Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial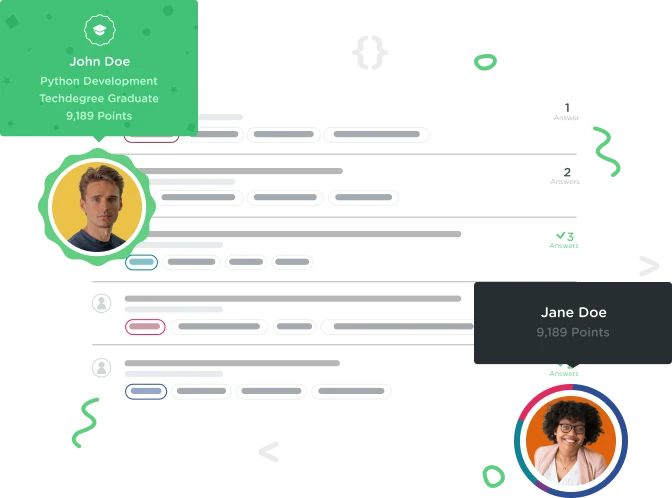

Camille Ferré
3,330 PointsFeedback on game
Hi all,
Following the videos on object oriented Python and the monster game, I created another game using classes. It is probably a bit messy but it would be great to have a few general feedback if possible. The game works properly. It is composed by two players that each have three animals that they need to take care of. I feel like there is a lot of repetition but I didn't know how to automatically automatize iterations. Let me know if any questions and if anyone has feedback on how to improve the game, it is more than welcome. thanks a lot
Animal classes
class Animal():
max_food = 10
max_soap = 10
max_activities = 10
def __init__(self):
self.clean = int(self.current_soap / self.max_soap *100)
self.fed = int(self.current_food / self.max_food *100)
self.happy = int(self.current_activities / self.max_activities *100)
if self.__class__.__name__ in ['Cow1', 'Cow2']:
self.name = 'cow'
elif self.__class__.__name__ in ['Hen1', 'Hen2']:
self.name = 'hen'
else:
self.name = 'sheep'
def __str__(self):
self.clean = int(self.current_soap / self.max_soap *100)
self.fed = int(self.current_food / self.max_food *100)
self.happy = int(self.current_activities / self.max_activities *100)
return " Your {} is {}% clean, {}% fed and {}% happy ".format(self.name,
self.clean,
self.fed,
self.happy)
class Cow(Animal):
max_food = 15
max_soap = 9
max_activities = 7
current_food = 5
current_soap = 3
current_activities = 3
class Hen(Animal):
max_food = 4
max_soap = 4
max_activities = 10
current_food = 2
current_soap = 2
current_activities = 3
class Sheep(Animal):
max_food = 6
max_soap = 12
max_activities = 8
current_food = 2
current_soap = 4
current_activities = 3
class Cow1(Cow):
pass
class Cow2(Cow):
pass
class Hen1(Hen):
pass
class Hen2(Hen):
pass
class Sheep1(Sheep):
pass
class Sheep2(Sheep):
pass
Player Classes:
class Player:
current_food = 5
current_soap = 3
current_activities = 3
def __init__ (self):
self.Name = input("Name: ").lower().title()
def __str__ (self):
return '{}, you have {} item(s) of food, {} soap(s) and {} activity(ies) left'.format(self.Name,
self.current_food,
self.current_soap,
self.current_activities)
class Player1 (Player):
pass
class Player2 (Player):
pass
Game
from animal import Cow1, Cow2
from animal import Sheep1, Sheep2
from animal import Hen1, Hen2
from player import Player1
from player import Player2
import random
import sys
class Game:
def rules(self):
print('Welcome to the farming game,')
print("You have three animals that have needs (food, cleanliness and happiness)")
print("They are at the moment in a bad shape, you need to take care of them!")
print("You have items to take care of them: food, soaps and activities")
print("At the end of every turn, your animals' needs will increase but your items will increase also")
print("The universe has some stuff planned for you too :)")
print("Make sure you take care of each of your animal!")
print("")
print("Type END at any time to stop or HELP to know about the rules again")
print("")
def setup(self):
self.player1 = Player1()
self.player2 = Player2()
self.animal1 = [Cow1(), Sheep1(), Hen1()]
self.animal2 = [Cow2(), Sheep2(), Hen2()]
def printi(self, player):
if player == self.player1:
animal = self.animal1
if player == self.player2:
animal = self.animal2
#check that the needs can not be higher than max
for ani in animal:
if ani.current_food > ani.max_food:
ani.current_food = ani.max_food
if ani.current_soap > ani.max_soap:
ani.current_soap = ani.max_soap
if ani.current_activities > ani.max_activities:
ani.current_activities = ani.max_activities
# check that the needs can not be negative
for ani in animal:
if ani.current_food < 0:
ani.current_food = 0
if ani.current_soap < 0:
ani.current_soap = 0
if ani.current_activities < 0:
ani.current_activities = 0
# check that the resources can not be negative
for play in [self.player1, self.player2]:
if play.current_food < 0:
play.current_food = 0
if play.current_soap < 0:
play.current_soap = 0
if play.current_activities < 0:
play.current_activities = 0
print(player)
for ani in animal:
print(ani)
def turn(self, player):
if player == self.player1:
animal = self.animal1
if player == self.player2:
animal = self.animal2
print("")
print("=" * 20)
self.detect_end(input("It's {}'s turn (Press enter)".format(player.Name))).lower
print("=" * 20)
print("")
self.printi(player)
print("-" * 20)
print("")
print("You can do one action on each animal:")
self.actions(player)
print("")
self.printi(player)
print("-"*20)
self.detect_end(input("Now ! Let's see what the universe has prepared for you today ! Are you ready? (Press enter)")).lower()
print("-"*20)
score = random.randint(1,2)
self.universe(score, player)
print("")
if self.death() == 0:
self.printi(player)
def actions(self, player):
if player == self.player1:
animal = self.animal1
if player == self.player2:
animal = self.animal2
for ani in animal:
print("")
action = 3
while action != 'c' and action != 'f' and action != 'p':
action = str(self.detect_end(input("Would you like to [F]eed, [C]lean or [P]lay: {} > ".format(ani)).lower()))
action = str(action)
if action == 'f':
qty = -1
while (qty < 0 or qty > player.current_food):
print("You have {} points to use for this action".format(int(player.current_food)))
qty = int(float(self.check_number(self.detect_end(input("How many points do you want to use? > ")).lower())))
player.current_food -= qty
ani.current_food += qty
if action == 'c':
qty = -1
while qty < 0 or qty > player.current_soap and self.check_number(qty):
print("You have {} points to use for this action".format(int(player.current_soap)))
qty = int(float(self.check_number(self.detect_end(input("How many points do you want to use? > ")).lower())))
player.current_soap -= qty
ani.current_soap += qty
if action == 'p':
qty = -1
while qty < 0 or qty > player.current_activities and self.check_number(qty):
print("You have {} points to use for this action".format(int(player.current_activities)))
qty = int(float(self.check_number(self.detect_end(input("How many points do you want to use? > ")).lower())))
player.current_activities -= qty
ani.current_activities += qty
self.cleanup(player)
def __init__(self):
self.rules()
print("It's time to enter the names of the two players")
self.setup()
while self.death() == 0 and self.win() == 0:
self.turn(self.player1)
self.turn(self.player2)
if self.death() == 0 and self.win() == 0:
self.end_points()
looser = self.death()
winner = self.win()
self.end(looser, winner)
def remove_points (self, list_obj, points, list_animal):
if 'f' in list_obj:
for animal in list_animal:
animal.current_food -= points
if 's' in list_obj:
for animal in list_animal:
animal.current_soap -= points
if 'a' in list_obj:
for animal in list_animal:
animal.current_activities -= points
def add_points (self, list_obj, points, list_animal):
if 'f' in list_obj:
for animal in list_animal:
animal.current_food += points
if 's' in list_obj:
for animal in list_animal:
animal.current_soap += points
if 'a' in list_obj:
for animal in list_animal:
animal.current_activities += points
def universe(self, score, player):
if player == self.player1:
animal = self.animal1
if player == self.player2:
animal = self.animal2
print("")
if score == 1:
print("You got hit by the rain, some of your food went bad but your animals danced in the rain, they are cleaner and happier")
self.remove_points('f', 3, [player])
self.add_points('sa', 3, animal)
elif score == 2:
print("There was a lot of sun today, your animal were too hot to go outside, they are sad ! But you cropped a lot and earned food !")
self.add_points('f', 3, [player])
self.remove_points('a', 5, animal)
print("")
self.detect_end(input("--> Press enter ").lower())
print("")
self.cleanup(player)
def cleanup(self, player):
if player == self.player1:
animal = self.animal1
if player == self.player2:
animal = self.animal2
print("")
for ani in animal:
if ani.current_food <= 0:
print("! ! " * 20)
print("Your {}'s level of food is below 0... Your {} is dead.".format(ani.name, ani.name))
animal.remove(ani)
print(" You only have {} animal(s) left (Press enter)".format(len(animal)))
print("! ! " * 20)
self.detect_end(input("")).lower
for ani in animal:
if ani.current_soap <= 0:
print("! ! " * 20)
print("Your {}'s level of cleanliness is below 0... Your {} is dead.".format(ani.name, ani.name))
animal.remove(ani)
print(" You only have {} animal(s) left (Press enter)".format(len(animal)))
print("! ! " * 20)
self.detect_end(input("")).lower
for ani in animal:
if ani.current_activities <= 0:
print("! ! " * 20)
print("Your {}'s level of happiness is below 0... Your {} is dead.".format(ani.name, ani.name))
animal.remove(ani)
print(" You only have {} animal(s) left (Press enter)".format(len(animal)))
print("! ! " * 20)
self.detect_end(input("")).lower
def death(self):
if len(self.animal1)*len(self.animal2) == 0 and len(self.animal1) == len(self.animal2):
looser = 'both'
return looser
elif len(self.animal1) == 0:
looser = self.player1.Name
return looser
elif len(self.animal2) == 0:
looser = self.player2.Name
return looser
else:
return 0
def win(self):
turn = turn1 = 0
winner = 0
for ani in self.animal1:
if ani.current_food >= ani.max_food and ani.current_soap >= ani.max_soap and ani.current_activities >= ani.max_activities:
turn += 1
for ani in self.animal2:
if ani.current_food >= ani.max_food and ani.current_soap >= ani.max_soap and ani.current_activities >= ani.max_activities:
turn1 += 1
if turn == turn1 == 3:
return 'both'
elif turn == 3:
return self.player1
elif turn1 == 3:
return self.player2
else:
return 0
def end(self, looser, winner):
if winner == self.player1:
print("Congratulation {} ! All of your animals are doing great ! You win !".format(winner.Name))
elif winner == self.player2:
print("Congratulation {} ! All of your animals are doing great ! You win !".format(winner.Name))
elif winner == 'both':
print("Congratulation ! All of your animals are doing great ! You both win !")
elif looser == self.player1:
print("Congratulation {} ! You win ! {} has lost all the animals".format(self.player2.Name, looser))
elif looser == self.player2:
print("Congratulation {} ! You win ! {} has lost all the animals".format(self.player1.Name, looser))
elif looser == 'both':
print("Both player have lost all the animals, it's a tie!")
print("Thank you for playing!")
def detect_end(self, check):
if check == 'help':
self.rules()
if check == 'end':
sys.exit()
return check
def check_number(self, entry):
try:
int(float(entry))
except ValueError:
entry = self.detect_end(input("This is not a number, try again > "))
return int(float(self.check_number(entry)))
else:
return int(float(entry))
def end_points(self):
for listani in [self.animal1, self.animal2]:
for ani in listani:
ani.current_food = ani.current_food * 0.9
ani.current_soap = ani.current_soap * 0.9
ani.current_activities = ani.current_activities * 0.9
for player in [self.player1, self.player2]:
player.current_food += 5
player.current_soap += 5
player.current_activities += 5
Game()