Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial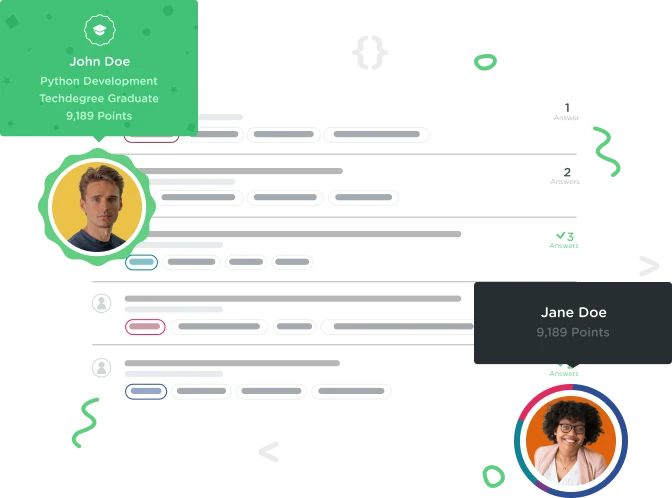
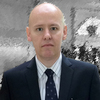
Nathan Tallack
22,160 PointsDeletes all reviews not just one.
Got something strange happening. Not sure why.
After updating my Review.delete method to use auth the delete action now removes ALL reviews, not just the one matching the ID.
@auth.login_required
def delete(self, id):
try:
review = models.Review.select().where(
(models.Review.created_by == g.user) &
(models.Review.id==id)
).get()
except models.Review.DoesNotExist:
return make_response(json.dumps(
{'error': 'That review does not exist or is not editable'}
), 403)
query = review.delete()
query.execute()
return '', 204, {'Location': url_for('resources.reviews.reviews')}
Very strange. Kenneth had his code with a comma separating the created_by and id conditions in his select. I tried using a & operator there, but still no go. Deletes every review when I call delete on the reviews route.
Hurting my brain trying to work out why.
2 Answers

Tatiana Vasilevskaya
Python Web Development Techdegree Graduate 28,600 PointsI think this is because delete() performs a delete on the entire table. To delete a single review, use delete_instance().
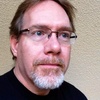
Chris Freeman
Treehouse Moderator 68,441 PointsChanged to answer.
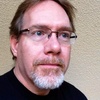
Chris Freeman
Treehouse Moderator 68,441 PointsDigging into the peewee documentation,
"Table-level operations, such as select(), update(), insert(), and delete(), are implemented as classmethods. Row-level operations such as save() and delete_instance() are implemented as instancemethods.
"
The delete() method offers the warning:
"Warning:
This method performs a delete on the entire table. To delete a single instance, see Model.delete_instance().
"
I had been thinking of the Django delete()
method which operates on table rows, not the whole table.
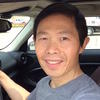
David Lin
35,864 PointsThanks to the above answers, the following works for me:
reviews.py
@auth.login_required
def delete(self, id):
try:
review = models.Review.select().where(
models.Review.created_by==g.user,
models.Review.id==id
).get()
except models.Review.DoesNotExist:
return make_response(json.dumps(
{'error': 'That review does not exist or is not editable'}
), 403)
query = review.delete_instance()
return '', 204, {'Location': url_for('resources.reviews.reviews')}
courses.py
@auth.login_required
def delete(self, id):
course = course_or_404(id)
query = course.delete_instance()
return '', 204, {'Location': url_for('resources.courses.courses')}
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsThis made my brain hurt, too! {ouch} tagging Kenneth Love for some Guru insight. (PyCon is in PDX this week, expect delayed response)