Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial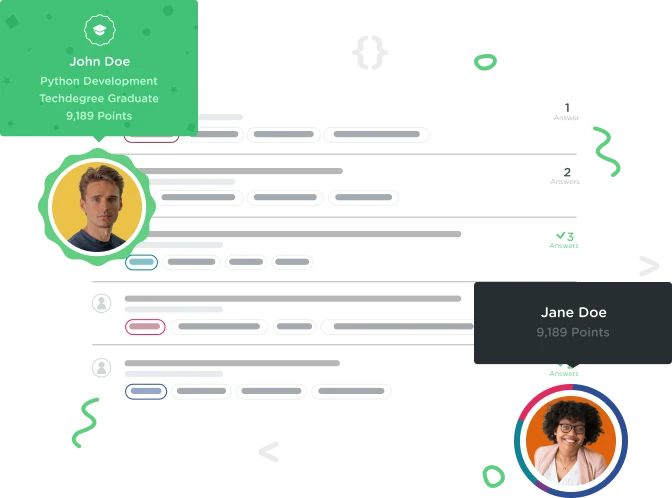
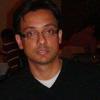
Mohammad Aslam
6,053 PointsDisemvowel Challenge
Can someone please tell me whats wrong with my code? The challenge is to remove vowels from a word. I don't think you can use the remove function on a string, so I made a list of the word first. I am not sure why the remove function is not removing vowels from my list, like it is supposed to. Would appreciate your help.
def disemvowel(word): for letter in list(word): if letter in ['a','e','i','o','u']: list(word).remove(letter) else: pass return list(word)
def disemvowel(word):
for letter in list(word):
if letter in ['a','e','i','o','u']:
list(word).remove(letter)
else:
pass
return list(word)
3 Answers
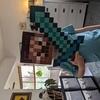
Gabbie Metheny
33,778 PointsYou're on the right track, you just need some tweaking!
Let's start with your list. Turning word
into list(word)
is great, except right now, you're generating a new list from scratch in your return
statement. If you store the list in a variable within the function, before the loop, your loop has access to it, and it will update each time through your loop.
def disemvowel(word):
word_list = list(word)
for letter in word_list:
# do something
return word_list
Next, you need to check for both upper and lowercase vowels. The easiest way to do this is to ask if letter.lower()
is in the list.
if letter.lower() in ['a', 'e', 'i', 'o', 'u']:
# do something
That way, if the letter in word
is uppercase, your loop will convert it to lowercase before checking it against your vowel list.
Now to the loop. The loop isn't going to iterate over each letter in word_list
as much as it will iterate over each index number in word_list
. This means that each time you remove a letter, every following letter's index number will change by 1, causing the letter right after a vowel to be skipped. If there are two vowels in a row, the second one will never get checked. There's a couple ways around this, but one is to create a new list and append non-vowels to it, rather than removing vowels from the old list. You can do this by checking to see if a letter is not in
your vowels list.
new_list = [] # store an empty list in a variable
for letter in word_list:
if letter.lower() not in ['a', 'e', 'i', 'o', 'u']:
new_list.append(letter)
Finally, you're currently returning the list itself, but you need to turn the list back into a string before returning it. You can use .join()
to accomplish this. Let me know how it goes!
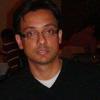
Mohammad Aslam
6,053 PointsThanks a lot! I was able to complete the challenge. What is the other way of getting around the skipping letter problem?
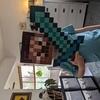
Gabbie Metheny
33,778 PointsOne other option is to make a copy of the list using a slice
, and iterating over the original list while mutating the slice. There are some details in this thread.
Another option is to iterate over the original string, and use the .replace()
method to replace vowels with an empty string (''), again some more details in another thread.
There's almost always more than one right way to code something!
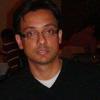
Mohammad Aslam
6,053 PointsThanks!
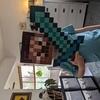
Gabbie Metheny
33,778 PointsOf course!