Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
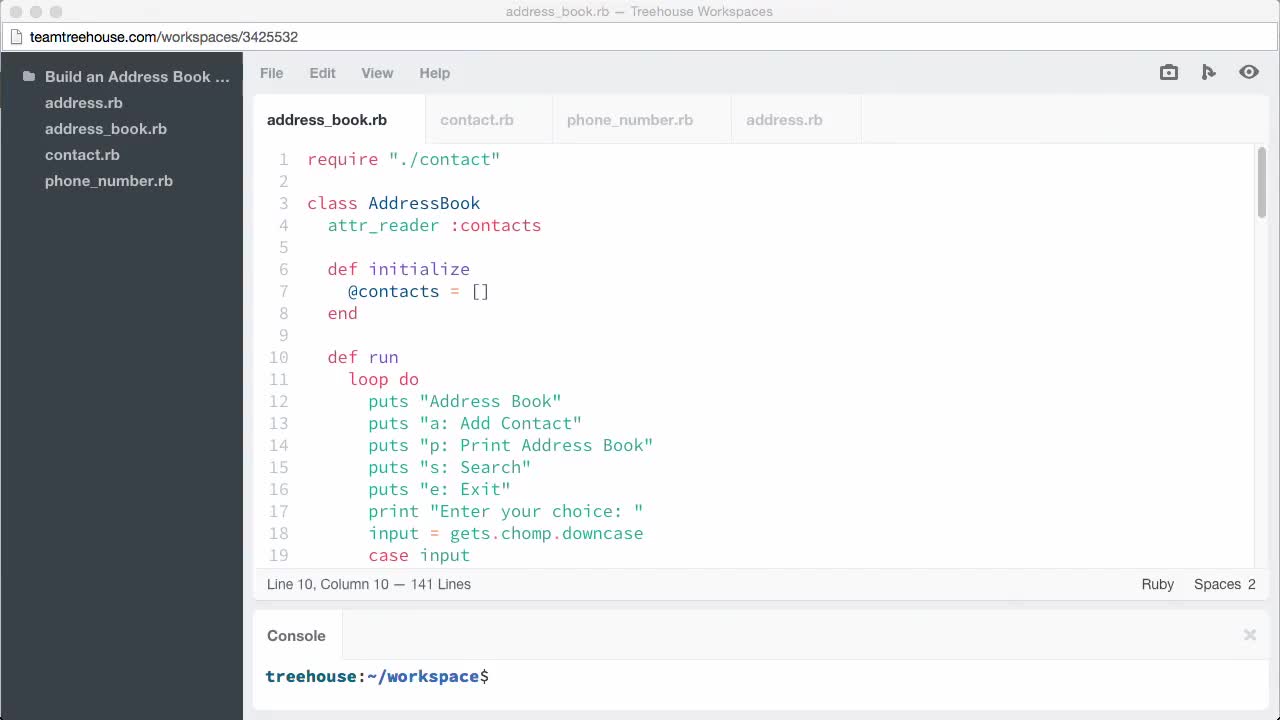
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Saving
Documentation Links
- Ruby IO Documentation
- Ruby YAML Documentation
- Ruby Psych Documentation - This is the class YAML interfaces with.
Code Samples
require "./contact"
require "yaml"
class AddressBook
attr_reader :contacts
def initialize
@contacts = []
open()
end
def open
if File.exist?("contacts.yml")
@contacts = YAML.load_file("contacts.yml")
end
end
def save
File.open("contacts.yml", "w") do |file|
file.write(contacts.to_yaml)
end
end
def run
loop do
puts "Address Book"
puts "a: Add Contact"
puts "p: Print Address Book"
puts "s: Search"
puts "e: Exit"
print "Enter your choice: "
input = gets.chomp.downcase
case input
when 'a'
add_contact
when 'p'
print_contact_list
when 's'
print "Search term: "
search = gets.chomp
find_by_name(search)
find_by_phone_number(search)
find_by_address(search)
when 'e'
save()
break
end
puts "\n"
end
end
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Andrew Phythian
19,747 Points2 Answers
-
K .
2,357 Points1 Answer
-
Melissa Ramirez
6,503 Points0 Answers
-
Erik Nuber
20,629 Points6 Answers
-
Immo Struchholz
10,515 Points1 Answer
-
Jasmine Frantz
8,299 Points1 Answer
-
Sean Flanagan
33,236 Points2 Answers
-
Andrew Goninon
14,925 Points3 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up