Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial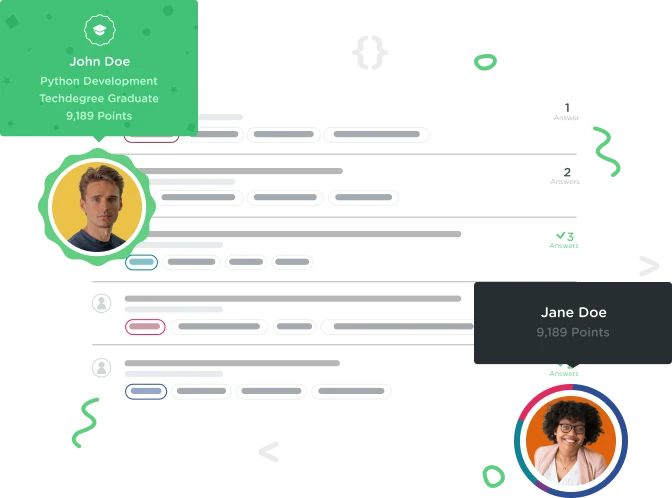

Matt Conway
1,572 PointsEnumerations & Optionals
Forgot to add instructions in last discussion. Wonder what is wrong with my code?
In the editor below you have two objects - classes named Point and Robot. The Robot stores its location as a point instance and contains a move function.
The task of this challenge is to complete the implementation for move. Move takes a parameter of type Direction which is an enumeration listing the possible movement directions.
When you tell the robot to move up (by specifying Direction.Up as the argument), the y coordinate should increase by 1. Similarly moving down means the y coordinate decreases by 1, moving right means the x coordinate increases by 1 and finally left means x decreases by 1.
/Users/mattconway/Desktop/Screen Shot 2016-03-01 at 5.04.57 PM.png
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
// Enter your code below
}
}
2 Answers

Helgi Helgason
7,599 PointsYou need to write a control statement that moves the Robot depending on the parameter. Since Direction is an enum and therefore exhaustive a switch statement would work well!
func move(direction: Direction) {
// Enter your code below
switch(direction) {
case Direction.Up: location.y += 1
break
case Direction.Down: location.y -= 1
break
case Direction.Left: location.x -= 1
break
case Direction.Right: location.x += 1
}

Reed Carson
8,306 Pointsheres a hint, youll want to use a switch statement in the move funciton. switch on "direction". since its an enum it will have only the 4 cases. for each case youll have to adjust the position in a different way
Jennifer Masserano
Courses Plus Student 2,571 PointsJennifer Masserano
Courses Plus Student 2,571 PointsDefinitely remove the breaks. Since this is an enum and exhaustive switch statement you don't need the breaks.