Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial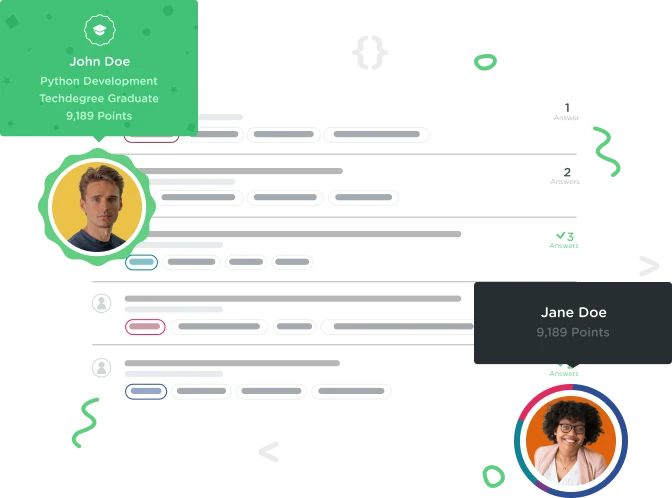
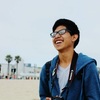
Victor Gordian
4,656 PointsEmpty strings?
What does an empty string do? or for what use?
var html = ' ';
2 Answers
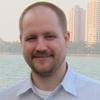
Ryan Field
Courses Plus Student 21,242 PointsHi, Victor. When you create an empty string like that, basically you are telling the program that you are creating a variable that is a string, and you will be using it to create a longer string later (through concatenation, etc.).
You don't need to declare it's type (you could just use var html;
), but this way you can tell with a glance what kind of variable it is when you look back on it later, which is especially useful if its declaration is quite a bit above its actual usage.
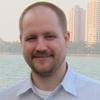
Ryan Field
Courses Plus Student 21,242 PointsHey, Jeramae. You're very close, but that's not quite the reason.
In your example, you're using var html =
which will overwrite whatever is stored in html each time the loop runs. This is why you only get 10 if you call document.write
outside of the for loop. If you used var html +=
instead, it would concatenate and you would have all 10 divs with document.write
called outside of the for loop.
As for why Dave uses html = ''
, that is somewhat unrelated to this. It's good practice to declare what type your variables are if they aren't being used right away. Using var html = ''
is stating that your variable is a string. Similarly, if you wanted to have an integer variable (but have no initial value), you could write var theCount = 0
. Like I mentioned above, though, you can just declare var theCount
and your code would function just fine in most cases. Declaring your variable type helps you avoid undefined values, too. Sometimes you want to know if a string is emtpy, but if you only declare var html
, an if statement like if (html === '')
will evaluate in false
because it is undefined.
As a side note, if you use variable inside things like for loops and if/else statements that will be used again later outside them, you should be declaring your variable outside, before them. If your variable is only being used inside a loop or if/else statement, declaring it inside is fine, though.
A good example:
var html = '';
for ( var i = 1; i <= 10; i += 1) {
html += '<div>' + i + '</div>';
}
document.write(html);

Jeramae Bohol
4,550 PointsThanks for the clarification Ryan.
Now it's crystal clear to me :)
Jeramae Bohol
4,550 PointsJeramae Bohol
4,550 PointsRyan, the code below results the same like the one on the video. But because it has the same results, I won't conclude that it's the same solution. But is my code below considered as also the same solution to the expected result?
I also discovered that when the document.write(html); is declared outside the loop, it will just display 10. I guess that's why Dave uses the var html = ''; to be able to concatenate it later to still display the 1 2 3 4 5 6 7 8 9 10 even when he declared the document.write(html); outside the loop.
Am I right? If not, please correct me!