Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial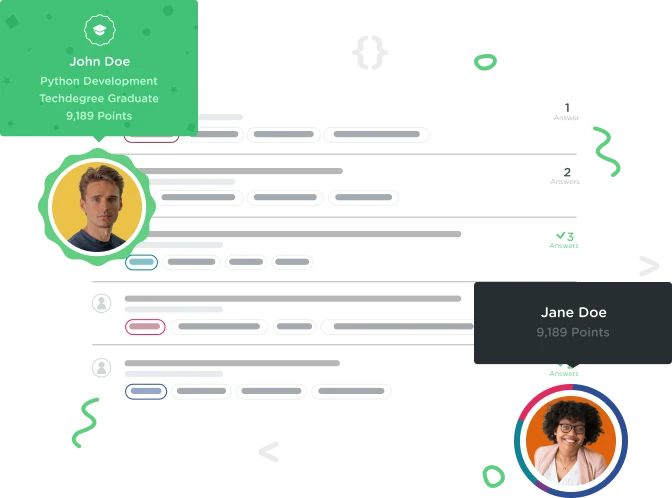

Iain Simmons
Treehouse Moderator 32,305 PointsException from model if username/email already exists isn't returned in JSON
Hi Kenneth Love, me again!
For the post
method on the User
resource, your existing code will return the built-in error page in HTML if the username or email already exists (we're raising a regular Exception
on the model).
Here's a solution that will return the error message in JSON format, along with the 400 HTTP status code.
Also note that again, you don't need make_response
or anything from Flask, since you can just return a dict and Flask-RESTful will properly return a JSON-encoded response from it.
def post(self):
args = self.reqparse.parse_args()
if args.get('password') == args.get('verify_password'):
try:
user = models.User.create_user(**args)
except Exception as error:
return {'error': str(error)}, 400
else:
return marshal(user, user_fields), 201
return {
'error': 'Password and password verification do not match'
}, 400
3 Answers
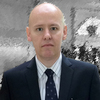
Nathan Tallack
22,164 PointsNicely done! :)
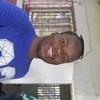
Innocent Ngwaru
7,554 PointsHi Iain. I m sorry I did not submit my question, and also asking on your question. For some reason I cannot use the 'Get Help' button. I dont know why? My question relates to Challenge 2 of 2 for Creater User in Flask Rest API, API Protection category. I have been stuck here for some time now. My code passed Challenge 1 of 2, and is as below:
@marshal_with(user_fields)
def post(self):
args = self.reqparse.parse_args()
if args.get('password') == args.get('confirm_password'):
try:
user = models.User.create_user(**args)
except ExceptionError:
return 'error'
else:
return marshal(user, user_fields), 201
return 'Password and password verification do not match'
users_api = Blueprint('resources.users', name) api = Api(users_api) api.add_resource(UserList, '/api/v1/users')

Ben Hedgepeth
Python Web Development Techdegree Student 10,288 PointsNice job pointing that out! I was think about that myself.