Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial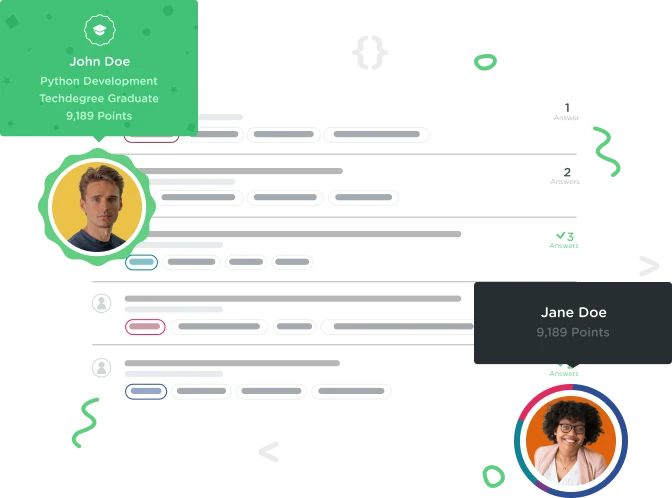
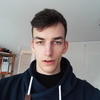
Immo Struchholz
10,515 PointsExtra credit
So, I created a deleteContact method and added it to the menu.
This is how it's supposed to work:
Asks user for name of contact they want to delete
creates result array
pushes results from findByName method into results array.
User specifies which contact to delete by providing a number (1 for the first, 2 for the second etc.)
index variable takes the answer and substracts 1 to get the index of the contact in the results array
delete variable with results[index]
loops through all contacts.
Removes contact from contacts if it is the same as the one the user specified.
I have an easier version of this working, but it doesn't work properly if there are multiple results matching the search query. It would always remove the first one in that case.
Example:
User searches for: Obama
2 contacts with Obama as last name were found (Barrack Obama, Michelle Obama)
Only Barrack Obama gets removed because it comes before Michelle Obama.
That's why I created this more complicated method.
This is how it's looking atm:
def deleteContact
print "Which contact do you want to delete? "
name = gets.chomp.downcase
results = []
results.push(findByName(name))
print "Which of these do you want to delete? Provide a number "
index = (gets.to_i) -1
delete = results[index]
puts delete.class #This returns NilClass for some reason, should return Fixnum
contacts.each do |contact|
if contact == delete
puts "Deleting #{contact.fullName}"
contacts.delete(contact)
end
end
printSeperator
end
It's not working. And I don't know why. I tested this in irb up until the loop and it was working fine. You can see that I put
puts delete.class
in there. I was just looking for the problem, it's not part of the program.
That is where the problem is I think. In irb this returned Fixnum, in the method it returns NilClass. I don't know why that is.
Here is the simpler method, that is working:
def deleteContact
print "Which contact do you want to remove?"
answer = gets.chomp.downcase
contacts.each do |contact|
if contact.fullName.downcase.include?(answer)
print "Delete #{contact.fullName}? (y/N)"
input = gets.chomp.downcase
case input
when 'y'
puts "Deleting #{contact.fullName}"
contacts.delete(contact)
end
end
end
printSeperator
end
It would be much appreciated if someone could help me out here.
1 Answer

Todd MacIntyre
12,248 PointsAll looks pretty good. It's hard to know for sure with out seeing the rest of the code, but if your method 'findByName' returns anything other than the contact in its entirety, you will run into problems.