Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial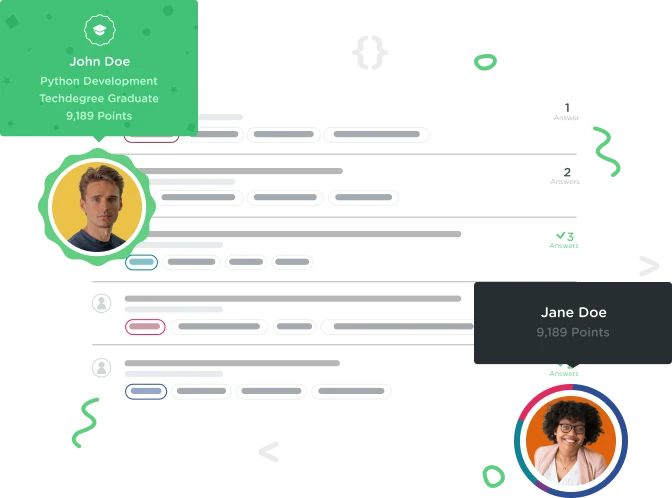

Andrew Phythian
19,747 PointsExtra credit solution - slightly different approach
I took a slightly different approach to the solution for extra credit.
I added the option to the menu (abbreviated code below)
puts "r: Remove a contact"
case input
when 'a'
add_contact
when 'r'
remove_contact
Then, in the print_contact_list method I added a number to each contact
def print_contact_list
puts "Contact List"
i = 1
contacts.each do |contact|
print "#{i}. "
print "#{contact.to_s('first_last')}"
i = i + 1
end
end
So when the contact list is printed it now produces the following output
1. Contact 1
2. Contact 2
3. Contact 3
This means that when I ask the user for a contact, they can give a number so they can target a specific contact rather than a name which could result in multiple returns if more than one contact shares an identical name.
In my remove_contact method, I added a check in there to make sure they'd picked the person they wanted as well as a way of backing out if they changed their mind...
def remove_contact
# Check that there are contacts to delete
if contacts.length > 0
# Present contact list to choose from
print_contact_list
# Loop to verify that a valid contact is chosen
loop do
print "Select a contact (number): "
id = gets.chomp
# Checks for a valid contact number
if id.to_i <= contacts.length
puts "You have selected contact #{id} - #{contacts[id.to_i - 1].first_name}"
print "Please confirm you wish to delete this contact (y/n): "
confirm = gets.chomp
case confirm
when 'y'
puts "Removing contact"
contact = id.to_i - 1
contacts.delete_at(contact)
puts "Here is the updated contacts list"
# Display updated contact list
print_contact_list
break
when 'n'
# Allows user to change their mind
puts "No problem, the contact will not be deleted."
break
end
else
puts "Please try again!"
end
end
else
puts "There are no contacts to remove."
end
end
It also gives the user an updated contact list after they have deleted so they get immediate feedback on their action.
2 Answers

Dillon Wyatt
5,990 Pointsdef print_contact_list
puts "Contact List"
i = 1
contacts.each do |contact|
print "#{i}. "
print "#{contact.to_s('first_last')}"
i = i + 1
end
end
Might I add a suggestion to help clean up this bit?
Ruby offers a method "each_with_index". You can use it on an array, pull out both the content of the slot and its position in one shot. This way you don't need to create an counter that must be incremented.
Thus
contacts.each_with_index do |contact, index|
print "#{index + 1}" # Indexes start at 0
print "#{contact}"
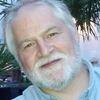
Jeff Muday
Treehouse Moderator 28,722 PointsNice work! Easy to read and does way more than the basic requirement. You're practicing a good paradigm making deletion of a contact require an explicit confirmation.
Andrew Phythian
19,747 PointsAndrew Phythian
19,747 PointsThanks for the suggestion, works perfectly.