Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial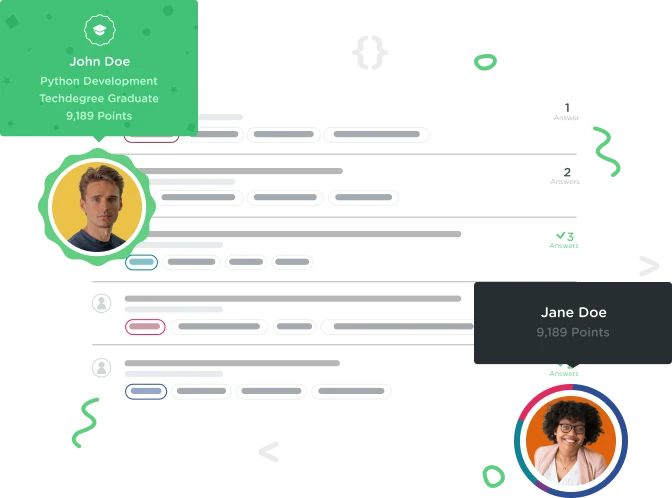
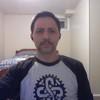
Jake Williams
2,900 Pointsdoubler.py challenge task 2
Not sure what I'm doing wrong.
class Double(int):
def __new__(self):
self = int.__new__:
return int(self)
2 Answers
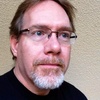
Chris Freeman
Treehouse Moderator 68,441 PointsHey Jake Williams, your code is not far off.
Breaking it down, the class definition is correct.
class Double(int):
The first method line needs two corrections:
def __new__(self):
- the first parameter of a method automatically receives the class reference. In a class instance, this is a reference to the instance itself. The actual parameter name can be almost anything, but to help improve code readability, it is customary to use
self
. For a class method, such as those decorated with@classmethod
or the dunder method__new__
, the first parameter represents the defined class. Again, this parameter can be named almost anything, but differentiate from a class instance method, it is customary to usecls
for class methods and__new__
. - Since, you don't know what other arguments and keyword arguments are going to be passed in, it is customary to include a catchall tuple for the positional arguments and a catchall dict for the keyword arguments. Again, these can be named almost anything, but it is customary to use
*args
and**kwargs
- this makes the corrected line look like
def __new__(cls, *args, **kwargs):
The second method line creates the new instance of the class, This needs to be broken down further:
self = int.__new__:
- Using
self
as the new instance name is perfect! Since usingself
is a customary reference for class instances - The proper way to call the
__new__
method is to reference the inherent class, or super class. This is done usingsuper()
instead of explicitly naming the class. This has the desired benefit of only have to change the inherited class name in one place (the class definition line). - the arguments to
__new__
should be the current class, which is captured bycls
in the first method line. followed the any arguments also captured in the catch-all parameters*args
and**wkargs
. - putting this all together yields:
self = super().__new__(cls, *args, **kwargs)
Finally, the third method line returns the result.
return int(self)
- Since the instance has be properly created in the second line , it can be simply returned:
return self
While there are many solutions that happen to pass the challenge, they may incorrect in various way. Common errors include:
- Explicitly name the inherited class, instead of using
super()
- forgetting to include a class placeholder
cls
. This accidentally works because the class reference gets absorbed into the*args
. This is bad form.
A well written __new__
method would allow simply changing the inherited class to get a new result:
# defining Double as subclass of int
# class Double(int):
>> Double("123")
246
# redefining Double as subclass of str
# class Double(str):
>>> Double("abcde")
'abcdeabcde'
Post back if you have more questions. Good luck!!
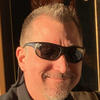
Peter Vann
36,427 PointsHi Jake!
Obviously, this passes task 1:
class Double(int):
pass
And you must have gotten that far.
I had to research this myself and found this:
https://teamtreehouse.com/community/create-a-new-int-instance-from-new
The answer by Qasa Lee worked for me.
This passes task 2:
class Double(int):
# Override __new__ passing in arguments and keywords
def __new__(*args, **kwargs):
# Creat new int instance and return it
return int.__new__(*args, **kwargs)
Task 3 should be pretty straightforward.
(It's just as simple as it sounds.)
Just edit the last line to multiply the return value by 2 (hence doubling the int as the class name suggests).
Also, for best results, make sure all your indentation is in multiples of 4 spaces precisely (4/8/12/16/20/etc. and don't use tabs).
I hope that helps.
Stay safe and happy coding!
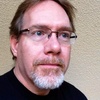
Chris Freeman
Treehouse Moderator 68,441 PointsThis is incorrect. This solution does not utilize super()
. This solution omits the class parameter.
The research answer at link does not provide the correct solution. I've marked up that post accordingly.