Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial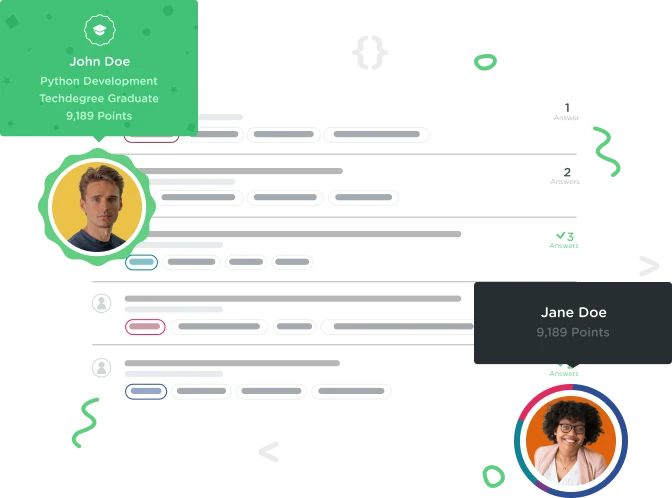

srikanth soma
1,572 PointsDisemvowel please make me understand where am I doing wrong?
def disemvowel(word):
vowels = ["a","A","e","E","i","I","o","O","u","U"]
words_list = list(word)
for letters in word_list:
if letters is in vowels:
try:
words_list.remove(letters)
except ValueError:
pass
return word
[MOD: added python to formatting -cf]
7 Answers
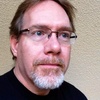
Chris Freeman
Treehouse Moderator 68,441 PointsYou are close. There are some typos and syntax that need fixing:
- Use a copy of
words_list
infor
loop to keep from modifying the iterable object. Add slice notation [:] - Correct typos
word_list
should bewords_list
- Remove "is" from
if
statement - Use
"".join()
to combine the letters ofwords_list
into a single string and return it.
Post back if you need more hints. Good luck!!

srikanth soma
1,572 PointsSorry, I don't understand that what does it do? and how can I add that?
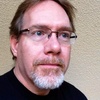
Chris Freeman
Treehouse Moderator 68,441 Points# by changing this line
for letters in words_list:
# to this line:
for letters in words_list[:]:
This creates a copy of words_list
to iterate over. So when a letter is removed from the actual words_list it does not mess up the for loop execution.

srikanth soma
1,572 PointsThanks for helping out I'll try that is that method called slicing?
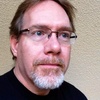
Chris Freeman
Treehouse Moderator 68,441 PointsCorrect. A slice
always returns a new copy of a list.
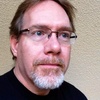
Chris Freeman
Treehouse Moderator 68,441 PointsJust chatted with Kenneth Love. The slice is not necessary if you avoid using the remove
approach. Think about using append
to accumulate the results. Something like:
results = []
for letter in word_list:
if letter in vowel_list:
results.append(letter)
return "".join(results)

srikanth soma
1,572 PointsI've not gone through slicing concept yet and instructor expects to use that so strange!

srikanth soma
1,572 Pointsdef disemvowel(word):
vowel_list = ["a","e","i","o","u","A","E","I","O","U"]
word_list = list(word)
for letter in word_list:
if letter in vowel_list:
word_list.remove(letter)
return word
Sorry I don't understand that your logic says if a letter in word is in vowel_list then append that to results what ? why are we adding vowels in to a new list ? can you integrate that with my above logic and can you add comments what do you mean by that ?
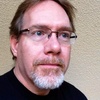
Chris Freeman
Treehouse Moderator 68,441 PointsMy other suggested approach is trying to solve without using a slice notation in the for
statement.
If using your specific solution, then I'd modify it by making an explicit copy of the word_list:
def disemvowel(word):
vowel_list = ["a","e","i","o","u","A","E","I","O","U"]
word_list = list(word)
copy_list = word_list.copy() # make copy of list
for letter in copy_list: # loop over copy so 'remove' doesn't mess up looping
if letter in vowel_list:
word_list.remove(letter)
return "".join(word_list) # fixed return value

srikanth soma
1,572 PointsThis worked but why do we need to create a copy of our list why can't we just iterate through our word_list?
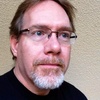
Chris Freeman
Treehouse Moderator 68,441 PointsBy using the remove
method it is altering the iterable used by the for loop. This removal changes the reference indexes used by the for loop to access items in the iterable. Look at example below.
vowels = "aeiou"
chars = list("abcdefghi")
print("first just print everything")
for char in chars:
print("comparing {}".format(char))
print("chars result: {}".format(chars))
chars = list("abcdefghi")
print("next use remove and print everything")
for char in chars:
print("comparing {}".format(char))
if char in vowels:
chars.remove(char)
print("chars result: {}".format(chars))
chars = list("abcdefghi")
print("Use copy with remove and print everything")
for char in chars.copy():
print("comparing {}".format(char))
if char in vowels:
chars.remove(char)
print("chars result: {}".format(chars))
# produces
first just print everything
comparing a
comparing b
comparing c
comparing d
comparing e
comparing f
comparing g
comparing h
comparing i
chars result: ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
next use remove and print everything
comparing a
comparing c
comparing d
comparing e
comparing g
comparing h
comparing i
chars result: ['b', 'c', 'd', 'f', 'g', 'h']
# notice skipped/missing iterations
Use copy with remove and print everything
comparing a
comparing b
comparing c
comparing d
comparing e
comparing f
comparing g
comparing h
comparing i
chars result: ['b', 'c', 'd', 'f', 'g', 'h']

srikanth soma
1,572 PointsI'm dumb I don't understand it thanks for helping out
srikanth soma
1,572 Pointssrikanth soma
1,572 PointsThanks but I still see the error saying I wasn't expecting letters
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsYou're nearly finished. Add the missing slice notation [:] to
words_list
in thefor
statement as mentioned in the first bullet point.