Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial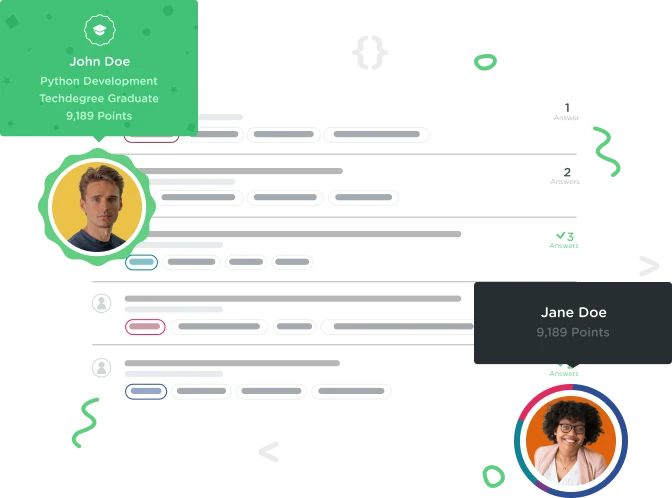

shekhar bhardwaj
Full Stack JavaScript Techdegree Student 12,373 PointsException in thread "main" org.hibernate.MappingException: Unknown entity: com.contact.model.Contact
My hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="connection.driver_class">org.h2.Driver</property>
<property name="connection.url">jdbc:h2:./data/contactmgr</property>
<property name="connection.username">sa</property>
<property name="hibernate.default_schema">PUBLIC</property>
<!-- JDBC connection pool (use the built-in) -->
<property name="connection.pool_size">1</property>
<!-- SQL dialect -->
<property name="dialect">org.hibernate.dialect.H2Dialect</property>
<!-- Disable the second-level cache -->
<property name="cache.provider_class">org.hibernate.cache.internal.NoCacheProvider</property>
<!-- Echo all executed SQL to stdout -->
<property name="show_sql">true</property>
<!-- Drop and re-create the database schema on startup -->
<property name="hbm2ddl.auto">create</property>
<property name="show_sql">true</property>
<mapping class="com.contact.model.Contact"/>
</session-factory>
</hibernate-configuration>
My Application.java class
package com.contact;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.nio.channels.FileLock;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.cfg.Configuration;
import org.hibernate.service.ServiceRegistry;
import com.contact.model.Contact;
public class Application {
//Session factory
private static final SessionFactory sessionFactory = buildSesssionFactory();
public static void main(String[] args) throws InterruptedException {
System.out.println("Session factory");
Contact contact = new Contact.ContactBuilder("Bob", "Marley").withEmail("bob.nik@gmail.com").withPhone(5859789733L).build();
//Open a Session
System.out.println("Open a Session");
Session session = sessionFactory.openSession();
//Begin a Transaction
System.out.println("Begin a Transaction");
session.beginTransaction();
//Use the session to save the contact
System.out.println("Use the session to save the contact");
session.save(contact);
//Commit the transaction
System.out.println("Commit the transaction");
session.getTransaction().commit();
// Close the session
System.out.println("Close the session");
session.close();
}
private static SessionFactory buildSesssionFactory() {
// Create a StandardServiceRegistry
Configuration configuration = new Configuration();
configuration.addAnnotatedClass(com.contact.model.Contact.class);
configuration.setProperty("hibernate.temp.use_jdbc_metadata_defaults","false");
configuration.configure();
final ServiceRegistry registry = new StandardServiceRegistryBuilder().applySettings(configuration.getProperties()).build();
return new org.hibernate.boot.MetadataSources(registry).buildMetadata().buildSessionFactory();
}
static boolean isLocked(String fileName) {
try {
RandomAccessFile f = new RandomAccessFile(fileName, "r");
try {
FileLock lock = f.getChannel().tryLock(0, Long.MAX_VALUE, true);
if (lock != null) {
lock.release();
return false;
}
} finally {
f.close();
}
} catch (IOException e) {
// ignore
}
return true;
}
}
my Contact.java pojo or Entity
package com.contact.model;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="CONTACT")
public class Contact {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
@Column
private String firstName;
@Column
private String lastName;
@Column
private String email;
@Column
private Long phone;
public Contact() {
}
public Contact(ContactBuilder contactBuilder) {
this.firstName = contactBuilder.firstName;
this.lastName = contactBuilder.lastName;
this.email = contactBuilder.email;
this.phone=contactBuilder.phone;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Long getPhone() {
return phone;
}
public void setPhone(Long phone) {
this.phone = phone;
}
@Override
public String toString() {
return "Contact [id=" + id + ", firstName=" + firstName + ", lastName=" + lastName + ", email=" + email
+ ", phone=" + phone + "]";
}
public static class ContactBuilder {
private String firstName;
private String lastName;
private String email;
private Long phone;
public ContactBuilder(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public ContactBuilder withEmail(String email) {
this.email = email;
return this;
}
public ContactBuilder withPhone(Long phone) {
this.phone = phone;
return this;
}
public Contact build() {
return new Contact(this);
}
}
}
Exception in thread "main" org.hibernate.MappingException: Unknown entity: com.contact.model.Contact at org.hibernate.internal.SessionFactoryImpl.getEntityPersister(SessionFactoryImpl.java:776) at org.hibernate.internal.SessionImpl.getEntityPersister(SessionImpl.java:1533) at org.hibernate.event.internal.AbstractSaveEventListener.saveWithGeneratedId(AbstractSaveEventListener.java:104) at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.saveWithGeneratedOrRequestedId(DefaultSaveOrUpdateEventListener.java:192) at org.hibernate.event.internal.DefaultSaveEventListener.saveWithGeneratedOrRequestedId(DefaultSaveEventListener.java:38) at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.entityIsTransient(DefaultSaveOrUpdateEventListener.java:177) at org.hibernate.event.internal.DefaultSaveEventListener.performSaveOrUpdate(DefaultSaveEventListener.java:32) at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.onSaveOrUpdate(DefaultSaveOrUpdateEventListener.java:73) at org.hibernate.internal.SessionImpl.fireSave(SessionImpl.java:682) at org.hibernate.internal.SessionImpl.save(SessionImpl.java:674) at org.hibernate.internal.SessionImpl.save(SessionImpl.java:669) at com.contact.Application.main(Application.java:38)
1 Answer

shekhar bhardwaj
Full Stack JavaScript Techdegree Student 12,373 Pointsconfiguration.addAnnotatedClass(com.contact.model.Contact.class);
resolves the issue, which is weird because it means
<mapping class="com.contact.model.Contact"/>
is not doing it for you. I don't like xml configuration anyways, good riddance.