Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial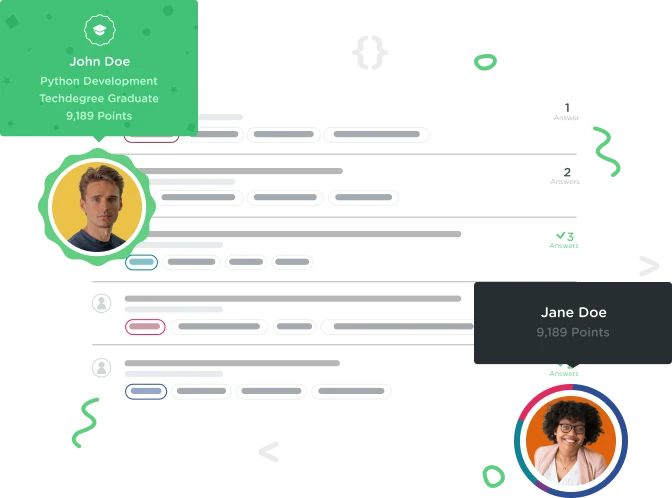

Boštjan Borič
Courses Plus Student 836 PointsDoes moving guesses.append(guess) to the end of the else clause really make a difference?
At 4:20 Kenneth is suggesting that guesses.append(guess)
should be moved to the end of the else clause in order to prevent breaking out of the loop before printing the messages, causing the user to not get any confirmation even if they get the answer on their 5th try. But this is a fundamental misunderstanding of the control flow of the while loop:
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
guesses.append(guess)
should be equivalent to:
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
guesses.append(guess)
# compare guess to secret number
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
The condition is only evaluated at the beginning of a new iteration of the while loop. Changing the length of the guesses list by appending to it and thereby possibly changing the boolean value of the "len(guesses) less than 5" expression should have absolutely no effect on the execution of the current iteration of the while loop - we won't break out of the while automagically at the moment when "len(guesses) == 5" if it happens somewhere in the middle of the current iteration.
1 Answer
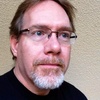
Chris Freeman
Treehouse Moderator 68,441 PointsYou are correct that the guess append statement in either location has the same outcome for this program.
If the program had intentions to do more with the previous guesses, then the earlier location would be preferred so that the last guess get appended before the while loop breaks.
Tagging Kenneth Love for further comment.
Boštjan Borič
Courses Plus Student 836 PointsBoštjan Borič
Courses Plus Student 836 PointsBtw, I can't seem to be able to spell out
len(guesses) < 5
in the middle of a paragraph, Markdown's single backticks aren't supported?