Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial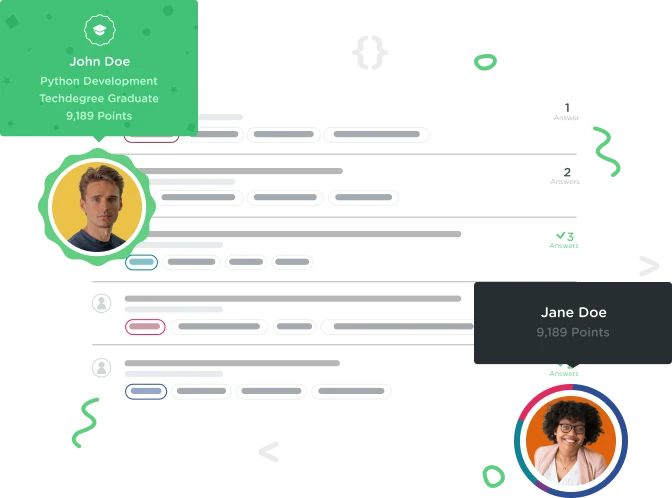

Herman Vicens
12,540 PointsDon't know/understand the second parameter of the FOR statement.
I am confused with the format. During the course, I've seen several ways of stating this parameter but not really understand what Im doing. Can someone explain the
for (List myVar : ListName) . When the LIST is an OBJECT and I need a specific property of that object, how should I write the ListName in this FOR loop? For example, getting the mAuthor value.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Set;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Set<String> getAllAuthors() {
Set<String> allAuthors = new HashSet<String>();
for (List authors : mPosts.getAuthor()) {
allAuthors.addAll(authors);
}
Set<String> allAuthorsSorted = new TreeSet<String>(allAuthors);
return allAuthorsSorted;
}
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
}
5 Answers
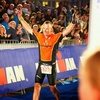
Steve Hunter
57,712 PointsRight, in your for
loop, you've created a local variable called post
which is of type BlogPost
. Correct.
However, you're trying to iterate over getAuthor()
- that's not right. You want to iterate over the collection of BlogPost
s which is called mPosts
.
Inside the loop, call a method that's part of the Set
or TreeSet
method which is .add()
. This does what it says;it adds to the set. This isn't a part of the current classes that we're dealing with - it is to do with the functionality of the particular datatype, such as String, Set, whatever.
Inside the brackets, pass in the local variable post
which is a BlogPost
instance. All instances of BlogPost
have a getAuthor
method which returns a String
representation of the author of the post.
So, something like:
for(BlogPost post : mPosts){
posts.add(post.getAuthor());
}
I wrote a long post in here about the objects, constructors etc - I'll dig that out as I think that'll help too.
Have a read of the above, and the link I'll send shortly and then get back to me with what's not clear, or what is clear. We can take it from there.
Steve.
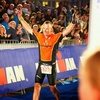
Steve Hunter
57,712 PointsHi Herman,
Take your example code:
for (List myVar : ListName){
// do something with myVar;
}
The variable, myVar
is local to the loop and is of type List. This is unlikely unless ListName
is a List of Lists. Let's say you have a list of names which is held in ListName
- the names are of type String
. The appropriate for
loop would look like:
for(String name : ListName){
// do something with a name
}
The variable, name
, is local to the loop and is of type String
. The loop iterates over ListName
, picking out each item in the list in turn. Each item is temporarily held in name
whilst the body of the loop is executed. Once that repeat of the loop concludes, the next loop starts, with the next item in the list then being stored in name
. This continues until there are no more entries in the list and the loop concludes.
I hope that explains the first part of your question. The second part, I am not clear on - which part of the challenge is requiring a for
loop, or what is it your are trying to solve? Let me know and I'll try to help.
Steve.
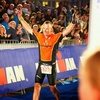
Steve Hunter
57,712 PointsJust clicked through to the challenge - I'll have a go at it and see if I can walk you through the solution. Apologies for not doing so before!
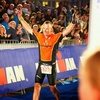
Steve Hunter
57,712 PointsHi again,
So, you're wanting to iterate over mPosts
which is a list of multiple BlogPost
s.
So, for your for
loop, you want to have a local variable, as described in my first answer, which is of type BlogPost
. Call it post
for the sake of clarity.
Then, inside your for
loop, you can access the name of the author by calling the getAuthor()
method on the post
variable.
You can .add()
that to a new Set
that you declare at the start of the method. Let's call that authors
. Inside the loop, take authors
and chain .add()
to it. Inside the brackets pass it post.getAuthor()
which will then add the author name into your set.
Return authors
after the for loop. You want it sorted alphabetically, so when you declare authors
make it a TreeSet
; but return a Set
of String
in your method signature. Don't forget to add to your imports.
That's it! Let me know how you get on.
Steve.

Herman Vicens
12,540 PointsThanks Steve! I fully understand the mechanics of a FOR loop, but I am strugling with the HOW to access the variables when they are in a BlogPost or some similar structure. I was a programmer 30 years ago (COBOL, FORTRAN, BASIC, Etc.). Yes, you can think about it but don't say it please!! Anyway, the concepts of OBJECTS, where you transfer/manipulate whole data structures in a variable is whats making my life miserable right now. Getting a particular variable like mAuthor from a method such as getAuthor that is in a different Class BlogPost is not a simple concept to understand. It's either BlogPost.author sometimes, and other times is just mPosts and other times is getAuthor() and I have seen also the (BlogPost obj).getAuthor() which blew my mind. This is the problem right now:
public class Blog {
List<BlogPost> mPosts;
public Set<String> getAllAuthors() {
Set<String> posts = new TreeSet<String>();
for (BlogPost post : getAuthor()) { // I can get this for loop to use the getAuthor method.
posts.addall(post);
}
return posts;
I've tried everything I could possibly can with the second part of the fot loop. I can't get to the getAuthor() methos to access the mPosts data. I know exactly how the for-loop works but don't know how to work with objects enough to understand the mecanics.
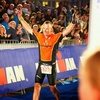
Steve Hunter
57,712 PointsMy background is also Fortran, Cobol, Pascal, Smalltalk, C - the good old stuff! I'm old too - don't worry.
I'll read the rest of your post and get back to you ASAP.

Herman Vicens
12,540 PointsThanks Steve!! Awsome work and awsome info. I am learning bit by bit and I don't expect everithing to click inmediately. I think I am ready to move forward in the course. I understood, finally, the relationship of the data structures in the For loop and where they come from. It is kind of a leap of feith when you call a Method (subroutine in my days) where there is only one statement and see all the things that happen with that one statement. fascinating!.. Your document fas the best explanation of the constructor so far. That I can say I understand.
Thanks again!
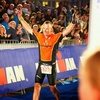
Steve Hunter
57,712 PointsOoo - subroutines. That's been a while.
It's all hard to compare. Best try to embrace the OOP principles!
If you want a further explanation of any concepts, let know - I will try to help!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHere's the link to the post I mentioned.
Have a read and get back to me, like I suggested. And give it an upvote if it helps!
Steve.