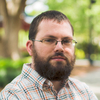
Kenneth Love
Treehouse Guest TeacherYou're still assuming you know the time zone beforehand. The tz
argument will be the time zone name. Make a pytz
time zone based in that and return starter
converted to it.
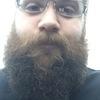
Jebadeiah Jones
10,429 PointsThis is what I needed to read to figure this out. Thanks, from almost 4 years in the future.
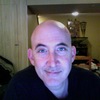
Charles Harpke
33,986 PointsArgh...sorry about that. I don't know why I was under the impression it needed to be coded....In any event...thank you!

Brendon Van der nest
12,826 Pointsimport datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(*arg):
timezone = pytz.timezone(*arg)
return starter.astimezone(timezone)
Kenneth Love Thank you sir!!! you gave me a huge clue." You're still assuming you know the time zone beforehand."
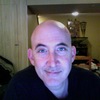
Charles Harpke
33,986 PointsThe time zone name I assign to 'tz' is more or less arbitrary?
import datetime
import pytz
fmt = "%Y-%m-%d %H:%M:%S %Z%z"
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(tz):
tz = pytz.timezone('US/Pacific')
return starter.astimezone(tz)
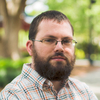
Kenneth Love
Treehouse Guest TeacherStop hard coding the timezone! :)
my_tz = pytz.timezone(tz)
Then return the starter like you're doing already.

Jen Szuster
1,940 PointsWhat am I doing wrong?
import datetime
import pytz
fmt = '%m-%d %H:%M %Z%z' starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
def to_timezone(tz): my_tz = pytz.timezone(tz) return starter.astimezone(tz)
Charles Harpke
33,986 PointsCharles Harpke
33,986 PointsI'm still trying to figure out the error of my ways on this one... 'to_timezone' has 1 argument....(tz)... I've used .astimezone on my already aware datetime in 'starter' message: 'to_timezone did not return the right, converted datetime...'