Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial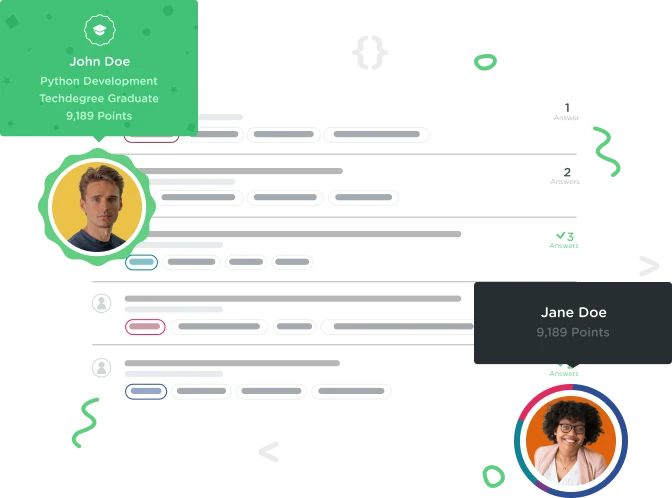

James Nelson
Courses Plus Student 3,567 PointsDate from an form into a php script to enter into a mysql database
So I am creating a form to have a date entered from a user along with some text for another field in the database. Basically it's a database that will record a date and specials for a bakery/deli. I want the user to be able to enter the current date or a future date and the special that corresponds. My question is how do I handle the date information from the form going into the database and how do I handle it to show up correctly coming out of the database later on?
1 Answer

Marc Quarles
3,455 PointsThere's a lot of ways to do this, but I think probably the easiest method would be to use a datetime field in mySQL and the PHP DateTime object:
To get the date into the database: <?PHP
// Receive data from the webpage, and get it into mysql database. $objDate = new DateTime($_POST['the_date']); // create DateTime object with the POSTed data $formatted_date = date_format($my_date, 'Y-m-d h:i:s'); //formats date into mySQL Datetime field format
// field "the_date" should be a datetime field $sql = "insert into table (the_date) values ('$formatted_date')";
mysql_query($sql, $conn); //execute the sql to create the record; could also use mysqli ?>
To retrieve the date from the database: <?PHP
//Retrieve date from database and format it for display
$sql="select the_date from table where id=1";
$result = mysql_query($sql); //query the database $row = mysql_fetch_object($result); // convert the response to an object
$the_date = new DateTime($row->the_date); Create a new DateTime object from the field returned by the sql query
//Now you can use date_format to put the date into the format you prefer: echo date_format($the_date,"l F jS, Y"); // returns something like "Monday, July 7, 2014"
?>
James Nelson
Courses Plus Student 3,567 PointsJames Nelson
Courses Plus Student 3,567 PointsThank you Marc. I read somewhere that using the PDOstatement method is a safer way to communicate with the database. I got the date to enter into the database using the date function instead of the date/time function. Now what I'm not sure of is how to pull the information from the database and display it in a specials block that I made on the homepage of the site.
Mike Baxter
4,442 PointsMike Baxter
4,442 PointsPDO doesn't add any (additional) security for communicating with the Database; it just makes it a little easier to escape and prepare your queries before you execute them, and that little bit of extra clarity in your code might help you avoid mistakes. But you can be just as safe in either of the approaches if you know what you're doing.