Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial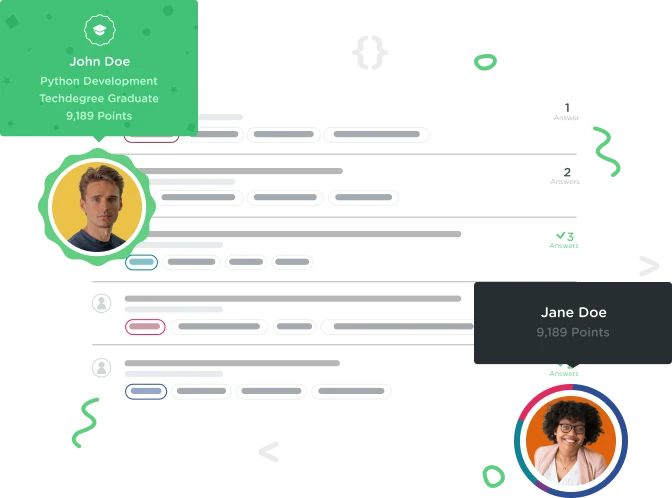
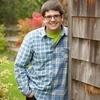
Sam Dale
6,136 PointsDungeon Game Feedback
Hi there. I just finished my version of the dungeon game and would love some feedback. I'm fresh on the Python track, so my code isn't incredibly concise/neat. I'd especially love some feedback on what I can do to write better code in upcoming courses. </br>Thanks Kenneth Love for the courses. Python Basics and Collections are definitely the clearest and most educational Python lessons on the Internet I've been able to find.
Things that I added:
- clear(), ability to change dungeon size, changed the board look, add "." wherever the player has been, if player wins show grue, if the player loses show the door, changed menu formatting/message display
import random
import os
cells = [] # list of tuples that represent the board
player = {"old": []} # player will contain "current" and list of old locations
board = [] # for drawing the board
def make_board(size):
new_board = []
for i in range(0, size * 4):
if i % 4 == 0:
new_board.append(" ___ " * size)
elif i % 4 == 3:
new_board.append("|___|" * size)
else:
new_board.append("| |" * size)
return new_board
def get_locations():
monster = random.choice(cells)
door = random.choice(cells)
player = random.choice(cells)
# if monster, door, or start are the same, do it again
if monster == door or monster == player or player == door:
return get_locations()
return monster, door, player
def move_player(player, move):
x, y = player["current"] # can't modify player itself since it's a tuple
player["old"].append((x, y))
if move == "LEFT":
y -= 1
elif move == "RIGHT":
y += 1
elif move == "UP":
x -= 1
elif move == "DOWN":
x += 1
return x, y
def get_moves(player, size):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
# player = (x, y)
if player[0] == 0:
moves.remove("UP")
elif player[0] == size - 1:
moves.remove("DOWN")
if player[1] == 0:
moves.remove("LEFT")
elif player[1] == size - 1:
moves.remove("RIGHT")
return moves
def draw(player, size, show_unknown = False, unknown = None, unknown_letter = None):
clear()
board = make_board(size)
tups = player["current"]
line = list(board[4 * player["current"][0] + 2])
if player["current"][1] == 0:
line[4 * player["current"][1] + 2] = "X"
else:
line[5 * player["current"][1] + 2] = "X"
board[4 * player["current"][0] + 2] = "".join(line)
for item in player["old"]:
if not item == player["current"]:
line = list(board[4 * item[0] + 2])
if item[1] == 0:
line[4 * item[1] + 2] = "."
else:
line[5 * item[1] + 2] = "."
board[4 * item[0] + 2] = "".join(line)
if show_unknown and unknown != None:
line = list(board[4 * unknown[0] + 2])
if unknown[1] == 0:
line[4 * unknown[1] + 2] = unknown_letter
else:
line[5 * unknown[1] + 2] = unknown_letter
board[4 * unknown[0] + 2] = "".join(line)
for line in board:
print(line)
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
# Welcome screen:
clear()
print("\nWelcome to the dungeon!\n")
# Create dungeon:
size = 4
try:
size = int(input("Enter size of dungeon (def. 4): "))
except:
print("Default size 4.")
for i in range(0, size):
for j in range(0, size):
cells.append((i, j))
# Fill dungeon:
monster, door, player["current"] = get_locations()
# Game loop:
clear()
message = ""
while True:
moves = get_moves(player["current"], size)
draw(player, size)
if message != "":
print(message)
print("You're currently in room {}.".format(player["current"]))
print("You can move {}.".format(", ".join(moves)))
print("Enter QUIT to quit.")
move = input("> ")
move = move.upper()
if move == "QUIT":
break
# if it's a good move, change the player's position
if move in moves:
player["current"] = move_player(player, move)
message = ""
else:
message = "** Walls are hard, stop walking into them! **"
continue
if player["current"] == door:
draw(player, size, show_unknown = True, unknown = monster, unknown_letter = "G")
print("You escaped!")
break
elif player["current"] == monster:
draw(player, size, show_unknown = True, unknown = door, unknown_letter = "D")
print("You were eaten by the grue!")
break