Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial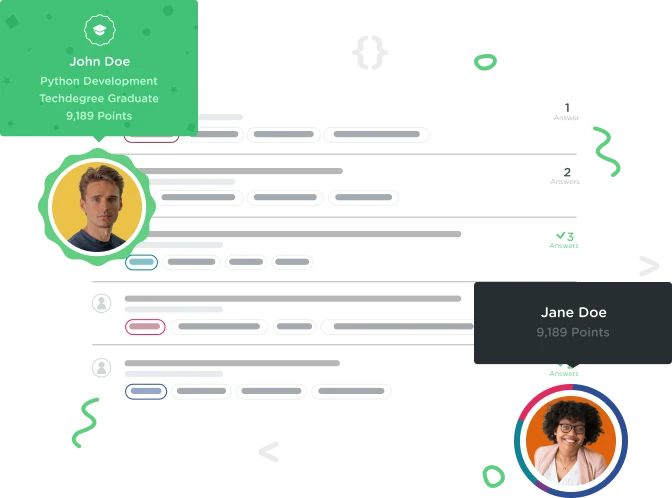

Clayton Perszyk
Treehouse Moderator 48,850 Pointsdatetime problems
This is my code for the time tango challenge and it seems like it should return a datetime object; but, it is not passing.
import datetime
def time_tango(date, time):
formatStr = "{} {}".format(date, time)
return datetime.datetime.strptime(formatStr, "%m/%d/%Y %I:%M:%S")
3 Answers
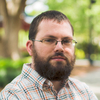
Kenneth Love
Treehouse Guest TeacherYou have a time
object and a date
object. Neither one will just turn into the string that you're expecting on their own (that's why strftime
exists), but there's an easier way that I covered in the video. A method named combine()
.
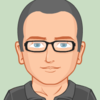
Martin Luckett
32,591 PointsThanks Kenneth. That worked great.

Clayton Perszyk
Treehouse Moderator 48,850 PointsThanks Kenneth for the link to the combine method; that definitely fixed my problem.

Hunter MacDermut
15,865 Pointsimport datetime
def time_tango(d, t):
dt = datetime.datetime.combine(d, t)
dt = dt.strftime("%m/%d/%y %H:%M")
return datetime.datetime.strptime(dt, "%m/%d/%y %H:%M")
Still struggling with this one. Tried recreating this locally with what I assumed the variables were that you were passing in:
import datetime
today = datetime.datetime.today()
date = today.date()
time = today.time()
def time_tango(d, t):
dt = datetime.datetime.combine(d, t)
dt = dt.strftime("%m/%d/%y %H:%M")
return datetime.datetime.strptime(dt, "%m/%d/%y %H:%M")
print(time_tango(date, time))
Returns:
2014-11-17 15:20:00
And I get what I think is the desired result. It would be helpful to clarify whether "date" and "time" are datetime objects or strings, and in what format the output should be. The challenge specifies the output should be a datetime, but you suggest using the combine method, which requires the "date" and "time" to be strings. Here's what I get if "date" and "time" are strings:
import datetime
date = "11/17/14"
time = "3:15:00"
def time_tango(d, t):
return datetime.datetime.strptime(d + " " + t, "%m/%d/%y %H:%M:%S")
print(time_tango(date, time))
Returns:
2014-11-17 03:15:00
Neither approach passes the challenge, so I must be overlooking something obvious. A nudge in the right direction would be greatly appreciated.

Clayton Perszyk
Treehouse Moderator 48,850 PointsI had a similar problem. Forget about strptime and strftime for this challenge. The only method you need is datetime.datetime.combine(). Kenneth provided a link to the documentation above, if you need to look into the combine() method further.
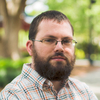
Kenneth Love
Treehouse Guest TeacherI'm gonna make the prompt a bit more obvious for this one. It's obviously not obvious (hah) enough.

Hunter MacDermut
15,865 PointsThanks Clayton for the tip and Kenneth for the clarification. Got it on the first try with the improved prompt!
Martin Luckett
32,591 PointsMartin Luckett
32,591 PointsClayton
I have the same problem (my code below)
Kenneth Love help please.